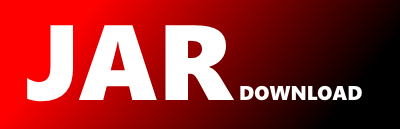
org.snmp4j.agent.example.SampleAgent Maven / Gradle / Ivy
/*_############################################################################
_##
_## SNMP4J-Agent 3 - SampleAgent.java
_##
_## Copyright (C) 2005-2018 Frank Fock (SNMP4J.org)
_##
_## Licensed under the Apache License, Version 2.0 (the "License");
_## you may not use this file except in compliance with the License.
_## You may obtain a copy of the License at
_##
_## http://www.apache.org/licenses/LICENSE-2.0
_##
_## Unless required by applicable law or agreed to in writing, software
_## distributed under the License is distributed on an "AS IS" BASIS,
_## WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
_## See the License for the specific language governing permissions and
_## limitations under the License.
_##
_##########################################################################*/
package org.snmp4j.agent.example;
import java.io.*;
import java.text.*;
import java.util.*;
import org.snmp4j.*;
import org.snmp4j.agent.*;
import org.snmp4j.agent.cfg.*;
import org.snmp4j.agent.io.*;
import org.snmp4j.agent.io.prop.*;
import org.snmp4j.agent.mo.*;
import org.snmp4j.agent.mo.snmp.dh.DHKickstartParameters;
import org.snmp4j.agent.mo.snmp.dh.DHKickstartParametersImpl;
import org.snmp4j.log.*;
import org.snmp4j.mp.*;
import org.snmp4j.security.dh.DHOperations;
import org.snmp4j.smi.*;
import org.snmp4j.transport.*;
import org.snmp4j.util.*;
import org.snmp4j.security.SecurityProtocols;
import org.snmp4j.agent.mo.util.VariableProvider;
import org.snmp4j.agent.request.SubRequest;
import org.snmp4j.agent.request.RequestStatus;
import org.snmp4j.agent.request.Request;
import org.snmp4j.agent.request.SubRequestIterator;
import org.snmp4j.agent.mo.snmp.TimeStamp;
/**
* The SampleAgent uses an {@link AgentConfigManager} instance to create a minimal SNMP agent using the configuration
* defined by {@code SampleAgentConfig.properties} in this package. That properties file defines the initial content of
* the registered MIB objects of this agent which may differ from the hard coded defaults.
*
* In order to add a new MIB object, call {@code server.register(..)} or replace the {@code Modules.java} file in this
* package by the {@code Modules.java} generated by AgenPro for your MIB module(s).
*
* The agent uses the {@link ConsoleLogFactory} to log messages.
*
* @author Frank Fock
* @version 3.2.1
*/
public class SampleAgent {
static {
LogFactory.setLogFactory(new ConsoleLogFactory());
LogFactory.getLogFactory().getRootLogger().setLogLevel(LogLevel.ALL);
}
private static final LogAdapter logger = LogFactory.getLogger(SampleAgent.class);
protected AgentConfigManager agent;
protected MOServer server;
private String configFile;
private File bootCounterFile;
// supported MIBs
protected Modules modules;
protected Properties tableSizeLimits;
@SuppressWarnings("unchecked")
public SampleAgent(Map> args) {
configFile = (String) (args.get("c")).get(0);
bootCounterFile = new File((String) (args.get("bc")).get(0));
List> tlsVersions = args.get("tls-version");
if (tlsVersions != null && (tlsVersions.size() > 0)) {
System.setProperty(SnmpConfigurator.P_TLS_VERSION, (String) tlsVersions.get(0));
}
server = new DefaultMOServer();
MOServer[] moServers = new MOServer[]{server};
InputStream configInputStream =
SampleAgent.class.getResourceAsStream("SampleAgentConfig.properties");
if (args.containsKey("cfg")) {
String configFilename = (String) ArgumentParser.getValue(args, "cfg", 0);
try {
configInputStream = new FileInputStream(configFilename);
} catch (FileNotFoundException ex1) {
logger.error("Config file '" + configFilename + "' not found: " + ex1.getMessage(), ex1);
throw new RuntimeException(ex1);
}
}
final Properties props = new Properties();
try {
props.load(configInputStream);
} catch (IOException ex) {
ex.printStackTrace();
}
MOInputFactory configurationFactory = new MOInputFactory() {
public MOInput createMOInput() {
return new PropertyMOInput(props, agent);
}
};
InputStream tableSizeLimitsInputStream =
SampleAgent.class.getResourceAsStream("SampleAgentTableSizeLimits.properties");
if (args.containsKey("ts")) {
try {
tableSizeLimitsInputStream =
new FileInputStream((String) ArgumentParser.getValue(args, "ts", 0));
} catch (FileNotFoundException ex1) {
ex1.printStackTrace();
}
}
tableSizeLimits = new Properties();
try {
tableSizeLimits.load(tableSizeLimitsInputStream);
} catch (IOException ex) {
ex.printStackTrace();
}
MessageDispatcher messageDispatcher = new MessageDispatcherImpl();
// Add TLSTM and DTLSM
try {
messageDispatcher.addTransportMapping(new TLSTM(new TlsAddress(), false));
messageDispatcher.addTransportMapping(new DTLSTM(new DtlsAddress(), false));
} catch (IOException e) {
logger.error("Exception while adding (D)TLS transport mappings: "+e.getMessage(), e);
}
addListenAddresses(messageDispatcher, args.get("address"));
Collection dhKickstartParameters = Collections.emptyList();
String dhKickstartInfoPath = (String) ArgumentParser.getFirstValue(args.get("dhks"));
if (dhKickstartInfoPath != null) {
File dhKickstartInfoFile = new File(dhKickstartInfoPath);
if (dhKickstartInfoFile.canRead()) {
try {
Properties kickstartProperties = new Properties();
FileInputStream fileInputStream = new FileInputStream(dhKickstartInfoFile);
kickstartProperties.load(fileInputStream);
fileInputStream.close();
dhKickstartParameters =
DHKickstartParametersImpl.readFromProperties("org.snmp4j.", kickstartProperties);
} catch (IOException iox) {
logger.error("Failed to load Diffie Hellman kickstart parameters from '" +
dhKickstartInfoPath + "': " + iox.getMessage(), iox);
}
} else {
logger.warn("Diffie Hellman kickstart parameters file cannot be read: " + dhKickstartInfoFile);
}
}
EngineBootsCounterFile engineBootsCounterFile = new EngineBootsCounterFile(bootCounterFile);
OctetString ownEngineId = engineBootsCounterFile.getEngineId(new OctetString(MPv3.createLocalEngineID()));
agent = new AgentConfigManager(ownEngineId,
messageDispatcher,
null,
moServers,
ThreadPool.create("SampleAgent", 3),
configurationFactory,
new DefaultMOPersistenceProvider(moServers, configFile), engineBootsCounterFile,
null, dhKickstartParameters);
}
protected void addListenAddresses(MessageDispatcher md, List