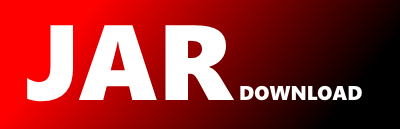
org.solovyev.common.math.graph.Graph Maven / Gradle / Ivy
The newest version!
package org.solovyev.common.math.graph;
import org.solovyev.common.JCloneable;
import org.solovyev.common.definitions.Empty;
import org.solovyev.common.definitions.MultiIdentity;
import org.solovyev.common.definitions.Property;
import org.solovyev.common.math.matrix.AbstractSparseMatrix;
import org.solovyev.common.math.matrix.Matrix;
import org.solovyev.common.clone.CloneUtils;
import org.solovyev.common.text.TextDisplay;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
/**
* User: serso
* Date: 28.03.2009
* Time: 15:55:54
*/
public class Graph implements TextDisplay, JCloneable> {
private List> nodes = new ArrayList>();
private Integer currentUsedId = MultiIdentity.defaulUsedId;
public Graph() {
}
public Graph(List> nodes) {
this.nodes = nodes;
}
public List> getNodes() {
return new ArrayList>(nodes);
}
public void setNodes(List> nodes) {
this.nodes = nodes;
}
public Graph(Matrix m) {
if (m != null && !m.isEmpty() && m.getNumberOfColumns() == m.getNumberOfRows()) {
Node node;
//creating nodes without linked nodes
for (int i = 0; i < m.getNumberOfRows(); i++) {
nodes.add(new Node(null, i));
}
N tmp;
//adding linked nodes
if (m instanceof AbstractSparseMatrix) {
List>> rows = ((AbstractSparseMatrix)m).getRows();
List> row;
for ( int i = 0; i < rows.size(); i++ ) {
row = rows.get(i);
if ( row != null ) {
node = this.nodes.get(i);
for ( Property el: row ) {
node.addLinkedNode(this.nodes.get(el.getId()), (N)el.getValue());
}
}
}
} else {
for (int i = 0; i < m.getNumberOfRows(); i++) {
node = this.nodes.get(i);
for (int j = 0; j < m.getNumberOfColumns(); j++) {
tmp = m.get(i, j);
if (tmp instanceof Empty) {
if (!((Empty) tmp).isEmpty()) {
node.addLinkedNode(this.nodes.get(j), tmp);
}
} else if (tmp instanceof Number) {
if (Math.abs(((Number) tmp).doubleValue()) > 0) {
node.addLinkedNode(this.nodes.get(j), tmp);
}
}
}
}
}
}
}
public Object[][] toMatrix() {
Object[][] result = new Object[this.nodes.size()][this.nodes.size()];
for (int i = 0; i < this.nodes.size(); i++) {
for (int j = 0; j < this.nodes.size(); j++) {
result[i][j] = 0d;
}
}
for (Node node : this.nodes) {
for (LinkedNode linkedNode : node.getLinkedNodes()) {
result[node.getId()][linkedNode.getNode().getId()] = linkedNode.getArc();
}
}
return result;
}
public void addNode(Node node) {
this.nodes.add(node);
}
public Node getNode(Integer id) {
Node node = null;
for (Node n : this.nodes) {
if (n.getId().equals(id)) {
node = n;
break;
}
}
return node;
}
public void deleteNode(Integer id) {
int i;
for (i = 0; i < this.nodes.size(); i++) {
if (this.nodes.get(i).getId().equals(id)) {
this.nodes.remove(i);
break;
}
}
//if node was found and deleted
if (i != this.nodes.size() - 1) {
//deleting links
for (Node n : this.nodes) {
n.deleteLinkedNode(id);
}
}
}
public void textDisplay(PrintWriter out) {
if (this.nodes.size() > 0) {
for (Node node : this.nodes) {
node.textDisplay(out);
out.println();
}
} else {
out.write("Graph is empty.");
out.println();
}
}
public List> getAdjSet(List> l) {
List> result = new ArrayList>();
for (Node node : l) {
for (LinkedNode n1 : node.getLinkedNodes()) {
if (!n1.getNode().isMarked()) {
n1.getNode().setMarked(true);
result.add(n1.getNode());
}
}
}
Collections.sort(result, new Graphs.NodesComparatorByDegree());
return result;
}
public void unmarkAll() {
for (Node node : this.getNodes()) {
node.setMarked(false);
}
}
@SuppressWarnings("unchecked")
public Graph clone() {
Graph result = null;
try {
result = (Graph) super.clone();
//nodes list
result.setNodes(CloneUtils.deepListCloning(this.getNodes()));
//adding linked nodes
for (Node node : this.nodes) {
for (LinkedNode linkedNode : node.getLinkedNodes()) {
result.nodes.get(node.getId()).addLinkedNode(result.nodes.get(linkedNode.getNode().getId()), linkedNode.getArc());
}
}
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
return result;
}
public void addNodeId() {
for (Node node : this.nodes) {
node.addNewId();
}
}
public void addNewId() {
//adding new id
for (Node node : this.nodes) {
//new id is the same as current
node.addNewId(node.getId());
}
}
public void setCurrentUsedId(Integer currentUsedId) {
this.currentUsedId = currentUsedId;
for (Node node : this.nodes) {
node.setCurrentUsedId(currentUsedId);
}
}
public Integer getCurrentUsedId() {
return currentUsedId;
}
public void sortNodes(Comparator> c) {
Collections.sort(this.nodes, c);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy