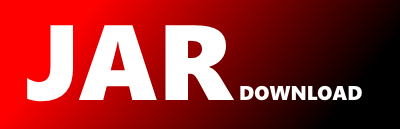
org.smart4j.framework.dao.DatabaseHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smart-framework Show documentation
Show all versions of smart-framework Show documentation
Smart Framework is a lightweight Java Web Framework
package org.smart4j.framework.dao;
import java.io.File;
import java.io.Serializable;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
import java.util.Map;
import javax.sql.DataSource;
import org.apache.commons.io.FileUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.smart4j.framework.InstanceFactory;
import org.smart4j.framework.core.ConfigHelper;
import org.smart4j.framework.ds.DataSourceFactory;
import org.smart4j.framework.util.ClassUtil;
/**
* 封装数据库相关操作
*
* @author huangyong
* @since 1.0
*/
public class DatabaseHelper {
private static final Logger logger = LoggerFactory.getLogger(DatabaseHelper.class);
/**
* 定义一个局部线程变量(使每个线程都拥有自己的连接)
*/
private static final ThreadLocal connContainer = new ThreadLocal();
/**
* 获取数据源工厂
*/
private static final DataSourceFactory dataSourceFactory = InstanceFactory.getDataSourceFactory();
/**
* 获取数据访问器
*/
private static final DataAccessor dataAccessor = InstanceFactory.getDataAccessor();
/**
* 数据库类型
*/
private static final String databaseType = ConfigHelper.getString("smart.framework.jdbc.type");
/**
* 获取数据库类型
*/
public static String getDatabaseType() {
return databaseType;
}
/**
* 获取数据源
*/
public static DataSource getDataSource() {
return dataSourceFactory.getDataSource();
}
/**
* 获取数据库连接
*/
public static Connection getConnection() {
Connection conn;
try {
// 先从 ThreadLocal 中获取 Connection
conn = connContainer.get();
if (conn == null) {
// 若不存在,则从 DataSource 中获取 Connection
conn = getDataSource().getConnection();
// 将 Connection 放入 ThreadLocal 中
if (conn != null) {
connContainer.set(conn);
}
}
} catch (SQLException e) {
logger.error("获取数据库连接出错!", e);
throw new RuntimeException(e);
}
return conn;
}
/**
* 开启事务
*/
public static void beginTransaction() {
Connection conn = getConnection();
if (conn != null) {
try {
conn.setAutoCommit(false);
} catch (SQLException e) {
logger.error("开启事务出错!", e);
throw new RuntimeException(e);
} finally {
connContainer.set(conn);
}
}
}
/**
* 提交事务
*/
public static void commitTransaction() {
Connection conn = getConnection();
if (conn != null) {
try {
conn.commit();
conn.close();
} catch (SQLException e) {
logger.error("提交事务出错!", e);
throw new RuntimeException(e);
} finally {
connContainer.remove();
}
}
}
/**
* 回滚事务
*/
public static void rollbackTransaction() {
Connection conn = getConnection();
if (conn != null) {
try {
conn.rollback();
conn.close();
} catch (SQLException e) {
logger.error("回滚事务出错!", e);
throw new RuntimeException(e);
} finally {
connContainer.remove();
}
}
}
/**
* 初始化 SQL 脚本
*/
public static void initSQL(String sqlPath) {
try {
File sqlFile = new File(ClassUtil.getClassPath() + sqlPath);
List sqlList = FileUtils.readLines(sqlFile);
for (String sql : sqlList) {
update(sql);
}
} catch (Exception e) {
logger.error("初始化 SQL 脚本出错!", e);
throw new RuntimeException(e);
}
}
/**
* 根据 SQL 语句查询 Entity
*/
public static T queryEntity(Class entityClass, String sql, Object... params) {
return dataAccessor.queryEntity(entityClass, sql, params);
}
/**
* 根据 SQL 语句查询 Entity 列表
*/
public static List queryEntityList(Class entityClass, String sql, Object... params) {
return dataAccessor.queryEntityList(entityClass, sql, params);
}
/**
* 根据 SQL 语句查询 Entity 映射(Field Name => Field Value)
*/
public static Map queryEntityMap(Class entityClass, String sql, Object... params) {
return dataAccessor.queryEntityMap(entityClass, sql, params);
}
/**
* 根据 SQL 语句查询 Array 格式的字段(单条记录)
*/
public static Object[] queryArray(String sql, Object... params) {
return dataAccessor.queryArray(sql, params);
}
/**
* 根据 SQL 语句查询 Array 格式的字段列表(多条记录)
*/
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy