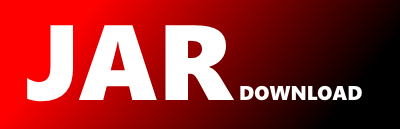
org.solovyev.common.math.graph.Node Maven / Gradle / Ivy
The newest version!
package org.solovyev.common.math.graph;
import org.solovyev.common.JCloneable;
import org.solovyev.common.definitions.IsMarked;
import org.solovyev.common.definitions.MultiIdentity;
import org.solovyev.common.text.TextDisplay;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* User: serso
* Date: 28.03.2009
* Time: 15:57:32
*/
public class Node extends MultiIdentity implements Comparable>, TextDisplay,
IsMarked, JCloneable> {
private List> linkedNodes = new ArrayList>();
private Object value;
private boolean isMarked = false;
public Node( T value, Integer id ) {
this( value, null, id );
}
public Node( T value, List> linkedNodes, List ids, Integer currentUsedId ) {
super (ids, currentUsedId);
this.value = value;
if ( linkedNodes != null ) {
this.linkedNodes.addAll(linkedNodes);
}
}
public Node( T value, List> linkedNodes, List ids ) {
this ( value, linkedNodes, ids, 0 );
}
public Node ( T value, List> linkedNodes, Integer id ) {
super(id);
this.value = value;
if ( linkedNodes != null ) {
this.linkedNodes.addAll(linkedNodes);
}
}
public List> getLinkedNodes() {
return linkedNodes;
}
public void setLinkedNodes(List> linkedNodes) {
this.linkedNodes = linkedNodes;
}
@SuppressWarnings("unchecked")
public T getValue() {
return (T)value;
}
public void setValue(T value) {
this.value = value;
}
public void addLinkedNode(Node node, N value) {
int i;
for ( i = this.linkedNodes.size() - 1; i >= 0; i-- ) {
if ( this.linkedNodes.get(i).getNode().getId() - node.getId() > 0 ) {
break;
}
}
if ( i == -1 ) {
this.linkedNodes.add(0, new LinkedNode(node, value));
} else {
this.linkedNodes.add(i, new LinkedNode(node, value));
}
//todo serso: check this place
//this really works faster
this.linkedNodes.add( new LinkedNode(node, value) );
Collections.sort( this.linkedNodes );
}
public int compareTo(Node o) {
int result = 0;
if ( o != null ) {
if ( this.getId() < o.getId() ) {
result = -1;
} else if ( this.getId() > o.getId() ) {
result = 1;
}
}
return result;
}
public void deleteLinkedNode( Integer id ) {
for ( int i = this.linkedNodes.size() - 1; i >= 0; i-- ) {
if ( this.linkedNodes.get( i ).getNode().getId().equals( id ) ) {
linkedNodes.remove( i );
break;
}
}
}
public void textDisplay(PrintWriter out, Boolean showLinkedNodes) {
out.write("Node: id=" + this.getId().toString() + ", value=" + ((this.value == null) ? "null" : value.toString()));
if ( showLinkedNodes ) {
out.write(" Linked Node Ids: ");
for (LinkedNode linkedNode : this.linkedNodes) {
out.write("(id: " + linkedNode.getNode().getId().toString() + ", dist: " + linkedNode.getArc().toString() + " )");
if (this.linkedNodes.indexOf(linkedNode) != this.linkedNodes.size() - 1) {
out.write(", ");
}
}
}
}
public void textDisplay(PrintWriter out) {
this.textDisplay(out, true);
}
public boolean isMarked() {
return this.isMarked;
}
public void setMarked( boolean isMarked) {
this.isMarked = isMarked;
}
@SuppressWarnings("unchecked")
public Node clone() {
Node result = null;
try {
result = (Node)super.clone();
result.setIds( new ArrayList( this.getIds() ) );
result.setLinkedNodes( new ArrayList>() );
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy