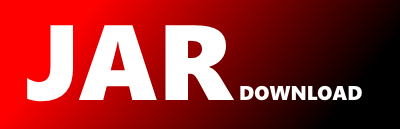
org.somda.sdc.biceps.model.participant.AbstractMetricValue Maven / Gradle / Ivy
package org.somda.sdc.biceps.model.participant;
import java.math.BigDecimal;
import java.time.Instant;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSeeAlso;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jetbrains.annotations.Nullable;
import org.jvnet.jaxb.lang.CopyStrategy;
import org.jvnet.jaxb.lang.CopyTo;
import org.jvnet.jaxb.lang.JAXBCopyStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
import org.somda.sdc.biceps.model.extension.ExtensionType;
import org.somda.sdc.common.model.TimestampAdapter;
/**
* Abstract value of a METRIC.
*
* Java class for AbstractMetricValue complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AbstractMetricValue", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/participant", propOrder = {
"extension",
"metricQuality",
"annotation"
})
@XmlSeeAlso({
NumericMetricValue.class,
StringMetricValue.class,
SampleArrayValue.class
})
public class AbstractMetricValue implements Cloneable, CopyTo, ToString
{
@XmlElement(name = "Extension", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/extension")
protected ExtensionType extension;
/**
* The quality state of the determined value of a METRIC.
*
*/
@XmlElement(name = "MetricQuality", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/participant", required = true)
protected AbstractMetricValue.MetricQuality metricQuality;
/**
* Annotation of a METRIC state value.
*
*/
@XmlElement(name = "Annotation", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/participant")
protected List annotation;
/**
* Time when measurement activity was started.
*
*/
@XmlAttribute(name = "StartTime")
@XmlJavaTypeAdapter(TimestampAdapter.class)
protected Instant startTime;
/**
* Time when measurement activity was stopped.
*
*/
@XmlAttribute(name = "StopTime")
@XmlJavaTypeAdapter(TimestampAdapter.class)
protected Instant stopTime;
/**
* Time when determined value has been derived from measurement.
*
*/
@XmlAttribute(name = "DeterminationTime")
@XmlJavaTypeAdapter(TimestampAdapter.class)
protected Instant determinationTime;
/**
* Gets the value of the extension property.
*
* @return
* possible object is
* {@link ExtensionType }
*
*/
@Nullable
public ExtensionType getExtension() {
return extension;
}
/**
* Sets the value of the extension property.
*
* @param value
* allowed object is
* {@link ExtensionType }
*
*/
public void setExtension(
@Nullable
ExtensionType value) {
this.extension = value;
}
/**
* The quality state of the determined value of a METRIC.
*
* @return
* possible object is
* {@link AbstractMetricValue.MetricQuality }
*
*/
public AbstractMetricValue.MetricQuality getMetricQuality() {
return metricQuality;
}
/**
* Sets the value of the metricQuality property.
*
* @param value
* allowed object is
* {@link AbstractMetricValue.MetricQuality }
*
* @see #getMetricQuality()
*/
public void setMetricQuality(AbstractMetricValue.MetricQuality value) {
this.metricQuality = value;
}
/**
* Annotation of a METRIC state value.
*
* Gets the value of the annotation property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the annotation property.
*
*
* For example, to add a new item, do as follows:
*
*
* getAnnotation().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AbstractMetricValue.Annotation }
*
*
*
* @return
* The value of the annotation property.
*/
public List getAnnotation() {
if (annotation == null) {
annotation = new ArrayList<>();
}
return this.annotation;
}
/**
* Time when measurement activity was started.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public Instant getStartTime() {
return startTime;
}
/**
* Sets the value of the startTime property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getStartTime()
*/
public void setStartTime(
@Nullable
Instant value) {
this.startTime = value;
}
/**
* Time when measurement activity was stopped.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public Instant getStopTime() {
return stopTime;
}
/**
* Sets the value of the stopTime property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getStopTime()
*/
public void setStopTime(
@Nullable
Instant value) {
this.stopTime = value;
}
/**
* Time when determined value has been derived from measurement.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public Instant getDeterminationTime() {
return determinationTime;
}
/**
* Sets the value of the determinationTime property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getDeterminationTime()
*/
public void setDeterminationTime(
@Nullable
Instant value) {
this.determinationTime = value;
}
public void setAnnotation(
@Nullable
List value) {
this.annotation = null;
if (value!= null) {
List draftl = this.getAnnotation();
draftl.addAll(value);
}
}
@Override
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final AbstractMetricValue that = ((AbstractMetricValue) object);
{
ExtensionType leftExtension;
leftExtension = this.getExtension();
ExtensionType rightExtension;
rightExtension = that.getExtension();
if (this.extension!= null) {
if (that.extension!= null) {
if (!leftExtension.equals(rightExtension)) {
return false;
}
} else {
return false;
}
} else {
if (that.extension!= null) {
return false;
}
}
}
{
AbstractMetricValue.MetricQuality leftMetricQuality;
leftMetricQuality = this.getMetricQuality();
AbstractMetricValue.MetricQuality rightMetricQuality;
rightMetricQuality = that.getMetricQuality();
if (this.metricQuality!= null) {
if (that.metricQuality!= null) {
if (!leftMetricQuality.equals(rightMetricQuality)) {
return false;
}
} else {
return false;
}
} else {
if (that.metricQuality!= null) {
return false;
}
}
}
{
List leftAnnotation;
leftAnnotation = (((this.annotation!= null)&&(!this.annotation.isEmpty()))?this.getAnnotation():null);
List rightAnnotation;
rightAnnotation = (((that.annotation!= null)&&(!that.annotation.isEmpty()))?that.getAnnotation():null);
if ((this.annotation!= null)&&(!this.annotation.isEmpty())) {
if ((that.annotation!= null)&&(!that.annotation.isEmpty())) {
if (!leftAnnotation.equals(rightAnnotation)) {
return false;
}
} else {
return false;
}
} else {
if ((that.annotation!= null)&&(!that.annotation.isEmpty())) {
return false;
}
}
}
{
Instant leftStartTime;
leftStartTime = this.getStartTime();
Instant rightStartTime;
rightStartTime = that.getStartTime();
if (this.startTime!= null) {
if (that.startTime!= null) {
if (!leftStartTime.equals(rightStartTime)) {
return false;
}
} else {
return false;
}
} else {
if (that.startTime!= null) {
return false;
}
}
}
{
Instant leftStopTime;
leftStopTime = this.getStopTime();
Instant rightStopTime;
rightStopTime = that.getStopTime();
if (this.stopTime!= null) {
if (that.stopTime!= null) {
if (!leftStopTime.equals(rightStopTime)) {
return false;
}
} else {
return false;
}
} else {
if (that.stopTime!= null) {
return false;
}
}
}
{
Instant leftDeterminationTime;
leftDeterminationTime = this.getDeterminationTime();
Instant rightDeterminationTime;
rightDeterminationTime = that.getDeterminationTime();
if (this.determinationTime!= null) {
if (that.determinationTime!= null) {
if (!leftDeterminationTime.equals(rightDeterminationTime)) {
return false;
}
} else {
return false;
}
} else {
if (that.determinationTime!= null) {
return false;
}
}
}
return true;
}
@Override
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
ExtensionType theExtension;
theExtension = this.getExtension();
if (this.extension!= null) {
currentHashCode += theExtension.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
AbstractMetricValue.MetricQuality theMetricQuality;
theMetricQuality = this.getMetricQuality();
if (this.metricQuality!= null) {
currentHashCode += theMetricQuality.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theAnnotation;
theAnnotation = (((this.annotation!= null)&&(!this.annotation.isEmpty()))?this.getAnnotation():null);
if ((this.annotation!= null)&&(!this.annotation.isEmpty())) {
currentHashCode += theAnnotation.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Instant theStartTime;
theStartTime = this.getStartTime();
if (this.startTime!= null) {
currentHashCode += theStartTime.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Instant theStopTime;
theStopTime = this.getStopTime();
if (this.stopTime!= null) {
currentHashCode += theStopTime.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Instant theDeterminationTime;
theDeterminationTime = this.getDeterminationTime();
if (this.determinationTime!= null) {
currentHashCode += theDeterminationTime.hashCode();
}
}
return currentHashCode;
}
@Override
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
ExtensionType theExtension;
theExtension = this.getExtension();
strategy.appendField(locator, this, "extension", buffer, theExtension, (this.extension!= null));
}
{
AbstractMetricValue.MetricQuality theMetricQuality;
theMetricQuality = this.getMetricQuality();
strategy.appendField(locator, this, "metricQuality", buffer, theMetricQuality, (this.metricQuality!= null));
}
{
List theAnnotation;
theAnnotation = (((this.annotation!= null)&&(!this.annotation.isEmpty()))?this.getAnnotation():null);
strategy.appendField(locator, this, "annotation", buffer, theAnnotation, ((this.annotation!= null)&&(!this.annotation.isEmpty())));
}
{
Instant theStartTime;
theStartTime = this.getStartTime();
strategy.appendField(locator, this, "startTime", buffer, theStartTime, (this.startTime!= null));
}
{
Instant theStopTime;
theStopTime = this.getStopTime();
strategy.appendField(locator, this, "stopTime", buffer, theStopTime, (this.stopTime!= null));
}
{
Instant theDeterminationTime;
theDeterminationTime = this.getDeterminationTime();
strategy.appendField(locator, this, "determinationTime", buffer, theDeterminationTime, (this.determinationTime!= null));
}
return buffer;
}
@Override
public Object clone() {
return copyTo(createNewInstance());
}
@Override
public Object copyTo(Object target) {
final CopyStrategy strategy = JAXBCopyStrategy.getInstance();
return copyTo(null, target, strategy);
}
@Override
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof AbstractMetricValue) {
final AbstractMetricValue copy = ((AbstractMetricValue) draftCopy);
{
Boolean extensionShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.extension!= null));
if (extensionShouldBeCopiedAndSet == Boolean.TRUE) {
ExtensionType sourceExtension;
sourceExtension = this.getExtension();
ExtensionType copyExtension = ((ExtensionType) strategy.copy(LocatorUtils.property(locator, "extension", sourceExtension), sourceExtension, (this.extension!= null)));
copy.setExtension(copyExtension);
} else {
if (extensionShouldBeCopiedAndSet == Boolean.FALSE) {
copy.extension = null;
}
}
}
{
Boolean metricQualityShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.metricQuality!= null));
if (metricQualityShouldBeCopiedAndSet == Boolean.TRUE) {
AbstractMetricValue.MetricQuality sourceMetricQuality;
sourceMetricQuality = this.getMetricQuality();
AbstractMetricValue.MetricQuality copyMetricQuality = ((AbstractMetricValue.MetricQuality) strategy.copy(LocatorUtils.property(locator, "metricQuality", sourceMetricQuality), sourceMetricQuality, (this.metricQuality!= null)));
copy.setMetricQuality(copyMetricQuality);
} else {
if (metricQualityShouldBeCopiedAndSet == Boolean.FALSE) {
copy.metricQuality = null;
}
}
}
{
Boolean annotationShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.annotation!= null)&&(!this.annotation.isEmpty())));
if (annotationShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceAnnotation;
sourceAnnotation = (((this.annotation!= null)&&(!this.annotation.isEmpty()))?this.getAnnotation():null);
@SuppressWarnings("unchecked")
List copyAnnotation = ((List ) strategy.copy(LocatorUtils.property(locator, "annotation", sourceAnnotation), sourceAnnotation, ((this.annotation!= null)&&(!this.annotation.isEmpty()))));
copy.setAnnotation(copyAnnotation);
} else {
if (annotationShouldBeCopiedAndSet == Boolean.FALSE) {
copy.annotation = null;
}
}
}
{
Boolean startTimeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.startTime!= null));
if (startTimeShouldBeCopiedAndSet == Boolean.TRUE) {
Instant sourceStartTime;
sourceStartTime = this.getStartTime();
Instant copyStartTime = ((Instant) strategy.copy(LocatorUtils.property(locator, "startTime", sourceStartTime), sourceStartTime, (this.startTime!= null)));
copy.setStartTime(copyStartTime);
} else {
if (startTimeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.startTime = null;
}
}
}
{
Boolean stopTimeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.stopTime!= null));
if (stopTimeShouldBeCopiedAndSet == Boolean.TRUE) {
Instant sourceStopTime;
sourceStopTime = this.getStopTime();
Instant copyStopTime = ((Instant) strategy.copy(LocatorUtils.property(locator, "stopTime", sourceStopTime), sourceStopTime, (this.stopTime!= null)));
copy.setStopTime(copyStopTime);
} else {
if (stopTimeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.stopTime = null;
}
}
}
{
Boolean determinationTimeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.determinationTime!= null));
if (determinationTimeShouldBeCopiedAndSet == Boolean.TRUE) {
Instant sourceDeterminationTime;
sourceDeterminationTime = this.getDeterminationTime();
Instant copyDeterminationTime = ((Instant) strategy.copy(LocatorUtils.property(locator, "determinationTime", sourceDeterminationTime), sourceDeterminationTime, (this.determinationTime!= null)));
copy.setDeterminationTime(copyDeterminationTime);
} else {
if (determinationTimeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.determinationTime = null;
}
}
}
}
return draftCopy;
}
@Override
public Object createNewInstance() {
return new AbstractMetricValue();
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"extension",
"type"
})
public static class Annotation implements Cloneable, CopyTo, ToString
{
@XmlElement(name = "Extension", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/extension")
protected ExtensionType extension;
/**
* The CODED VALUE that describes the annotation of the ELEMENT.
*
* Example: attach triggers in waveform curves.
*
*/
@XmlElement(name = "Type", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/participant", required = true)
protected CodedValue type;
/**
* Gets the value of the extension property.
*
* @return
* possible object is
* {@link ExtensionType }
*
*/
@Nullable
public ExtensionType getExtension() {
return extension;
}
/**
* Sets the value of the extension property.
*
* @param value
* allowed object is
* {@link ExtensionType }
*
*/
public void setExtension(
@Nullable
ExtensionType value) {
this.extension = value;
}
/**
* The CODED VALUE that describes the annotation of the ELEMENT.
*
* Example: attach triggers in waveform curves.
*
* @return
* possible object is
* {@link CodedValue }
*
*/
public CodedValue getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link CodedValue }
*
* @see #getType()
*/
public void setType(CodedValue value) {
this.type = value;
}
@Override
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final AbstractMetricValue.Annotation that = ((AbstractMetricValue.Annotation) object);
{
ExtensionType leftExtension;
leftExtension = this.getExtension();
ExtensionType rightExtension;
rightExtension = that.getExtension();
if (this.extension!= null) {
if (that.extension!= null) {
if (!leftExtension.equals(rightExtension)) {
return false;
}
} else {
return false;
}
} else {
if (that.extension!= null) {
return false;
}
}
}
{
CodedValue leftType;
leftType = this.getType();
CodedValue rightType;
rightType = that.getType();
if (this.type!= null) {
if (that.type!= null) {
if (!leftType.equals(rightType)) {
return false;
}
} else {
return false;
}
} else {
if (that.type!= null) {
return false;
}
}
}
return true;
}
@Override
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
ExtensionType theExtension;
theExtension = this.getExtension();
if (this.extension!= null) {
currentHashCode += theExtension.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
CodedValue theType;
theType = this.getType();
if (this.type!= null) {
currentHashCode += theType.hashCode();
}
}
return currentHashCode;
}
@Override
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
ExtensionType theExtension;
theExtension = this.getExtension();
strategy.appendField(locator, this, "extension", buffer, theExtension, (this.extension!= null));
}
{
CodedValue theType;
theType = this.getType();
strategy.appendField(locator, this, "type", buffer, theType, (this.type!= null));
}
return buffer;
}
@Override
public Object clone() {
return copyTo(createNewInstance());
}
@Override
public Object copyTo(Object target) {
final CopyStrategy strategy = JAXBCopyStrategy.getInstance();
return copyTo(null, target, strategy);
}
@Override
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof AbstractMetricValue.Annotation) {
final AbstractMetricValue.Annotation copy = ((AbstractMetricValue.Annotation) draftCopy);
{
Boolean extensionShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.extension!= null));
if (extensionShouldBeCopiedAndSet == Boolean.TRUE) {
ExtensionType sourceExtension;
sourceExtension = this.getExtension();
ExtensionType copyExtension = ((ExtensionType) strategy.copy(LocatorUtils.property(locator, "extension", sourceExtension), sourceExtension, (this.extension!= null)));
copy.setExtension(copyExtension);
} else {
if (extensionShouldBeCopiedAndSet == Boolean.FALSE) {
copy.extension = null;
}
}
}
{
Boolean typeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.type!= null));
if (typeShouldBeCopiedAndSet == Boolean.TRUE) {
CodedValue sourceType;
sourceType = this.getType();
CodedValue copyType = ((CodedValue) strategy.copy(LocatorUtils.property(locator, "type", sourceType), sourceType, (this.type!= null)));
copy.setType(copyType);
} else {
if (typeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.type = null;
}
}
}
}
return draftCopy;
}
@Override
public Object createNewInstance() {
return new AbstractMetricValue.Annotation();
}
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"extension"
})
public static class MetricQuality implements Cloneable, CopyTo, ToString
{
@XmlElement(name = "Extension", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/extension")
protected ExtensionType extension;
/**
* While Validity is "Ong" or "NA", the enclosing METRIC value SHALL not possess a determined value. See also pm:MeasurementValidity.
*
* NOTE—In case of other values the enclosing METRIC is allowed to possess a determined value.
*
*/
@XmlAttribute(name = "Validity", required = true)
protected MeasurementValidity validity;
/**
* Describes whether data is generated by a real METRIC source or is part of any test or demo data. The implied value SHALL be "Real".
*
*/
@XmlAttribute(name = "Mode")
protected GenerationMode mode;
/**
* See pm:QualityIndicator. The implied value SHALL be "1".
*
*/
@XmlAttribute(name = "Qi")
protected BigDecimal qi;
/**
* Gets the value of the extension property.
*
* @return
* possible object is
* {@link ExtensionType }
*
*/
@Nullable
public ExtensionType getExtension() {
return extension;
}
/**
* Sets the value of the extension property.
*
* @param value
* allowed object is
* {@link ExtensionType }
*
*/
public void setExtension(
@Nullable
ExtensionType value) {
this.extension = value;
}
/**
* While Validity is "Ong" or "NA", the enclosing METRIC value SHALL not possess a determined value. See also pm:MeasurementValidity.
*
* NOTE—In case of other values the enclosing METRIC is allowed to possess a determined value.
*
* @return
* possible object is
* {@link MeasurementValidity }
*
*/
public MeasurementValidity getValidity() {
return validity;
}
/**
* Sets the value of the validity property.
*
* @param value
* allowed object is
* {@link MeasurementValidity }
*
* @see #getValidity()
*/
public void setValidity(MeasurementValidity value) {
this.validity = value;
}
/**
* Describes whether data is generated by a real METRIC source or is part of any test or demo data. The implied value SHALL be "Real".
*
* @return
* possible object is
* {@link GenerationMode }
*
*/
@Nullable
public GenerationMode getMode() {
return mode;
}
/**
* Sets the value of the mode property.
*
* @param value
* allowed object is
* {@link GenerationMode }
*
* @see #getMode()
*/
public void setMode(
@Nullable
GenerationMode value) {
this.mode = value;
}
/**
* See pm:QualityIndicator. The implied value SHALL be "1".
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
@Nullable
public BigDecimal getQi() {
return qi;
}
/**
* Sets the value of the qi property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
* @see #getQi()
*/
public void setQi(
@Nullable
BigDecimal value) {
this.qi = value;
}
@Override
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final AbstractMetricValue.MetricQuality that = ((AbstractMetricValue.MetricQuality) object);
{
ExtensionType leftExtension;
leftExtension = this.getExtension();
ExtensionType rightExtension;
rightExtension = that.getExtension();
if (this.extension!= null) {
if (that.extension!= null) {
if (!leftExtension.equals(rightExtension)) {
return false;
}
} else {
return false;
}
} else {
if (that.extension!= null) {
return false;
}
}
}
{
MeasurementValidity leftValidity;
leftValidity = this.getValidity();
MeasurementValidity rightValidity;
rightValidity = that.getValidity();
if (this.validity!= null) {
if (that.validity!= null) {
if (!leftValidity.equals(rightValidity)) {
return false;
}
} else {
return false;
}
} else {
if (that.validity!= null) {
return false;
}
}
}
{
GenerationMode leftMode;
leftMode = this.getMode();
GenerationMode rightMode;
rightMode = that.getMode();
if (this.mode!= null) {
if (that.mode!= null) {
if (!leftMode.equals(rightMode)) {
return false;
}
} else {
return false;
}
} else {
if (that.mode!= null) {
return false;
}
}
}
{
BigDecimal leftQi;
leftQi = this.getQi();
BigDecimal rightQi;
rightQi = that.getQi();
if (this.qi!= null) {
if (that.qi!= null) {
if (!leftQi.equals(rightQi)) {
return false;
}
} else {
return false;
}
} else {
if (that.qi!= null) {
return false;
}
}
}
return true;
}
@Override
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
ExtensionType theExtension;
theExtension = this.getExtension();
if (this.extension!= null) {
currentHashCode += theExtension.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
MeasurementValidity theValidity;
theValidity = this.getValidity();
if (this.validity!= null) {
currentHashCode += theValidity.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
GenerationMode theMode;
theMode = this.getMode();
if (this.mode!= null) {
currentHashCode += theMode.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
BigDecimal theQi;
theQi = this.getQi();
if (this.qi!= null) {
currentHashCode += theQi.hashCode();
}
}
return currentHashCode;
}
@Override
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
ExtensionType theExtension;
theExtension = this.getExtension();
strategy.appendField(locator, this, "extension", buffer, theExtension, (this.extension!= null));
}
{
MeasurementValidity theValidity;
theValidity = this.getValidity();
strategy.appendField(locator, this, "validity", buffer, theValidity, (this.validity!= null));
}
{
GenerationMode theMode;
theMode = this.getMode();
strategy.appendField(locator, this, "mode", buffer, theMode, (this.mode!= null));
}
{
BigDecimal theQi;
theQi = this.getQi();
strategy.appendField(locator, this, "qi", buffer, theQi, (this.qi!= null));
}
return buffer;
}
@Override
public Object clone() {
return copyTo(createNewInstance());
}
@Override
public Object copyTo(Object target) {
final CopyStrategy strategy = JAXBCopyStrategy.getInstance();
return copyTo(null, target, strategy);
}
@Override
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof AbstractMetricValue.MetricQuality) {
final AbstractMetricValue.MetricQuality copy = ((AbstractMetricValue.MetricQuality) draftCopy);
{
Boolean extensionShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.extension!= null));
if (extensionShouldBeCopiedAndSet == Boolean.TRUE) {
ExtensionType sourceExtension;
sourceExtension = this.getExtension();
ExtensionType copyExtension = ((ExtensionType) strategy.copy(LocatorUtils.property(locator, "extension", sourceExtension), sourceExtension, (this.extension!= null)));
copy.setExtension(copyExtension);
} else {
if (extensionShouldBeCopiedAndSet == Boolean.FALSE) {
copy.extension = null;
}
}
}
{
Boolean validityShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.validity!= null));
if (validityShouldBeCopiedAndSet == Boolean.TRUE) {
MeasurementValidity sourceValidity;
sourceValidity = this.getValidity();
MeasurementValidity copyValidity = ((MeasurementValidity) strategy.copy(LocatorUtils.property(locator, "validity", sourceValidity), sourceValidity, (this.validity!= null)));
copy.setValidity(copyValidity);
} else {
if (validityShouldBeCopiedAndSet == Boolean.FALSE) {
copy.validity = null;
}
}
}
{
Boolean modeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.mode!= null));
if (modeShouldBeCopiedAndSet == Boolean.TRUE) {
GenerationMode sourceMode;
sourceMode = this.getMode();
GenerationMode copyMode = ((GenerationMode) strategy.copy(LocatorUtils.property(locator, "mode", sourceMode), sourceMode, (this.mode!= null)));
copy.setMode(copyMode);
} else {
if (modeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.mode = null;
}
}
}
{
Boolean qiShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.qi!= null));
if (qiShouldBeCopiedAndSet == Boolean.TRUE) {
BigDecimal sourceQi;
sourceQi = this.getQi();
BigDecimal copyQi = ((BigDecimal) strategy.copy(LocatorUtils.property(locator, "qi", sourceQi), sourceQi, (this.qi!= null)));
copy.setQi(copyQi);
} else {
if (qiShouldBeCopiedAndSet == Boolean.FALSE) {
copy.qi = null;
}
}
}
}
return draftCopy;
}
@Override
public Object createNewInstance() {
return new AbstractMetricValue.MetricQuality();
}
}
}