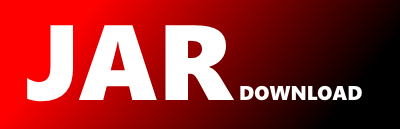
src.org.snmp4j.Session Maven / Gradle / Ivy
/*_############################################################################
_##
_## SNMP4J - Session.java
_##
_## Copyright (C) 2003-2008 Frank Fock and Jochen Katz (SNMP4J.org)
_##
_## Licensed under the Apache License, Version 2.0 (the "License");
_## you may not use this file except in compliance with the License.
_## You may obtain a copy of the License at
_##
_## http://www.apache.org/licenses/LICENSE-2.0
_##
_## Unless required by applicable law or agreed to in writing, software
_## distributed under the License is distributed on an "AS IS" BASIS,
_## WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
_## See the License for the specific language governing permissions and
_## limitations under the License.
_##
_##########################################################################*/
package org.snmp4j;
import org.snmp4j.event.*;
import java.io.IOException;
/**
* Session
defines a common interface for all classes that
* implement SNMP protocol operations based on SNMP4J.
*
* @author Frank Fock
* @version 1.2
*/
public interface Session {
/**
* Closes the session and frees any allocated resources, i.e. sockets.
* After a Session
has been closed it must
* be used.
* @throws IOException
* if the session could not free all resources.
*/
public void close() throws IOException;
/**
* Sends a PDU
to the given target and returns the received
* response PDU
.
* @param pdu
* the PDU
to send.
* @param target
* the Target
instance that specifies how and where to send
* the PDU.
* @return
* the received response encapsulated in a ResponseEvent
* instance. To obtain the received response PDU
call
* {@link ResponseEvent#getResponse()}. If the request timed out,
* that method will return null
. If the sent pdu
* is an unconfirmed PDU (notification, response, or report), then
* null
will be returned.
* @throws IOException
* if the message could not be send.
*/
public ResponseEvent send(PDU pdu, Target target) throws IOException;
/**
* Asynchronously sends a PDU
to the given target. The response
* is then returned by calling the supplied ResponseListener
* instance.
*
* @param pdu
* the PDU instance to send.
* @param target
* the Target instance representing the target SNMP engine where to send
* the pdu
.
* @param userHandle
* an user defined handle that is returned when the request is returned
* via the listener
object.
* @param listener
* a ResponseListener
instance that is called when
* pdu
is a confirmed PDU and the request is either answered
* or timed out.
* @throws IOException
* if the message could not be send.
*/
public void send(PDU pdu, Target target, Object userHandle,
ResponseListener listener) throws IOException;
/**
* Sends a PDU
to the given target and returns the received
* response PDU
encapsulated in a ResponseEvent
* object that also includes:
*
* - the transport address of the response sending peer,
*
- the
Target
information of the target,
* - the request
PDU
,
* - the response
PDU
(if any).
*
* @param pdu
* the PDU instance to send.
* @param target
* the Target instance representing the target SNMP engine where to send
* the pdu
.
* @param transport
* specifies the TransportMapping
to be used when sending
* the PDU. If transport
is null
, the associated
* message dispatcher will try to determine the transport mapping by the
* target
's address.
* @return
* the received response encapsulated in a ResponseEvent
* instance. To obtain the received response PDU
call
* {@link ResponseEvent#getResponse()}. If the request timed out,
* that method will return null
. If the sent pdu
* is an unconfirmed PDU (notification, response, or report), then
* null
will be returned.
* @throws IOException
* if the message could not be send.
*/
public ResponseEvent send(PDU pdu, Target target,
TransportMapping transport) throws IOException;
/**
* Asynchronously sends a PDU
to the given target. The response
* is then returned by calling the supplied ResponseListener
* instance.
*
* @param pdu
* the PDU instance to send.
* @param target
* the Target instance representing the target SNMP engine where to send
* the pdu
.
* @param transport
* specifies the TransportMapping
to be used when sending
* the PDU. If transport
is null
, the associated
* message dispatcher will try to determine the transport mapping by the
* target
's address.
* @param userHandle
* an user defined handle that is returned when the request is returned
* via the listener
object.
* @param listener
* a ResponseListener
instance that is called when
* pdu
is a confirmed PDU and the request is either answered
* or timed out.
* @throws IOException
* if the message could not be send.
*/
public void send(PDU pdu, Target target, TransportMapping transport,
Object userHandle,
ResponseListener listener) throws IOException;
/**
* Cancels an asynchronous request. Any asynchronous request must be canceled
* when the supplied response listener is being called, even if the
* ResponseEvent
indicates an error.
* @param request
* a request PDU as sent via {@link #send(PDU pdu, Target target,
* Object userHandle, ResponseListener listener)} or any .
* @param listener
* a ResponseListener instance.
*/
public void cancel(PDU request, ResponseListener listener);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy