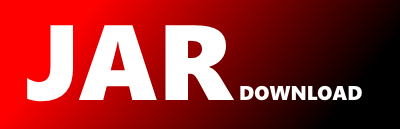
org.snmp4j.agent.AgentState Maven / Gradle / Ivy
/*_############################################################################
_##
_## SNMP4J-Agent 3 - AgentState.java
_##
_## Copyright (C) 2005-2021 Frank Fock (SNMP4J.org)
_##
_## Licensed under the Apache License, Version 2.0 (the "License");
_## you may not use this file except in compliance with the License.
_## You may obtain a copy of the License at
_##
_## http://www.apache.org/licenses/LICENSE-2.0
_##
_## Unless required by applicable law or agreed to in writing, software
_## distributed under the License is distributed on an "AS IS" BASIS,
_## WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
_## See the License for the specific language governing permissions and
_## limitations under the License.
_##
_##########################################################################*/
package org.snmp4j.agent;
import java.util.List;
/**
* The {@link AgentState} interface provides information about the state of a SNMP agent.
* States are represented by integer values. This allows implementing classes to extend
* states supported by an agent.
*
* @author Frank Fock
* @version 3.0.2
* @since 3.0
*/
public interface AgentState {
/**
* Agent has been created.
*/
int STATE_CREATED = 0;
/**
* Agent has been initialized.
*/
int STATE_INITIALIZED = 10;
/**
* Agent has been configured.
*/
int STATE_CONFIGURED = 20;
/**
* Agent's state has been restored.
*/
int STATE_RESTORED = 30;
/**
* Agent is suspended, i.e. does not accept/answer communication requests.
*/
int STATE_SUSPENDED = 35;
/**
* Agent is running.
*/
int STATE_RUNNING = 40;
/**
* Agent's state has been saved.
*/
int STATE_SAVED = 50;
/**
* Agent has been shutdown.
*/
int STATE_SHUTDOWN = -1;
/**
* Gets the current state of the agent.
* @return
* an integer representing the current state. See {@link #STATE_CREATED}.
*/
int getState();
/**
* Sets the new state independent from the current state.
*
* @param newState
* the new state.
*/
void setState(int newState);
/**
* Advance the state to the given state. If the current state is greater than
* the provided state, the current state will not be changed.
*
* @param newState the new minimum state.
*/
void advanceState(int newState);
/**
* Add an error description to the internal error list.
*
* @param error
* an ErrorDescriptor instance to add.
*/
void addError(ErrorDescriptor error);
/**
* Get the error descriptors associated with this agent state.
*
* @return the errors descriptor list.
*/
List getErrors();
/**
* The {@link ErrorDescriptor} provides detailed information about the agent state change problem.
*/
interface ErrorDescriptor {
/**
* Gets the error description.
* @return
* a description of the error.
*/
String getDescription();
/**
* The source state (before the error).
* @return
* a integer value between {@link #STATE_CREATED} and {@link #STATE_SAVED}.
*/
int getSourceState();
/**
* The target state (goal without error).
* @return
* a integer value between {@link #STATE_CREATED} and {@link #STATE_SAVED}.
*/
int getTargetState();
/**
* The exception that occurred.
* @return
* an exception.
*/
Exception getException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy