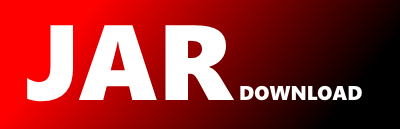
org.smooks.edifact.binding.service.ObjectFactory Maven / Gradle / Ivy
/*-
* ========================LICENSE_START=================================
* service-edifact-binding
* %%
* Copyright (C) 2020 - 2024 Smooks
* %%
* Licensed under the terms of the Apache License Version 2.0, or
* the GNU Lesser General Public License version 3.0 or later.
*
* SPDX-License-Identifier: Apache-2.0 OR LGPL-3.0-or-later
*
* ======================================================================
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* ======================================================================
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software Foundation,
* Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
* =========================LICENSE_END==================================
*/
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.11.12 at 08:58:01 AM UTC
//
package org.smooks.edifact.binding.service;
import javax.xml.namespace.QName;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.annotation.XmlElementDecl;
import jakarta.xml.bind.annotation.XmlRegistry;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the org.smooks.edifact.binding.service package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _AUTACK_QNAME = new QName("http://www.ibm.com/dfdl/edi/un/service/4.1", "AUTACK");
private final static QName _CONTRL_QNAME = new QName("http://www.ibm.com/dfdl/edi/un/service/4.1", "CONTRL");
private final static QName _KEYMAN_QNAME = new QName("http://www.ibm.com/dfdl/edi/un/service/4.1", "KEYMAN");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: org.smooks.edifact.binding.service
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link KEYMAN }
*
*/
public KEYMAN createKEYMAN() {
return new KEYMAN();
}
/**
* Create an instance of {@link KEYMAN.SegGrp4 }
*
*/
public KEYMAN.SegGrp4 createKEYMANSegGrp4() {
return new KEYMAN.SegGrp4();
}
/**
* Create an instance of {@link KEYMAN.SegGrp1 }
*
*/
public KEYMAN.SegGrp1 createKEYMANSegGrp1() {
return new KEYMAN.SegGrp1();
}
/**
* Create an instance of {@link KEYMAN.SegGrp1 .SegGrp2 }
*
*/
public KEYMAN.SegGrp1 .SegGrp2 createKEYMANSegGrp1SegGrp2() {
return new KEYMAN.SegGrp1 .SegGrp2();
}
/**
* Create an instance of {@link CONTRL }
*
*/
public CONTRL createCONTRL() {
return new CONTRL();
}
/**
* Create an instance of {@link CONTRL.SegGrp3 }
*
*/
public CONTRL.SegGrp3 createCONTRLSegGrp3() {
return new CONTRL.SegGrp3();
}
/**
* Create an instance of {@link CONTRL.SegGrp3 .SegGrp4 }
*
*/
public CONTRL.SegGrp3 .SegGrp4 createCONTRLSegGrp3SegGrp4() {
return new CONTRL.SegGrp3 .SegGrp4();
}
/**
* Create an instance of {@link CONTRL.SegGrp1 }
*
*/
public CONTRL.SegGrp1 createCONTRLSegGrp1() {
return new CONTRL.SegGrp1();
}
/**
* Create an instance of {@link AUTACK }
*
*/
public AUTACK createAUTACK() {
return new AUTACK();
}
/**
* Create an instance of {@link AUTACK.SegGrp1 }
*
*/
public AUTACK.SegGrp1 createAUTACKSegGrp1() {
return new AUTACK.SegGrp1();
}
/**
* Create an instance of {@link UNA }
*
*/
public UNA createUNA() {
return new UNA();
}
/**
* Create an instance of {@link UCDDataElementErrorIndication }
*
*/
public UCDDataElementErrorIndication createUCDDataElementErrorIndication() {
return new UCDDataElementErrorIndication();
}
/**
* Create an instance of {@link UCFGroupResponse }
*
*/
public UCFGroupResponse createUCFGroupResponse() {
return new UCFGroupResponse();
}
/**
* Create an instance of {@link UCIInterchangeResponse }
*
*/
public UCIInterchangeResponse createUCIInterchangeResponse() {
return new UCIInterchangeResponse();
}
/**
* Create an instance of {@link UCMMessagePackageResponse }
*
*/
public UCMMessagePackageResponse createUCMMessagePackageResponse() {
return new UCMMessagePackageResponse();
}
/**
* Create an instance of {@link UCSSegmentErrorIndication }
*
*/
public UCSSegmentErrorIndication createUCSSegmentErrorIndication() {
return new UCSSegmentErrorIndication();
}
/**
* Create an instance of {@link UGHAntiCollisionSegmentGroupHeader }
*
*/
public UGHAntiCollisionSegmentGroupHeader createUGHAntiCollisionSegmentGroupHeader() {
return new UGHAntiCollisionSegmentGroupHeader();
}
/**
* Create an instance of {@link UGTAntiCollisionSegmentGroupTrailer }
*
*/
public UGTAntiCollisionSegmentGroupTrailer createUGTAntiCollisionSegmentGroupTrailer() {
return new UGTAntiCollisionSegmentGroupTrailer();
}
/**
* Create an instance of {@link UIBInteractiveInterchangeHeader }
*
*/
public UIBInteractiveInterchangeHeader createUIBInteractiveInterchangeHeader() {
return new UIBInteractiveInterchangeHeader();
}
/**
* Create an instance of {@link UIHInteractiveMessageHeader }
*
*/
public UIHInteractiveMessageHeader createUIHInteractiveMessageHeader() {
return new UIHInteractiveMessageHeader();
}
/**
* Create an instance of {@link UIRInteractiveStatus }
*
*/
public UIRInteractiveStatus createUIRInteractiveStatus() {
return new UIRInteractiveStatus();
}
/**
* Create an instance of {@link UITInteractiveMessageTrailer }
*
*/
public UITInteractiveMessageTrailer createUITInteractiveMessageTrailer() {
return new UITInteractiveMessageTrailer();
}
/**
* Create an instance of {@link UIZInteractiveInterchangeTrailer }
*
*/
public UIZInteractiveInterchangeTrailer createUIZInteractiveInterchangeTrailer() {
return new UIZInteractiveInterchangeTrailer();
}
/**
* Create an instance of {@link UNBInterchangeHeader }
*
*/
public UNBInterchangeHeader createUNBInterchangeHeader() {
return new UNBInterchangeHeader();
}
/**
* Create an instance of {@link UNEGroupTrailer }
*
*/
public UNEGroupTrailer createUNEGroupTrailer() {
return new UNEGroupTrailer();
}
/**
* Create an instance of {@link UNGGroupHeader }
*
*/
public UNGGroupHeader createUNGGroupHeader() {
return new UNGGroupHeader();
}
/**
* Create an instance of {@link UNHMessageHeader }
*
*/
public UNHMessageHeader createUNHMessageHeader() {
return new UNHMessageHeader();
}
/**
* Create an instance of {@link UNOObjectHeader }
*
*/
public UNOObjectHeader createUNOObjectHeader() {
return new UNOObjectHeader();
}
/**
* Create an instance of {@link UNPObjectTrailer }
*
*/
public UNPObjectTrailer createUNPObjectTrailer() {
return new UNPObjectTrailer();
}
/**
* Create an instance of {@link UNSSectionControl }
*
*/
public UNSSectionControl createUNSSectionControl() {
return new UNSSectionControl();
}
/**
* Create an instance of {@link UNTMessageTrailer }
*
*/
public UNTMessageTrailer createUNTMessageTrailer() {
return new UNTMessageTrailer();
}
/**
* Create an instance of {@link UNZInterchangeTrailer }
*
*/
public UNZInterchangeTrailer createUNZInterchangeTrailer() {
return new UNZInterchangeTrailer();
}
/**
* Create an instance of {@link USASecurityAlgorithm }
*
*/
public USASecurityAlgorithm createUSASecurityAlgorithm() {
return new USASecurityAlgorithm();
}
/**
* Create an instance of {@link USBSecuredDataIdentification }
*
*/
public USBSecuredDataIdentification createUSBSecuredDataIdentification() {
return new USBSecuredDataIdentification();
}
/**
* Create an instance of {@link USCCertificate }
*
*/
public USCCertificate createUSCCertificate() {
return new USCCertificate();
}
/**
* Create an instance of {@link USDDataEncryptionHeader }
*
*/
public USDDataEncryptionHeader createUSDDataEncryptionHeader() {
return new USDDataEncryptionHeader();
}
/**
* Create an instance of {@link USESecurityMessageRelation }
*
*/
public USESecurityMessageRelation createUSESecurityMessageRelation() {
return new USESecurityMessageRelation();
}
/**
* Create an instance of {@link USFKeyManagementFunction }
*
*/
public USFKeyManagementFunction createUSFKeyManagementFunction() {
return new USFKeyManagementFunction();
}
/**
* Create an instance of {@link USHSecurityHeader }
*
*/
public USHSecurityHeader createUSHSecurityHeader() {
return new USHSecurityHeader();
}
/**
* Create an instance of {@link USLSecurityListStatus }
*
*/
public USLSecurityListStatus createUSLSecurityListStatus() {
return new USLSecurityListStatus();
}
/**
* Create an instance of {@link USRSecurityResult }
*
*/
public USRSecurityResult createUSRSecurityResult() {
return new USRSecurityResult();
}
/**
* Create an instance of {@link USTSecurityTrailer }
*
*/
public USTSecurityTrailer createUSTSecurityTrailer() {
return new USTSecurityTrailer();
}
/**
* Create an instance of {@link USUDataEncryptionTrailer }
*
*/
public USUDataEncryptionTrailer createUSUDataEncryptionTrailer() {
return new USUDataEncryptionTrailer();
}
/**
* Create an instance of {@link USXSecurityReferences }
*
*/
public USXSecurityReferences createUSXSecurityReferences() {
return new USXSecurityReferences();
}
/**
* Create an instance of {@link USYSecurityOnReferences }
*
*/
public USYSecurityOnReferences createUSYSecurityOnReferences() {
return new USYSecurityOnReferences();
}
/**
* Create an instance of {@link S001SyntaxIdentifier }
*
*/
public S001SyntaxIdentifier createS001SyntaxIdentifier() {
return new S001SyntaxIdentifier();
}
/**
* Create an instance of {@link S002InterchangeSender }
*
*/
public S002InterchangeSender createS002InterchangeSender() {
return new S002InterchangeSender();
}
/**
* Create an instance of {@link S003InterchangeRecipient }
*
*/
public S003InterchangeRecipient createS003InterchangeRecipient() {
return new S003InterchangeRecipient();
}
/**
* Create an instance of {@link S004DateAndTimeOfPreparation }
*
*/
public S004DateAndTimeOfPreparation createS004DateAndTimeOfPreparation() {
return new S004DateAndTimeOfPreparation();
}
/**
* Create an instance of {@link S005RecipientReferencePasswordDetails }
*
*/
public S005RecipientReferencePasswordDetails createS005RecipientReferencePasswordDetails() {
return new S005RecipientReferencePasswordDetails();
}
/**
* Create an instance of {@link S006ApplicationSenderIdentification }
*
*/
public S006ApplicationSenderIdentification createS006ApplicationSenderIdentification() {
return new S006ApplicationSenderIdentification();
}
/**
* Create an instance of {@link S007ApplicationRecipientIdentification }
*
*/
public S007ApplicationRecipientIdentification createS007ApplicationRecipientIdentification() {
return new S007ApplicationRecipientIdentification();
}
/**
* Create an instance of {@link S008MessageVersion }
*
*/
public S008MessageVersion createS008MessageVersion() {
return new S008MessageVersion();
}
/**
* Create an instance of {@link S009MessageIdentifier }
*
*/
public S009MessageIdentifier createS009MessageIdentifier() {
return new S009MessageIdentifier();
}
/**
* Create an instance of {@link S010StatusOfTheTransfer }
*
*/
public S010StatusOfTheTransfer createS010StatusOfTheTransfer() {
return new S010StatusOfTheTransfer();
}
/**
* Create an instance of {@link S011DataElementIdentification }
*
*/
public S011DataElementIdentification createS011DataElementIdentification() {
return new S011DataElementIdentification();
}
/**
* Create an instance of {@link S016MessageSubsetIdentification }
*
*/
public S016MessageSubsetIdentification createS016MessageSubsetIdentification() {
return new S016MessageSubsetIdentification();
}
/**
* Create an instance of {@link S017MessageImplementationGuidelineIdentification }
*
*/
public S017MessageImplementationGuidelineIdentification createS017MessageImplementationGuidelineIdentification() {
return new S017MessageImplementationGuidelineIdentification();
}
/**
* Create an instance of {@link S018ScenarioIdentification }
*
*/
public S018ScenarioIdentification createS018ScenarioIdentification() {
return new S018ScenarioIdentification();
}
/**
* Create an instance of {@link S020ReferenceIdentification }
*
*/
public S020ReferenceIdentification createS020ReferenceIdentification() {
return new S020ReferenceIdentification();
}
/**
* Create an instance of {@link S021ObjectTypeIdentification }
*
*/
public S021ObjectTypeIdentification createS021ObjectTypeIdentification() {
return new S021ObjectTypeIdentification();
}
/**
* Create an instance of {@link S022StatusOfTheObject }
*
*/
public S022StatusOfTheObject createS022StatusOfTheObject() {
return new S022StatusOfTheObject();
}
/**
* Create an instance of {@link S300DateAndOrTimeOfInitiation }
*
*/
public S300DateAndOrTimeOfInitiation createS300DateAndOrTimeOfInitiation() {
return new S300DateAndOrTimeOfInitiation();
}
/**
* Create an instance of {@link S301StatusOfTransferInteractive }
*
*/
public S301StatusOfTransferInteractive createS301StatusOfTransferInteractive() {
return new S301StatusOfTransferInteractive();
}
/**
* Create an instance of {@link S302DialogueReference }
*
*/
public S302DialogueReference createS302DialogueReference() {
return new S302DialogueReference();
}
/**
* Create an instance of {@link S303TransactionReference }
*
*/
public S303TransactionReference createS303TransactionReference() {
return new S303TransactionReference();
}
/**
* Create an instance of {@link S305DialogueIdentification }
*
*/
public S305DialogueIdentification createS305DialogueIdentification() {
return new S305DialogueIdentification();
}
/**
* Create an instance of {@link S306InteractiveMessageIdentifier }
*
*/
public S306InteractiveMessageIdentifier createS306InteractiveMessageIdentifier() {
return new S306InteractiveMessageIdentifier();
}
/**
* Create an instance of {@link S307StatusInformation }
*
*/
public S307StatusInformation createS307StatusInformation() {
return new S307StatusInformation();
}
/**
* Create an instance of {@link S500SecurityIdentificationDetails }
*
*/
public S500SecurityIdentificationDetails createS500SecurityIdentificationDetails() {
return new S500SecurityIdentificationDetails();
}
/**
* Create an instance of {@link S501SecurityDateAndTime }
*
*/
public S501SecurityDateAndTime createS501SecurityDateAndTime() {
return new S501SecurityDateAndTime();
}
/**
* Create an instance of {@link S502SecurityAlgorithm }
*
*/
public S502SecurityAlgorithm createS502SecurityAlgorithm() {
return new S502SecurityAlgorithm();
}
/**
* Create an instance of {@link S503AlgorithmParameter }
*
*/
public S503AlgorithmParameter createS503AlgorithmParameter() {
return new S503AlgorithmParameter();
}
/**
* Create an instance of {@link S504ListParameter }
*
*/
public S504ListParameter createS504ListParameter() {
return new S504ListParameter();
}
/**
* Create an instance of {@link S505ServiceCharacterForSignature }
*
*/
public S505ServiceCharacterForSignature createS505ServiceCharacterForSignature() {
return new S505ServiceCharacterForSignature();
}
/**
* Create an instance of {@link S508ValidationResult }
*
*/
public S508ValidationResult createS508ValidationResult() {
return new S508ValidationResult();
}
/**
* Create an instance of {@link KEYMAN.SegGrp4 .SegGrp5 }
*
*/
public KEYMAN.SegGrp4 .SegGrp5 createKEYMANSegGrp4SegGrp5() {
return new KEYMAN.SegGrp4 .SegGrp5();
}
/**
* Create an instance of {@link KEYMAN.SegGrp1 .SegGrp2 .SegGrp3 }
*
*/
public KEYMAN.SegGrp1 .SegGrp2 .SegGrp3 createKEYMANSegGrp1SegGrp2SegGrp3() {
return new KEYMAN.SegGrp1 .SegGrp2 .SegGrp3();
}
/**
* Create an instance of {@link CONTRL.SegGrp3 .SegGrp4 .SegGrp5 }
*
*/
public CONTRL.SegGrp3 .SegGrp4 .SegGrp5 createCONTRLSegGrp3SegGrp4SegGrp5() {
return new CONTRL.SegGrp3 .SegGrp4 .SegGrp5();
}
/**
* Create an instance of {@link CONTRL.SegGrp1 .SegGrp2 }
*
*/
public CONTRL.SegGrp1 .SegGrp2 createCONTRLSegGrp1SegGrp2() {
return new CONTRL.SegGrp1 .SegGrp2();
}
/**
* Create an instance of {@link AUTACK.SegGrp3 }
*
*/
public AUTACK.SegGrp3 createAUTACKSegGrp3() {
return new AUTACK.SegGrp3();
}
/**
* Create an instance of {@link AUTACK.SegGrp4 }
*
*/
public AUTACK.SegGrp4 createAUTACKSegGrp4() {
return new AUTACK.SegGrp4();
}
/**
* Create an instance of {@link AUTACK.SegGrp1 .SegGrp2 }
*
*/
public AUTACK.SegGrp1 .SegGrp2 createAUTACKSegGrp1SegGrp2() {
return new AUTACK.SegGrp1 .SegGrp2();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AUTACK }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link AUTACK }{@code >}
*/
@XmlElementDecl(namespace = "http://www.ibm.com/dfdl/edi/un/service/4.1", name = "AUTACK")
public JAXBElement createAUTACK(AUTACK value) {
return new JAXBElement(_AUTACK_QNAME, AUTACK.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link CONTRL }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link CONTRL }{@code >}
*/
@XmlElementDecl(namespace = "http://www.ibm.com/dfdl/edi/un/service/4.1", name = "CONTRL")
public JAXBElement createCONTRL(CONTRL value) {
return new JAXBElement(_CONTRL_QNAME, CONTRL.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link KEYMAN }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link KEYMAN }{@code >}
*/
@XmlElementDecl(namespace = "http://www.ibm.com/dfdl/edi/un/service/4.1", name = "KEYMAN")
public JAXBElement createKEYMAN(KEYMAN value) {
return new JAXBElement(_KEYMAN_QNAME, KEYMAN.class, null, value);
}
}