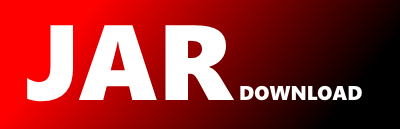
org.solovyev.android.http.AndroidHttpUtils Maven / Gradle / Ivy
package org.solovyev.android.http;
import com.loopj.android.http.AsyncHttpClient;
import com.loopj.android.http.AsyncHttpResponseHandler;
import com.loopj.android.http.RequestParams;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.methods.HttpUriRequest;
import org.apache.http.impl.client.DefaultHttpClient;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.solovyev.common.collections.CollectionsUtils;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* User: serso
* Date: 5/28/12
* Time: 2:17 PM
*/
public class AndroidHttpUtils {
private AndroidHttpUtils() {
throw new AssertionError();
}
public static R execute(@NotNull HttpTransaction httpTransaction) throws IOException {
return CollectionsUtils.getFirstListElement(execute(Arrays.asList(httpTransaction)));
}
@NotNull
public static List execute(@NotNull List extends HttpTransaction> httpTransactions) throws IOException {
final DefaultHttpClient httpClient = new DefaultHttpClient();
final List result = new ArrayList();
for (HttpTransaction httpTransaction : httpTransactions) {
final HttpUriRequest request = httpTransaction.createRequest();
final HttpResponse httpResponse = httpClient.execute(request);
final R response = httpTransaction.getResponse(httpResponse);
result.add(response);
}
return result;
}
public static void asyncExecute(@NotNull HttpTransactionDef httpTransactionDef,
@Nullable AsyncHttpResponseHandler responseHandle) throws IOException {
final AsyncHttpClient httpClient = new AsyncHttpClient();
final RequestParams requestParams = new RequestParams();
for (NameValuePair requestParam : httpTransactionDef.getRequestParameters()) {
requestParams.put(requestParam.getName(), requestParam.getValue());
}
httpTransactionDef.getHttpMethod().doAsyncRequest(httpClient, httpTransactionDef.getUri(), requestParams, responseHandle);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy