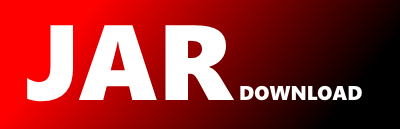
org.somda.sdc.biceps.model.participant.CalibrationInfo Maven / Gradle / Ivy
package org.somda.sdc.biceps.model.participant;
import java.time.Instant;
import java.util.ArrayList;
import java.util.List;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jetbrains.annotations.Nullable;
import org.jvnet.jaxb.lang.CopyStrategy;
import org.jvnet.jaxb.lang.CopyTo;
import org.jvnet.jaxb.lang.JAXBCopyStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
import org.somda.sdc.biceps.model.extension.ExtensionType;
import org.somda.sdc.common.model.TimestampAdapter;
/**
* Provides information in terms of component calibration. By default, it only maintains a calibration flag.
*
* Java class for CalibrationInfo complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CalibrationInfo", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/participant", propOrder = {
"extension",
"calibrationDocumentation"
})
public class CalibrationInfo implements Cloneable, CopyTo, ToString
{
@XmlElement(name = "Extension", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/extension")
protected ExtensionType extension;
/**
* CalibrationDocumentation provides information regarding necessary or performed calibration steps including potential calibration results like accuracy.
*
*/
@XmlElement(name = "CalibrationDocumentation", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/participant")
protected List calibrationDocumentation;
/**
* ATTRIBUTE definition of ComponentCalibration.
*
*/
@XmlAttribute(name = "ComponentCalibrationState")
protected CalibrationState componentCalibrationState;
/**
* Type of the calibration. The implied value SHALL be "Unspec".
*
*/
@XmlAttribute(name = "Type")
protected CalibrationType type;
/**
* Time of the calibration.
*
*/
@XmlAttribute(name = "Time")
@XmlJavaTypeAdapter(TimestampAdapter.class)
protected Instant time;
/**
* Gets the value of the extension property.
*
* @return
* possible object is
* {@link ExtensionType }
*
*/
@Nullable
public ExtensionType getExtension() {
return extension;
}
/**
* Sets the value of the extension property.
*
* @param value
* allowed object is
* {@link ExtensionType }
*
*/
public void setExtension(
@Nullable
ExtensionType value) {
this.extension = value;
}
/**
* CalibrationDocumentation provides information regarding necessary or performed calibration steps including potential calibration results like accuracy.
*
* Gets the value of the calibrationDocumentation property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the calibrationDocumentation property.
*
*
* For example, to add a new item, do as follows:
*
*
* getCalibrationDocumentation().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CalibrationInfo.CalibrationDocumentation }
*
*
*
* @return
* The value of the calibrationDocumentation property.
*/
public List getCalibrationDocumentation() {
if (calibrationDocumentation == null) {
calibrationDocumentation = new ArrayList<>();
}
return this.calibrationDocumentation;
}
/**
* ATTRIBUTE definition of ComponentCalibration.
*
* @return
* possible object is
* {@link CalibrationState }
*
*/
@Nullable
public CalibrationState getComponentCalibrationState() {
return componentCalibrationState;
}
/**
* Sets the value of the componentCalibrationState property.
*
* @param value
* allowed object is
* {@link CalibrationState }
*
* @see #getComponentCalibrationState()
*/
public void setComponentCalibrationState(
@Nullable
CalibrationState value) {
this.componentCalibrationState = value;
}
/**
* Type of the calibration. The implied value SHALL be "Unspec".
*
* @return
* possible object is
* {@link CalibrationType }
*
*/
@Nullable
public CalibrationType getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link CalibrationType }
*
* @see #getType()
*/
public void setType(
@Nullable
CalibrationType value) {
this.type = value;
}
/**
* Time of the calibration.
*
* @return
* possible object is
* {@link String }
*
*/
@Nullable
public Instant getTime() {
return time;
}
/**
* Sets the value of the time property.
*
* @param value
* allowed object is
* {@link String }
*
* @see #getTime()
*/
public void setTime(
@Nullable
Instant value) {
this.time = value;
}
public void setCalibrationDocumentation(
@Nullable
List value) {
this.calibrationDocumentation = null;
if (value!= null) {
List draftl = this.getCalibrationDocumentation();
draftl.addAll(value);
}
}
@Override
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final CalibrationInfo that = ((CalibrationInfo) object);
{
ExtensionType leftExtension;
leftExtension = this.getExtension();
ExtensionType rightExtension;
rightExtension = that.getExtension();
if (this.extension!= null) {
if (that.extension!= null) {
if (!leftExtension.equals(rightExtension)) {
return false;
}
} else {
return false;
}
} else {
if (that.extension!= null) {
return false;
}
}
}
{
List leftCalibrationDocumentation;
leftCalibrationDocumentation = (((this.calibrationDocumentation!= null)&&(!this.calibrationDocumentation.isEmpty()))?this.getCalibrationDocumentation():null);
List rightCalibrationDocumentation;
rightCalibrationDocumentation = (((that.calibrationDocumentation!= null)&&(!that.calibrationDocumentation.isEmpty()))?that.getCalibrationDocumentation():null);
if ((this.calibrationDocumentation!= null)&&(!this.calibrationDocumentation.isEmpty())) {
if ((that.calibrationDocumentation!= null)&&(!that.calibrationDocumentation.isEmpty())) {
if (!leftCalibrationDocumentation.equals(rightCalibrationDocumentation)) {
return false;
}
} else {
return false;
}
} else {
if ((that.calibrationDocumentation!= null)&&(!that.calibrationDocumentation.isEmpty())) {
return false;
}
}
}
{
CalibrationState leftComponentCalibrationState;
leftComponentCalibrationState = this.getComponentCalibrationState();
CalibrationState rightComponentCalibrationState;
rightComponentCalibrationState = that.getComponentCalibrationState();
if (this.componentCalibrationState!= null) {
if (that.componentCalibrationState!= null) {
if (!leftComponentCalibrationState.equals(rightComponentCalibrationState)) {
return false;
}
} else {
return false;
}
} else {
if (that.componentCalibrationState!= null) {
return false;
}
}
}
{
CalibrationType leftType;
leftType = this.getType();
CalibrationType rightType;
rightType = that.getType();
if (this.type!= null) {
if (that.type!= null) {
if (!leftType.equals(rightType)) {
return false;
}
} else {
return false;
}
} else {
if (that.type!= null) {
return false;
}
}
}
{
Instant leftTime;
leftTime = this.getTime();
Instant rightTime;
rightTime = that.getTime();
if (this.time!= null) {
if (that.time!= null) {
if (!leftTime.equals(rightTime)) {
return false;
}
} else {
return false;
}
} else {
if (that.time!= null) {
return false;
}
}
}
return true;
}
@Override
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
ExtensionType theExtension;
theExtension = this.getExtension();
if (this.extension!= null) {
currentHashCode += theExtension.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theCalibrationDocumentation;
theCalibrationDocumentation = (((this.calibrationDocumentation!= null)&&(!this.calibrationDocumentation.isEmpty()))?this.getCalibrationDocumentation():null);
if ((this.calibrationDocumentation!= null)&&(!this.calibrationDocumentation.isEmpty())) {
currentHashCode += theCalibrationDocumentation.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
CalibrationState theComponentCalibrationState;
theComponentCalibrationState = this.getComponentCalibrationState();
if (this.componentCalibrationState!= null) {
currentHashCode += theComponentCalibrationState.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
CalibrationType theType;
theType = this.getType();
if (this.type!= null) {
currentHashCode += theType.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Instant theTime;
theTime = this.getTime();
if (this.time!= null) {
currentHashCode += theTime.hashCode();
}
}
return currentHashCode;
}
@Override
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
ExtensionType theExtension;
theExtension = this.getExtension();
strategy.appendField(locator, this, "extension", buffer, theExtension, (this.extension!= null));
}
{
List theCalibrationDocumentation;
theCalibrationDocumentation = (((this.calibrationDocumentation!= null)&&(!this.calibrationDocumentation.isEmpty()))?this.getCalibrationDocumentation():null);
strategy.appendField(locator, this, "calibrationDocumentation", buffer, theCalibrationDocumentation, ((this.calibrationDocumentation!= null)&&(!this.calibrationDocumentation.isEmpty())));
}
{
CalibrationState theComponentCalibrationState;
theComponentCalibrationState = this.getComponentCalibrationState();
strategy.appendField(locator, this, "componentCalibrationState", buffer, theComponentCalibrationState, (this.componentCalibrationState!= null));
}
{
CalibrationType theType;
theType = this.getType();
strategy.appendField(locator, this, "type", buffer, theType, (this.type!= null));
}
{
Instant theTime;
theTime = this.getTime();
strategy.appendField(locator, this, "time", buffer, theTime, (this.time!= null));
}
return buffer;
}
@Override
public Object clone() {
return copyTo(createNewInstance());
}
@Override
public Object copyTo(Object target) {
final CopyStrategy strategy = JAXBCopyStrategy.getInstance();
return copyTo(null, target, strategy);
}
@Override
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof CalibrationInfo) {
final CalibrationInfo copy = ((CalibrationInfo) draftCopy);
{
Boolean extensionShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.extension!= null));
if (extensionShouldBeCopiedAndSet == Boolean.TRUE) {
ExtensionType sourceExtension;
sourceExtension = this.getExtension();
ExtensionType copyExtension = ((ExtensionType) strategy.copy(LocatorUtils.property(locator, "extension", sourceExtension), sourceExtension, (this.extension!= null)));
copy.setExtension(copyExtension);
} else {
if (extensionShouldBeCopiedAndSet == Boolean.FALSE) {
copy.extension = null;
}
}
}
{
Boolean calibrationDocumentationShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.calibrationDocumentation!= null)&&(!this.calibrationDocumentation.isEmpty())));
if (calibrationDocumentationShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceCalibrationDocumentation;
sourceCalibrationDocumentation = (((this.calibrationDocumentation!= null)&&(!this.calibrationDocumentation.isEmpty()))?this.getCalibrationDocumentation():null);
@SuppressWarnings("unchecked")
List copyCalibrationDocumentation = ((List ) strategy.copy(LocatorUtils.property(locator, "calibrationDocumentation", sourceCalibrationDocumentation), sourceCalibrationDocumentation, ((this.calibrationDocumentation!= null)&&(!this.calibrationDocumentation.isEmpty()))));
copy.setCalibrationDocumentation(copyCalibrationDocumentation);
} else {
if (calibrationDocumentationShouldBeCopiedAndSet == Boolean.FALSE) {
copy.calibrationDocumentation = null;
}
}
}
{
Boolean componentCalibrationStateShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.componentCalibrationState!= null));
if (componentCalibrationStateShouldBeCopiedAndSet == Boolean.TRUE) {
CalibrationState sourceComponentCalibrationState;
sourceComponentCalibrationState = this.getComponentCalibrationState();
CalibrationState copyComponentCalibrationState = ((CalibrationState) strategy.copy(LocatorUtils.property(locator, "componentCalibrationState", sourceComponentCalibrationState), sourceComponentCalibrationState, (this.componentCalibrationState!= null)));
copy.setComponentCalibrationState(copyComponentCalibrationState);
} else {
if (componentCalibrationStateShouldBeCopiedAndSet == Boolean.FALSE) {
copy.componentCalibrationState = null;
}
}
}
{
Boolean typeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.type!= null));
if (typeShouldBeCopiedAndSet == Boolean.TRUE) {
CalibrationType sourceType;
sourceType = this.getType();
CalibrationType copyType = ((CalibrationType) strategy.copy(LocatorUtils.property(locator, "type", sourceType), sourceType, (this.type!= null)));
copy.setType(copyType);
} else {
if (typeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.type = null;
}
}
}
{
Boolean timeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.time!= null));
if (timeShouldBeCopiedAndSet == Boolean.TRUE) {
Instant sourceTime;
sourceTime = this.getTime();
Instant copyTime = ((Instant) strategy.copy(LocatorUtils.property(locator, "time", sourceTime), sourceTime, (this.time!= null)));
copy.setTime(copyTime);
} else {
if (timeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.time = null;
}
}
}
}
return draftCopy;
}
@Override
public Object createNewInstance() {
return new CalibrationInfo();
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"documentation",
"calibrationResult"
})
public static class CalibrationDocumentation implements Cloneable, CopyTo, ToString
{
/**
* Human-readable documentation of a CalibrationDocumentation entry.
*
*/
@XmlElement(name = "Documentation", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/participant")
protected List documentation;
@XmlElement(name = "CalibrationResult", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/participant")
protected List calibrationResult;
/**
* Human-readable documentation of a CalibrationDocumentation entry.
*
* Gets the value of the documentation property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the documentation property.
*
*
* For example, to add a new item, do as follows:
*
*
* getDocumentation().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LocalizedText }
*
*
*
* @return
* The value of the documentation property.
*/
public List getDocumentation() {
if (documentation == null) {
documentation = new ArrayList<>();
}
return this.documentation;
}
/**
* Gets the value of the calibrationResult property.
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the calibrationResult property.
*
*
* For example, to add a new item, do as follows:
*
*
* getCalibrationResult().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CalibrationInfo.CalibrationDocumentation.CalibrationResult }
*
*
*
* @return
* The value of the calibrationResult property.
*/
public List getCalibrationResult() {
if (calibrationResult == null) {
calibrationResult = new ArrayList<>();
}
return this.calibrationResult;
}
public void setDocumentation(
@Nullable
List value) {
this.documentation = null;
if (value!= null) {
List draftl = this.getDocumentation();
draftl.addAll(value);
}
}
public void setCalibrationResult(
@Nullable
List value) {
this.calibrationResult = null;
if (value!= null) {
List draftl = this.getCalibrationResult();
draftl.addAll(value);
}
}
@Override
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final CalibrationInfo.CalibrationDocumentation that = ((CalibrationInfo.CalibrationDocumentation) object);
{
List leftDocumentation;
leftDocumentation = (((this.documentation!= null)&&(!this.documentation.isEmpty()))?this.getDocumentation():null);
List rightDocumentation;
rightDocumentation = (((that.documentation!= null)&&(!that.documentation.isEmpty()))?that.getDocumentation():null);
if ((this.documentation!= null)&&(!this.documentation.isEmpty())) {
if ((that.documentation!= null)&&(!that.documentation.isEmpty())) {
if (!leftDocumentation.equals(rightDocumentation)) {
return false;
}
} else {
return false;
}
} else {
if ((that.documentation!= null)&&(!that.documentation.isEmpty())) {
return false;
}
}
}
{
List leftCalibrationResult;
leftCalibrationResult = (((this.calibrationResult!= null)&&(!this.calibrationResult.isEmpty()))?this.getCalibrationResult():null);
List rightCalibrationResult;
rightCalibrationResult = (((that.calibrationResult!= null)&&(!that.calibrationResult.isEmpty()))?that.getCalibrationResult():null);
if ((this.calibrationResult!= null)&&(!this.calibrationResult.isEmpty())) {
if ((that.calibrationResult!= null)&&(!that.calibrationResult.isEmpty())) {
if (!leftCalibrationResult.equals(rightCalibrationResult)) {
return false;
}
} else {
return false;
}
} else {
if ((that.calibrationResult!= null)&&(!that.calibrationResult.isEmpty())) {
return false;
}
}
}
return true;
}
@Override
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
List theDocumentation;
theDocumentation = (((this.documentation!= null)&&(!this.documentation.isEmpty()))?this.getDocumentation():null);
if ((this.documentation!= null)&&(!this.documentation.isEmpty())) {
currentHashCode += theDocumentation.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
List theCalibrationResult;
theCalibrationResult = (((this.calibrationResult!= null)&&(!this.calibrationResult.isEmpty()))?this.getCalibrationResult():null);
if ((this.calibrationResult!= null)&&(!this.calibrationResult.isEmpty())) {
currentHashCode += theCalibrationResult.hashCode();
}
}
return currentHashCode;
}
@Override
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
List theDocumentation;
theDocumentation = (((this.documentation!= null)&&(!this.documentation.isEmpty()))?this.getDocumentation():null);
strategy.appendField(locator, this, "documentation", buffer, theDocumentation, ((this.documentation!= null)&&(!this.documentation.isEmpty())));
}
{
List theCalibrationResult;
theCalibrationResult = (((this.calibrationResult!= null)&&(!this.calibrationResult.isEmpty()))?this.getCalibrationResult():null);
strategy.appendField(locator, this, "calibrationResult", buffer, theCalibrationResult, ((this.calibrationResult!= null)&&(!this.calibrationResult.isEmpty())));
}
return buffer;
}
@Override
public Object clone() {
return copyTo(createNewInstance());
}
@Override
public Object copyTo(Object target) {
final CopyStrategy strategy = JAXBCopyStrategy.getInstance();
return copyTo(null, target, strategy);
}
@Override
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof CalibrationInfo.CalibrationDocumentation) {
final CalibrationInfo.CalibrationDocumentation copy = ((CalibrationInfo.CalibrationDocumentation) draftCopy);
{
Boolean documentationShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.documentation!= null)&&(!this.documentation.isEmpty())));
if (documentationShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceDocumentation;
sourceDocumentation = (((this.documentation!= null)&&(!this.documentation.isEmpty()))?this.getDocumentation():null);
@SuppressWarnings("unchecked")
List copyDocumentation = ((List ) strategy.copy(LocatorUtils.property(locator, "documentation", sourceDocumentation), sourceDocumentation, ((this.documentation!= null)&&(!this.documentation.isEmpty()))));
copy.setDocumentation(copyDocumentation);
} else {
if (documentationShouldBeCopiedAndSet == Boolean.FALSE) {
copy.documentation = null;
}
}
}
{
Boolean calibrationResultShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.calibrationResult!= null)&&(!this.calibrationResult.isEmpty())));
if (calibrationResultShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceCalibrationResult;
sourceCalibrationResult = (((this.calibrationResult!= null)&&(!this.calibrationResult.isEmpty()))?this.getCalibrationResult():null);
@SuppressWarnings("unchecked")
List copyCalibrationResult = ((List ) strategy.copy(LocatorUtils.property(locator, "calibrationResult", sourceCalibrationResult), sourceCalibrationResult, ((this.calibrationResult!= null)&&(!this.calibrationResult.isEmpty()))));
copy.setCalibrationResult(copyCalibrationResult);
} else {
if (calibrationResultShouldBeCopiedAndSet == Boolean.FALSE) {
copy.calibrationResult = null;
}
}
}
}
return draftCopy;
}
@Override
public Object createNewInstance() {
return new CalibrationInfo.CalibrationDocumentation();
}
/**
* Java class for anonymous complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"code",
"value"
})
public static class CalibrationResult implements Cloneable, CopyTo, ToString
{
/**
* Code that semantically describes the calibration result.
*
*/
@XmlElement(name = "Code", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/participant", required = true)
protected CodedValue code;
/**
* Measurement that represents the value related to that specific calibration result.
*
*/
@XmlElement(name = "Value", namespace = "http://standards.ieee.org/downloads/11073/11073-10207-2017/participant", required = true)
protected Measurement value;
/**
* Code that semantically describes the calibration result.
*
* @return
* possible object is
* {@link CodedValue }
*
*/
public CodedValue getCode() {
return code;
}
/**
* Sets the value of the code property.
*
* @param value
* allowed object is
* {@link CodedValue }
*
* @see #getCode()
*/
public void setCode(CodedValue value) {
this.code = value;
}
/**
* Measurement that represents the value related to that specific calibration result.
*
* @return
* possible object is
* {@link Measurement }
*
*/
public Measurement getValue() {
return value;
}
/**
* Sets the value of the value property.
*
* @param value
* allowed object is
* {@link Measurement }
*
* @see #getValue()
*/
public void setValue(Measurement value) {
this.value = value;
}
@Override
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final CalibrationInfo.CalibrationDocumentation.CalibrationResult that = ((CalibrationInfo.CalibrationDocumentation.CalibrationResult) object);
{
CodedValue leftCode;
leftCode = this.getCode();
CodedValue rightCode;
rightCode = that.getCode();
if (this.code!= null) {
if (that.code!= null) {
if (!leftCode.equals(rightCode)) {
return false;
}
} else {
return false;
}
} else {
if (that.code!= null) {
return false;
}
}
}
{
Measurement leftValue;
leftValue = this.getValue();
Measurement rightValue;
rightValue = that.getValue();
if (this.value!= null) {
if (that.value!= null) {
if (!leftValue.equals(rightValue)) {
return false;
}
} else {
return false;
}
} else {
if (that.value!= null) {
return false;
}
}
}
return true;
}
@Override
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
CodedValue theCode;
theCode = this.getCode();
if (this.code!= null) {
currentHashCode += theCode.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Measurement theValue;
theValue = this.getValue();
if (this.value!= null) {
currentHashCode += theValue.hashCode();
}
}
return currentHashCode;
}
@Override
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
CodedValue theCode;
theCode = this.getCode();
strategy.appendField(locator, this, "code", buffer, theCode, (this.code!= null));
}
{
Measurement theValue;
theValue = this.getValue();
strategy.appendField(locator, this, "value", buffer, theValue, (this.value!= null));
}
return buffer;
}
@Override
public Object clone() {
return copyTo(createNewInstance());
}
@Override
public Object copyTo(Object target) {
final CopyStrategy strategy = JAXBCopyStrategy.getInstance();
return copyTo(null, target, strategy);
}
@Override
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof CalibrationInfo.CalibrationDocumentation.CalibrationResult) {
final CalibrationInfo.CalibrationDocumentation.CalibrationResult copy = ((CalibrationInfo.CalibrationDocumentation.CalibrationResult) draftCopy);
{
Boolean codeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.code!= null));
if (codeShouldBeCopiedAndSet == Boolean.TRUE) {
CodedValue sourceCode;
sourceCode = this.getCode();
CodedValue copyCode = ((CodedValue) strategy.copy(LocatorUtils.property(locator, "code", sourceCode), sourceCode, (this.code!= null)));
copy.setCode(copyCode);
} else {
if (codeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.code = null;
}
}
}
{
Boolean valueShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.value!= null));
if (valueShouldBeCopiedAndSet == Boolean.TRUE) {
Measurement sourceValue;
sourceValue = this.getValue();
Measurement copyValue = ((Measurement) strategy.copy(LocatorUtils.property(locator, "value", sourceValue), sourceValue, (this.value!= null)));
copy.setValue(copyValue);
} else {
if (valueShouldBeCopiedAndSet == Boolean.FALSE) {
copy.value = null;
}
}
}
}
return draftCopy;
}
@Override
public Object createNewInstance() {
return new CalibrationInfo.CalibrationDocumentation.CalibrationResult();
}
}
}
}