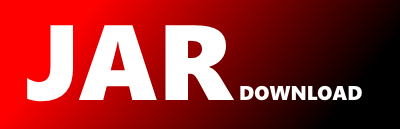
org.snmp4j.security.PrivacyProtocol Maven / Gradle / Ivy
/*_############################################################################
_##
_## SNMP4J 2 - PrivacyProtocol.java
_##
_## Copyright (C) 2003-2016 Frank Fock and Jochen Katz (SNMP4J.org)
_##
_## Licensed under the Apache License, Version 2.0 (the "License");
_## you may not use this file except in compliance with the License.
_## You may obtain a copy of the License at
_##
_## http://www.apache.org/licenses/LICENSE-2.0
_##
_## Unless required by applicable law or agreed to in writing, software
_## distributed under the License is distributed on an "AS IS" BASIS,
_## WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
_## See the License for the specific language governing permissions and
_## limitations under the License.
_##
_##########################################################################*/
package org.snmp4j.security;
import org.snmp4j.smi.OID;
import org.snmp4j.smi.OctetString;
/**
* The PrivacyProtocol
interface defines a common
* interface for all SNMP privacy protocols.
*
* @author Frank Fock
* @author Jochen Katz
* @version 1.9
*/
public interface PrivacyProtocol extends SecurityProtocol {
/**
* Encrypts a message using a given encryption key, engine boots count, and
* engine ID.
*
* @param unencryptedData
* the unencrypted data. This byte array may contain leading and trailing
* bytes that will not be encrypted.
* @param offset
* the offset into the unencryptedData
where to start
* encryption.
* @param length
* the length of the substring starting at offset
to encrypt.
* @param encryptionKey
* the key to be used for encryption.
* @param engineBoots
* the engine boots counter to use.
* @param engineTime
* the engine time to use.
* @param decryptParams
* returns the decryption parameters needed to decrypt the data that
* has been encrypted by this method.
* @return
* the encrypted copy of unencryptedData
.
*/
byte[] encrypt(byte[] unencryptedData,
int offset,
int length,
byte[] encryptionKey,
long engineBoots,
long engineTime,
DecryptParams decryptParams);
/**
* Decrypts a message using a given decryption key, engine boots count, and
* engine ID.
*
* @param cryptedData
* the crypted data. This byte array may contain leading and trailing
* bytes that will not be decrypted.
* @param offset
* the offset into the cryptedData
where to start
* encryption.
* @param length
* the length of the substring starting at offset
to decrypt.
* @param decryptionKey
* the key to be used for decryption.
* @param engineBoots
* the engine boots counter to use.
* @param engineTime
* the engine time to use.
* @param decryptParams
* contains the decryption parameters.
* @return
* the decrypted data, or null
if decryption failed.
*/
byte[] decrypt(byte[] cryptedData,
int offset,
int length,
byte[] decryptionKey,
long engineBoots,
long engineTime,
DecryptParams decryptParams);
/**
* Gets the OID uniquely identifying the privacy protocol.
* @return
* an OID
instance.
*/
OID getID();
/**
* Gets the length of a scoped PDU when encrypted with this security protocol.
* @param scopedPDULength
* the length of the (unencrypted) scoped PDU.
* @return
* the length of the encrypted scoped PDU.
*/
int getEncryptedLength(int scopedPDULength);
/**
* Gets the minimum key size for this privacy protocol.
* @return
* the minimum key size for this privacy protocol.
*/
int getMinKeyLength();
/**
* Gets the maximum key size for this privacy protocol.
* @return
* the minimum key size for this privacy protocol.
*/
int getMaxKeyLength();
/**
* Gets the length of the decryption parameters used by this security
* protocol.
* @return
* a positive integer denoting the length of decryption parameters returned
* by this security protocol.
*/
int getDecryptParamsLength();
/**
* Extend a localized key that is too short.
*
* Some privacy protocols require a key that is longer than the key
* generated by the password-to-key algorithm of the authentication
* protocol. This function extends a short key to the required length.
*
* @param shortKey
* the short key that was generated using
* {@link AuthenticationProtocol#passwordToKey} function.
* @param password
* the password to use for key extension.
* @param engineID
* the SNMP engine ID of the authoritative engine.
* @param authProtocol
* the authentication protocol that should be used.
* @return
* the extended key or shortKey
if no extension is needed.
* @since 1.9
*/
byte[] extendShortKey(byte[] shortKey,
OctetString password,
byte[] engineID,
AuthenticationProtocol authProtocol);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy