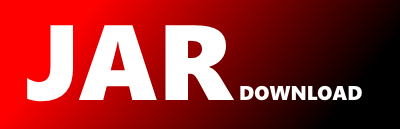
org.solovyev.common.collections.tree.LinkedTreeNode Maven / Gradle / Ivy
/*
* Copyright 2013 serso aka se.solovyev
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* ---------------------------------------------------------------------
* Contact details
*
* Email: [email protected]
* Site: http://se.solovyev.org
*/
package org.solovyev.common.collections.tree;
import org.solovyev.common.JPredicate;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
/**
* User: serso
* Date: 4/1/12
* Time: 12:24 PM
*/
class LinkedTreeNode implements MutableTreeNode {
@Nullable
private T value;
@Nullable
private TreeNode parent;
@Nonnull
private Collection> children = new ArrayList>();
private LinkedTreeNode() {
}
@Nonnull
static LinkedTreeNode newInstance(@Nullable T value) {
final LinkedTreeNode result = new LinkedTreeNode();
result.value = value;
return result;
}
@Nullable
@Override
public MutableTreeNode findOwnChild(@Nonnull JPredicate> finder) {
return org.solovyev.common.collections.Collections.find(children.iterator(), finder);
}
@Override
public void setValue(@Nullable T value) {
this.value = value;
}
@Nonnull
@Override
public Collection> getOwnChildren() {
return java.util.Collections.unmodifiableCollection(children);
}
@Nonnull
@Override
public Iterator extends MutableTreeNode> getOwnChildrenIterator() {
return this.children.iterator();
}
@Nonnull
@Override
public Collection extends MutableTreeNode> getAllChildren() {
final Collection> result = new ArrayList>(children);
for (MutableTreeNode child : children) {
result.addAll(child.getAllChildren());
}
return result;
}
@Override
public void addChild(@Nonnull MutableTreeNode node) {
node.setParent(this);
this.children.add(node);
}
@Nonnull
@Override
public MutableTreeNode addChild(@Nonnull T value) {
final LinkedTreeNode node = LinkedTreeNode.newInstance(value);
addChild(node);
return node;
}
@Nonnull
@Override
public MutableTreeNode addChildIfNotExists(@Nonnull final T value) {
MutableTreeNode result = this.findOwnChild(new JPredicate>() {
@Override
public boolean apply(@Nullable TreeNode input) {
return input != null && value.equals(input.getValue());
}
});
if (result == null) {
result = this.addChild(value);
}
return result;
}
@Override
public void removeOwnChildIf(@Nonnull JPredicate> predicate) {
org.solovyev.common.collections.Collections.removeIf(this.children.iterator(), predicate);
}
@Override
public void removeChildIf(@Nonnull JPredicate> predicate) {
org.solovyev.common.collections.Collections.removeIf(this.iterator(), predicate);
}
@Nullable
@Override
public T getValue() {
return this.value;
}
@Nonnull
@Override
public Iterator> iterator() {
return new DepthTreeIterator(this.children);
}
@Nonnull
@Override
public Iterator extends TreeNode> getIterator() {
return iterator();
}
@Override
public int getSize() {
int result = children.size();
for (MutableTreeNode child : children) {
result += child.getSize();
}
return result;
}
@Override
public boolean isLeaf() {
return this.children.isEmpty();
}
@Override
public boolean isRoot() {
return this.parent == null;
}
@Override
public int getDepth() {
int depth = 0;
TreeNode> parent = this.parent;
while (parent != null) {
parent = parent.getParent();
depth++;
}
return depth;
}
@Override
public String toString() {
return "SimpleTreeNode{" +
"value=" + value +
", number of own children=" + children.size() +
'}';
}
@Override
@Nullable
public TreeNode getParent() {
return parent;
}
@Override
public void setParent(@Nullable TreeNode parent) {
this.parent = parent;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy