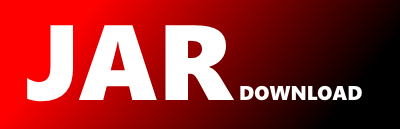
org.solovyev.common.collections.tree.TreeNodeImpl Maven / Gradle / Ivy
package org.solovyev.common.collections.tree;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.solovyev.common.JPredicate;
import org.solovyev.common.collections.CollectionsUtils;
import java.util.*;
/**
* User: serso
* Date: 4/1/12
* Time: 12:24 PM
*/
public class TreeNodeImpl implements MutableTreeNode {
@Nullable
private T data;
@Nullable
private TreeNode parent;
@NotNull
private Collection> children = new ArrayList>();
private TreeNodeImpl() {
}
@NotNull
public static TreeNodeImpl newInstance(@Nullable T data) {
final TreeNodeImpl result = new TreeNodeImpl();
result.data = data;
return result;
}
@Nullable
@Override
public MutableTreeNode findOwnChild(@NotNull JPredicate> finder) {
return CollectionsUtils.find(children.iterator(), finder);
}
@Override
public void setData(@Nullable T data) {
this.data = data;
}
@NotNull
@Override
public Collection> getOwnChildren() {
return Collections.unmodifiableCollection(children);
}
@NotNull
@Override
public Iterator extends MutableTreeNode> getOwnChildrenIterator() {
return this.children.iterator();
}
@NotNull
@Override
public Collection extends MutableTreeNode> getAllChildren() {
final Collection> result = new ArrayList>(children);
for (MutableTreeNode child : children) {
result.addAll(child.getAllChildren());
}
return result;
}
@Override
public void addChild(@NotNull MutableTreeNode node) {
node.setParent(this);
this.children.add(node);
}
@NotNull
@Override
public MutableTreeNode addChild(@NotNull T data) {
final TreeNodeImpl node = TreeNodeImpl.newInstance(data);
addChild(node);
return node;
}
@NotNull
@Override
public MutableTreeNode addChildIfNotExists(@NotNull final T data) {
MutableTreeNode result = this.findOwnChild(new JPredicate>() {
@Override
public boolean apply(@Nullable TreeNode input) {
return input != null && data.equals(input.getData());
}
});
if ( result == null ) {
result = this.addChild(data);
}
return result;
}
@Override
public void removeOwnChildIf(@NotNull JPredicate> predicate) {
CollectionsUtils.removeIf(this.children.iterator(), predicate);
}
@Override
public void removeChildIf(@NotNull JPredicate> predicate) {
CollectionsUtils.removeIf(this.iterator(), predicate);
}
@Nullable
@Override
public T getData() {
return this.data;
}
@NotNull
@Override
public Iterator> iterator() {
return new DepthTreeIterator(this.children);
}
@NotNull
@Override
public Iterator extends TreeNode> getIterator() {
return iterator();
}
@Override
public int getSize() {
int result = children.size();
for (MutableTreeNode child : children) {
result += child.getSize();
}
return result;
}
@Override
public boolean isLeaf() {
return this.children.isEmpty();
}
@Override
public boolean isRoot() {
return this.parent == null;
}
@Override
public int getDepth() {
int depth = 0;
TreeNode> parent = this.parent;
while( parent != null ) {
parent = parent.getParent();
depth++;
}
return depth;
}
@Override
public String toString() {
return "SimpleTreeNode{" +
"data=" + data +
", number of own children=" + children.size() +
'}';
}
@Override
@Nullable
public TreeNode getParent() {
return parent;
}
@Override
public void setParent(@Nullable TreeNode parent) {
this.parent = parent;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy