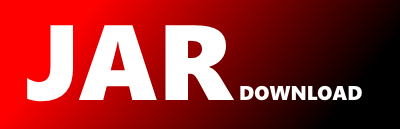
org.smallmind.persistence.cache.praxis.ByKeySingularVector Maven / Gradle / Ivy
/*
* Copyright (c) 2007, 2008, 2009, 2010, 2011, 2012, 2013, 2014, 2015, 2016 David Berkman
*
* This file is part of the SmallMind Code Project.
*
* The SmallMind Code Project is free software, you can redistribute
* it and/or modify it under either, at your discretion...
*
* 1) The terms of GNU Affero General Public License as published by the
* Free Software Foundation, either version 3 of the License, or (at
* your option) any later version.
*
* ...or...
*
* 2) The terms of the Apache License, Version 2.0.
*
* The SmallMind Code Project is distributed in the hope that it will
* be useful, but WITHOUT ANY WARRANTY; without even the implied warranty
* of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* General Public License or Apache License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* and the Apache License along with the SmallMind Code Project. If not, see
* or .
*
* Additional permission under the GNU Affero GPL version 3 section 7
* ------------------------------------------------------------------
* If you modify this Program, or any covered work, by linking or
* combining it with other code, such other code is not for that reason
* alone subject to any of the requirements of the GNU Affero GPL
* version 3.
*/
package org.smallmind.persistence.cache.praxis;
import java.io.Serializable;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import org.smallmind.nutsnbolts.util.SingleItemIterable;
import org.smallmind.persistence.Durable;
import org.smallmind.persistence.cache.CacheOperationException;
import org.smallmind.persistence.cache.DurableKey;
import org.smallmind.persistence.cache.DurableVector;
import org.smallmind.persistence.orm.ORMDao;
import org.smallmind.persistence.orm.OrmDaoManager;
import org.terracotta.annotations.AutolockRead;
import org.terracotta.annotations.AutolockWrite;
import org.terracotta.annotations.InstrumentedClass;
@InstrumentedClass
public class ByKeySingularVector, D extends Durable> extends DurableVector {
private transient volatile ORMDao ormDao;
private DurableKey durableKey;
public ByKeySingularVector (DurableKey durableKey, int timeToLiveSeconds) {
super(null, 1, timeToLiveSeconds, false);
this.durableKey = durableKey;
}
private ORMDao getORMDao () {
if (ormDao == null) {
if ((ormDao = OrmDaoManager.get(durableKey.getDurableClass())) == null) {
throw new CacheOperationException("Unable to locate an implementation of ORMDao within DaoManager for the requested durable(%s)", durableKey.getDurableClass().getSimpleName());
}
}
return ormDao;
}
private D getDurable () {
D durable;
ORMDao ormDao;
if ((durable = (ormDao = getORMDao()).get(ormDao.getIdFromString(durableKey.getIdAsString()))) == null) {
throw new CacheOperationException("Unable to locate the requested durable(%s) instance(%s)", durableKey.getDurableClass().getSimpleName(), durableKey.getIdAsString());
}
return durable;
}
@AutolockRead
public DurableVector copy () {
return new ByKeySingularVector<>(durableKey, getTimeToLiveSeconds());
}
public boolean isSingular () {
return true;
}
@AutolockWrite
public synchronized boolean add (D durable) {
if (!getDurable().equals(durable)) {
durableKey = new DurableKey<>(durableKey.getDurableClass(), durable.getId());
return true;
}
return false;
}
public boolean remove (D durable) {
throw new UnsupportedOperationException("Attempted removal from a 'singular' vector");
}
@AutolockRead
public synchronized D head () {
return getDurable();
}
@AutolockRead
public synchronized List asBestEffortLazyList () {
return Collections.singletonList(getDurable());
}
@AutolockRead
public synchronized Iterator iterator () {
return new SingleItemIterable<>(getDurable()).iterator();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy