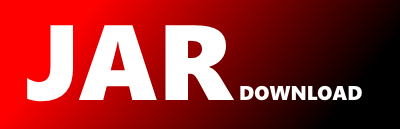
org.solovyev.android.http.DownloadFileAsyncTask Maven / Gradle / Ivy
package org.solovyev.android.http;
import android.content.Context;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.solovyev.android.RuntimeIoException;
import org.solovyev.android.async.CommonAsyncTask;
import org.solovyev.common.Converter;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
/**
* User: serso
* Date: 5/29/12
* Time: 9:50 PM
*/
public class DownloadFileAsyncTask extends CommonAsyncTask> {
@Nullable
private OnPostExecute> onPostExecute;
public DownloadFileAsyncTask(@NotNull Context context) {
super(context);
}
public DownloadFileAsyncTask(@NotNull Context context, @NotNull OnPostExecute> onPostExecute) {
super(context);
this.onPostExecute = onPostExecute;
}
@NotNull
@Override
protected List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy