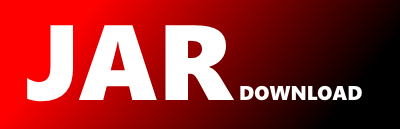
org.snakeyaml.engine.v2.events.ScalarEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snakeyaml-engine Show documentation
Show all versions of snakeyaml-engine Show documentation
Core YAML 1.2 parser and emitter for Java
The newest version!
/*
* Copyright (c) 2018, SnakeYAML
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package org.snakeyaml.engine.v2.events;
import java.util.Objects;
import java.util.Optional;
import java.util.stream.Collectors;
import org.snakeyaml.engine.v2.common.Anchor;
import org.snakeyaml.engine.v2.common.CharConstants;
import org.snakeyaml.engine.v2.common.ScalarStyle;
import org.snakeyaml.engine.v2.exceptions.Mark;
/**
* Marks a scalar value.
*/
public final class ScalarEvent extends NodeEvent {
private final Optional tag;
// style flag of a scalar event indicates the style of the scalar.
private final ScalarStyle style;
private final String value;
// The implicit flag of a scalar event is a pair of boolean values that
// indicate if the tag may be omitted when the scalar is emitted in a plain
// and non-plain style correspondingly.
private final ImplicitTuple implicit;
public ScalarEvent(Optional anchor, Optional tag, ImplicitTuple implicit,
String value, ScalarStyle style, Optional startMark, Optional endMark) {
super(anchor, startMark, endMark);
Objects.requireNonNull(tag);
this.tag = tag;
this.implicit = implicit;
Objects.requireNonNull(value);
this.value = value;
Objects.requireNonNull(style);
this.style = style;
}
public ScalarEvent(Optional anchor, Optional tag, ImplicitTuple implicit,
String value, ScalarStyle style) {
this(anchor, tag, implicit, value, style, Optional.empty(), Optional.empty());
}
/**
* Tag of this scalar.
*
* @return The tag of this scalar, or null
if no explicit tag is available.
*/
public Optional getTag() {
return this.tag;
}
/**
* Style of the scalar.
*
* - null
* - Flow Style - Plain
* - '\''
* - Flow Style - Single-Quoted
* - '"'
* - Flow Style - Double-Quoted
* - '|'
* - Block Style - Literal
* - '>'
* - Block Style - Folded
*
*
* @return Style of the scalar.
*/
public ScalarStyle getScalarStyle() {
return this.style;
}
/**
* String representation of the value.
*
* Without quotes and escaping.
*
*
* @return Value as Unicode string.
*/
public String getValue() {
return this.value;
}
public ImplicitTuple getImplicit() {
return this.implicit;
}
@Override
public ID getEventId() {
return ID.Scalar;
}
public boolean isPlain() {
return style == ScalarStyle.PLAIN;
}
public boolean isLiteral() {
return style == ScalarStyle.LITERAL;
}
public boolean isSQuoted() {
return style == ScalarStyle.SINGLE_QUOTED;
}
public boolean isDQuoted() {
return style == ScalarStyle.DOUBLE_QUOTED;
}
public boolean isFolded() {
return style == ScalarStyle.FOLDED;
}
public boolean isJson() {
return style == ScalarStyle.JSON_SCALAR_STYLE;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder("=VAL");
getAnchor().ifPresent(a -> builder.append(" &" + a));
if (implicit.bothFalse()) {
getTag().ifPresent(theTag -> builder.append(" <" + theTag + ">"));
}
builder.append(" ");
builder.append(getScalarStyle().toString());
builder.append(escapedValue());
return builder.toString();
}
// escape
public String escapedValue() {
return value.codePoints().filter(i -> i < Character.MAX_VALUE)
.mapToObj(ch -> CharConstants.escapeChar(String.valueOf(Character.toChars(ch))))
.collect(Collectors.joining(""));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy