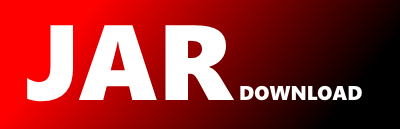
org.smallmind.persistence.cache.AbstractCacheDao Maven / Gradle / Ivy
/*
* Copyright (c) 2007, 2008, 2009, 2010, 2011, 2012, 2013, 2014, 2015, 2016 David Berkman
*
* This file is part of the SmallMind Code Project.
*
* The SmallMind Code Project is free software, you can redistribute
* it and/or modify it under either, at your discretion...
*
* 1) The terms of GNU Affero General Public License as published by the
* Free Software Foundation, either version 3 of the License, or (at
* your option) any later version.
*
* ...or...
*
* 2) The terms of the Apache License, Version 2.0.
*
* The SmallMind Code Project is distributed in the hope that it will
* be useful, but WITHOUT ANY WARRANTY; without even the implied warranty
* of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* General Public License or Apache License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* and the Apache License along with the SmallMind Code Project. If not, see
* or .
*
* Additional permission under the GNU Affero GPL version 3 section 7
* ------------------------------------------------------------------
* If you modify this Program, or any covered work, by linking or
* combining it with other code, such other code is not for that reason
* alone subject to any of the requirements of the GNU Affero GPL
* version 3.
*/
package org.smallmind.persistence.cache;
import java.io.Serializable;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.smallmind.persistence.Durable;
public abstract class AbstractCacheDao, D extends Durable> implements CacheDao {
private CacheDomain cacheDomain;
public AbstractCacheDao (CacheDomain cacheDomain) {
this.cacheDomain = cacheDomain;
}
public String getMetricSource () {
return cacheDomain.getMetricSource();
}
public PersistenceCache getInstanceCache (Class durableClass) {
return cacheDomain.getInstanceCache(durableClass);
}
public PersistenceCache getVectorCache (Class durableClass) {
return cacheDomain.getVectorCache(durableClass);
}
public D get (Class durableClass, I id) {
DurableKey durableKey = new DurableKey(durableClass, id);
return getInstanceCache(durableClass).get(durableKey.getKey());
}
public Map, D> get (Class durableClass, List> durableKeys) {
Map, D> resultMap = new HashMap, D>();
if ((durableKeys != null) && (!durableKeys.isEmpty())) {
HashMap> durableKeyMap = new HashMap>();
Map valueMap;
String[] keys = new String[durableKeys.size()];
int index = 0;
for (DurableKey durableKey : durableKeys) {
keys[index] = durableKey.getKey();
durableKeyMap.put(keys[index++], durableKey);
}
if ((valueMap = getInstanceCache(durableClass).get(keys)) != null) {
for (Map.Entry resultEntry : valueMap.entrySet()) {
resultMap.put(durableKeyMap.get(resultEntry.getKey()), resultEntry.getValue());
}
}
}
return resultMap;
}
public void delete (Class durableClass, D durable) {
if (durable != null) {
DurableKey durableKey = new DurableKey(durableClass, durable.getId());
getInstanceCache(durableClass).remove(durableKey.getKey());
}
}
public DurableVector getVector (VectorKey vectorKey) {
return getVectorCache(vectorKey.getElementClass()).get(vectorKey.getKey());
}
public DurableVector persistVector (VectorKey vectorKey, DurableVector vector) {
DurableVector migratedVector;
DurableVector cachedVector;
migratedVector = migrateVector(vectorKey.getElementClass(), vector);
return ((cachedVector = getVectorCache(vectorKey.getElementClass()).putIfAbsent(vectorKey.getKey(), migratedVector, migratedVector.getTimeToLiveSeconds())) != null) ? cachedVector : vector;
}
public void deleteVector (VectorKey vectorKey) {
getVectorCache(vectorKey.getElementClass()).remove(vectorKey.getKey());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy