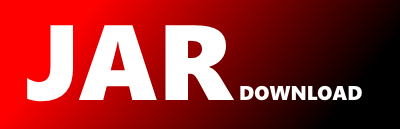
jscl.FixedCapacityListMessageRegistry Maven / Gradle / Ivy
package jscl;
import org.solovyev.common.msg.Message;
import org.solovyev.common.msg.MessageRegistry;
import javax.annotation.Nonnull;
import javax.annotation.concurrent.NotThreadSafe;
import java.util.ArrayList;
import java.util.List;
@NotThreadSafe
public class FixedCapacityListMessageRegistry implements MessageRegistry {
@Nonnull
private final List messages;
private final int capacity;
private volatile int size;
public FixedCapacityListMessageRegistry(int capacity) {
this.size = 0;
this.capacity = capacity;
this.messages = new ArrayList(capacity);
}
@edu.umd.cs.findbugs.annotations.SuppressWarnings(value = {"VO_VOLATILE_INCREMENT"}, justification = "NotThreadSafe - outer synchronization")
public void addMessage(@Nonnull Message message) {
if (!this.messages.contains(message)) {
if (this.size <= this.capacity) {
this.messages.add(message);
this.size++;
} else {
this.messages.remove(0);
this.messages.add(message);
}
}
}
@edu.umd.cs.findbugs.annotations.SuppressWarnings(value = {"VO_VOLATILE_INCREMENT"}, justification = "NotThreadSafe - outer synchronization")
@Nonnull
@Override
public Message getMessage() {
if (hasMessage()) {
this.size--;
return messages.remove(0);
} else {
throw new IllegalStateException("No messages!");
}
}
@Override
public boolean hasMessage() {
return size > 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy