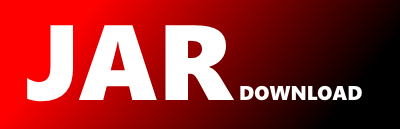
org.snapscript.studio.agent.runtime.RuntimeValueSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snap-agent Show documentation
Show all versions of snap-agent Show documentation
Dynamic scripting for the JVM
The newest version!
package org.snapscript.studio.agent.runtime;
import java.lang.reflect.Constructor;
import java.util.Arrays;
import java.util.List;
import java.util.concurrent.atomic.AtomicBoolean;
import org.snapscript.common.Cache;
import org.snapscript.common.CopyOnWriteCache;
import org.snapscript.common.LazyBuilder;
import org.snapscript.common.LazyCache;
import org.snapscript.common.LeastRecentlyUsedCache;
public class RuntimeValueSource {
private final LazyBuilder builder;
private final Cache cache;
private final Cache values;
public RuntimeValueSource(Class extends RuntimeValue>... types) {
this.builder = new RuntimeAttributeBuilder(types);
this.cache = new LazyCache(builder);
this.values = new LeastRecentlyUsedCache(1000);
}
public String getAttribute(String name) {
String value = values.fetch(name);
if(value == null) {
RuntimeValue attribute = cache.fetch(name);
String result = attribute.getValue();
values.cache(name, result);
return result;
}
return value;
}
private static class RuntimeAttributeBuilder implements LazyBuilder {
private final List> types;
private final Cache instances;
private final AtomicBoolean loaded;
public RuntimeAttributeBuilder(Class extends RuntimeValue>... types) {
this.instances = new CopyOnWriteCache();
this.loaded = new AtomicBoolean();
this.types = Arrays.asList(types);
}
@Override
public RuntimeValue create(String key) {
RuntimeValue value = instances.fetch(key);
if (value == null) {
return load(key);
}
return value;
}
private RuntimeValue load(String key) {
try {
if(!loaded.get()) {
for(Class extends RuntimeValue> type : types) {
RuntimeValue value = load(type);
String name = value.getName();
if(name != null) {
instances.cache(name, value);
}
}
loaded.set(true);
}
return instances.fetch(key);
} catch (Exception e) {
e.printStackTrace();
}
return new EmptyValue(key);
}
private RuntimeValue load(Class extends RuntimeValue> type) {
try {
Constructor extends RuntimeValue> constructor = type.getDeclaredConstructor();
if (!constructor.isAccessible()) {
constructor.setAccessible(true);
}
return constructor.newInstance();
} catch (Exception e) {
e.printStackTrace();
}
return new EmptyValue();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy