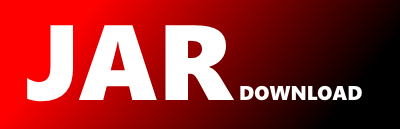
org.solovyev.common.collections.tree.TreeNode Maven / Gradle / Ivy
package org.solovyev.common.collections.tree;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.solovyev.common.JPredicate;
import java.util.Collection;
import java.util.Iterator;
/**
* User: serso
* Date: 4/1/12
* Time: 12:19 PM
*/
/**
* Class representing the element of the tree
*/
public interface TreeNode extends Iterable> {
@Nullable
TreeNode findOwnChild(@NotNull JPredicate> finder);
/**
* NOTE: immutable collection is returned
* @return OWN children of the node (first level children)
*/
@NotNull
Collection extends TreeNode> getOwnChildren();
/**
* @return iterator over the OWN children of the node (first level children).
* This iterator depending on the implementation may support or may not support java.util.Iterator#remove() method.
*/
@NotNull
Iterator extends TreeNode> getOwnChildrenIterator();
/**
* @return data stored with the object (may be null is no actual data is stored)
*/
@Nullable
T getData();
// NOTE: this method iterates through ALL children (children of children etc)
/**
* @return iterator over ALL children of the current node (children of children etc).
* This iterator depending on the implementation may support or may not support java.util.Iterator#remove() method.
* The actual traversal algorithm is not determined - it just guarantees iterating over all children
*/
@Override
@NotNull
Iterator> iterator();
@NotNull
Iterator extends TreeNode> getIterator();
@NotNull
Collection extends TreeNode> getAllChildren();
/**
* @return number of ALL children of current node
*/
int getSize();
/**
* @return true if current node is leaf (= has no children)
*/
boolean isLeaf();
/**
* @return true if current node is root (=has no parent)
*/
boolean isRoot();
/**
* @return depth of the current node in the tree (= number of parents of current node)
*/
int getDepth();
/**
* @return parent node to current node (if and only if root then result is null)
*/
@Nullable
TreeNode getParent();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy