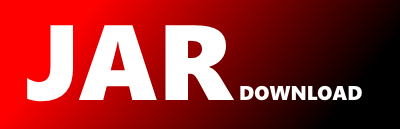
org.solovyev.common.collections.tree.UnmodifiableTreeNode Maven / Gradle / Ivy
/*
* Copyright 2013 serso aka se.solovyev
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* ---------------------------------------------------------------------
* Contact details
*
* Email: [email protected]
* Site: http://se.solovyev.org
*/
package org.solovyev.common.collections.tree;
import org.solovyev.common.JPredicate;
import org.solovyev.common.collections.UnmodifiableIterator;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.Collection;
import java.util.Iterator;
class UnmodifiableTreeNode implements MutableTreeNode {
/*
**********************************************************************
*
* FIELDS
*
**********************************************************************
*/
@Nonnull
private final MutableTreeNode treeNode;
/*
**********************************************************************
*
* CONSTRUCTORS
*
**********************************************************************
*/
private UnmodifiableTreeNode(@Nonnull MutableTreeNode treeNode) {
this.treeNode = treeNode;
}
@Nonnull
public static UnmodifiableTreeNode wrap(@Nonnull MutableTreeNode treeNode) {
return new UnmodifiableTreeNode(treeNode);
}
@Nonnull
public static UnmodifiableTreeNode wrap(@Nonnull TreeNode treeNode) {
if (treeNode instanceof MutableTreeNode) {
return wrap((MutableTreeNode) treeNode);
} else {
return new UnmodifiableTreeNode(TreeNodeAdapter.adapt(treeNode));
}
}
/*
**********************************************************************
*
* FIELDS
*
**********************************************************************
*/
@Nullable
public MutableTreeNode findOwnChild(@Nonnull JPredicate> finder) {
return treeNode.findOwnChild(finder);
}
public void setValue(@Nullable T value) {
throw new UnsupportedOperationException();
}
@Override
@Nonnull
public Collection extends MutableTreeNode> getOwnChildren() {
return Trees.unmodifiableMutableTreeNodes(treeNode.getOwnChildren());
}
@Override
@Nonnull
public Iterator extends MutableTreeNode> getOwnChildrenIterator() {
return UnmodifiableIterator.wrap(treeNode.getOwnChildrenIterator());
}
@Override
@Nonnull
public Collection extends MutableTreeNode> getAllChildren() {
return Trees.unmodifiableMutableTreeNodes(treeNode.getAllChildren());
}
public void addChild(@Nonnull MutableTreeNode node) {
throw new UnsupportedOperationException();
}
@Nonnull
public MutableTreeNode addChild(@Nonnull T value) {
throw new UnsupportedOperationException();
}
@Nonnull
public MutableTreeNode addChildIfNotExists(@Nonnull T value) {
throw new UnsupportedOperationException();
}
public void removeOwnChildIf(@Nonnull JPredicate> predicate) {
throw new UnsupportedOperationException();
}
public void removeChildIf(@Nonnull JPredicate> predicate) {
throw new UnsupportedOperationException();
}
public void setParent(@Nullable TreeNode parent) {
throw new UnsupportedOperationException();
}
@Override
@Nullable
public T getValue() {
return treeNode.getValue();
}
@Override
@Nonnull
public Iterator> iterator() {
return UnmodifiableIterator.wrap(treeNode.iterator());
}
@Override
@Nonnull
public Iterator extends TreeNode> getIterator() {
return UnmodifiableIterator.wrap(treeNode.getIterator());
}
@Override
public int getSize() {
return treeNode.getSize();
}
@Override
public boolean isLeaf() {
return treeNode.isLeaf();
}
@Override
public boolean isRoot() {
return treeNode.isRoot();
}
@Override
public int getDepth() {
return treeNode.getDepth();
}
@Override
@Nullable
public TreeNode getParent() {
final TreeNode result = treeNode.getParent();
if (result != null) {
return wrap(result);
} else {
return null;
}
}
/*
**********************************************************************
*
* STATIC
*
**********************************************************************
*/
private static class TreeNodeAdapter implements MutableTreeNode {
@Nonnull
private final TreeNode treeNode;
private TreeNodeAdapter(@Nonnull TreeNode treeNode) {
this.treeNode = treeNode;
}
@Nonnull
private static TreeNodeAdapter adapt(@Nonnull TreeNode treeNode) {
return new TreeNodeAdapter(treeNode);
}
@Nullable
public MutableTreeNode findOwnChild(@Nonnull JPredicate> finder) {
final TreeNode result = treeNode.findOwnChild(finder);
if (result != null) {
return wrap(result);
} else {
return null;
}
}
@Override
public void setValue(@Nullable T value) {
throw new AssertionError("Should never happen!");
}
@Override
@Nonnull
public Collection extends MutableTreeNode> getOwnChildren() {
return Trees.unmodifiableTreeNodes(treeNode.getOwnChildren());
}
@Override
@Nonnull
public Iterator extends MutableTreeNode> getOwnChildrenIterator() {
return UnmodifiableIterator.wrap(TreeNodeIteratorAdapter.adapt(treeNode.getOwnChildrenIterator()));
}
@Override
@Nullable
public T getValue() {
return treeNode.getValue();
}
@Override
@Nonnull
public Iterator> iterator() {
return UnmodifiableIterator.wrap(treeNode.iterator());
}
@Override
@Nonnull
public Iterator extends TreeNode> getIterator() {
return UnmodifiableIterator.wrap(treeNode.getIterator());
}
@Override
@Nonnull
public Collection extends MutableTreeNode> getAllChildren() {
return Trees.unmodifiableTreeNodes(treeNode.getAllChildren());
}
@Override
public void addChild(@Nonnull MutableTreeNode node) {
throw new AssertionError("Should never happen!");
}
@Nonnull
@Override
public MutableTreeNode addChild(@Nonnull T value) {
throw new AssertionError("Should never happen!");
}
@Nonnull
@Override
public MutableTreeNode addChildIfNotExists(@Nonnull T value) {
throw new AssertionError("Should never happen!");
}
@Override
public void removeOwnChildIf(@Nonnull JPredicate> predicate) {
throw new AssertionError("Should never happen!");
}
@Override
public void removeChildIf(@Nonnull JPredicate> predicate) {
throw new AssertionError("Should never happen!");
}
@Override
public void setParent(@Nullable TreeNode parent) {
throw new AssertionError("Should never happen!");
}
@Override
public int getSize() {
return treeNode.getSize();
}
@Override
public boolean isLeaf() {
return treeNode.isLeaf();
}
@Override
public boolean isRoot() {
return treeNode.isRoot();
}
@Override
public int getDepth() {
return treeNode.getDepth();
}
@Override
@Nullable
public TreeNode getParent() {
return treeNode.getParent();
}
}
private static class TreeNodeIteratorAdapter implements Iterator> {
@Nonnull
private final Iterator extends TreeNode> i;
private TreeNodeIteratorAdapter(@Nonnull Iterator extends TreeNode> i) {
this.i = i;
}
@Nonnull
private static Iterator> adapt(@Nonnull Iterator extends TreeNode> i) {
return new TreeNodeIteratorAdapter(i);
}
@Override
public boolean hasNext() {
return i.hasNext();
}
@Override
public MutableTreeNode next() {
return wrap(i.next());
}
@Override
public void remove() {
i.remove();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy