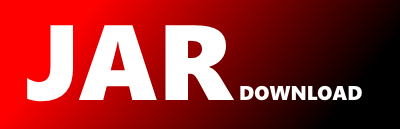
org.somda.sdc.dpws.model.ObjectFactory Maven / Gradle / Ivy
package org.somda.sdc.dpws.model;
import java.util.List;
import javax.xml.namespace.QName;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.annotation.XmlElementDecl;
import jakarta.xml.bind.annotation.XmlRegistry;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the org.somda.sdc.dpws.model package.
* An ObjectFactory allows you to programmatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private static final QName _ThisModel_QNAME = new QName("http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", "ThisModel");
private static final QName _ThisDevice_QNAME = new QName("http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", "ThisDevice");
private static final QName _Host_QNAME = new QName("http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", "Host");
private static final QName _Hosted_QNAME = new QName("http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", "Hosted");
private static final QName _ServiceId_QNAME = new QName("http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", "ServiceId");
private static final QName _Types_QNAME = new QName("http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", "Types");
private static final QName _Profile_QNAME = new QName("http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", "Profile");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: org.somda.sdc.dpws.model
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link ThisModelType }
*
* @return
* the new instance of {@link ThisModelType }
*/
public ThisModelType createThisModelType() {
return new ThisModelType();
}
/**
* Create an instance of {@link ThisDeviceType }
*
* @return
* the new instance of {@link ThisDeviceType }
*/
public ThisDeviceType createThisDeviceType() {
return new ThisDeviceType();
}
/**
* Create an instance of {@link Relationship }
*
* @return
* the new instance of {@link Relationship }
*/
public Relationship createRelationship() {
return new Relationship();
}
/**
* Create an instance of {@link HostServiceType }
*
* @return
* the new instance of {@link HostServiceType }
*/
public HostServiceType createHostServiceType() {
return new HostServiceType();
}
/**
* Create an instance of {@link HostedServiceType }
*
* @return
* the new instance of {@link HostedServiceType }
*/
public HostedServiceType createHostedServiceType() {
return new HostedServiceType();
}
/**
* Create an instance of {@link AssertionType }
*
* @return
* the new instance of {@link AssertionType }
*/
public AssertionType createAssertionType() {
return new AssertionType();
}
/**
* Create an instance of {@link LocalizedStringType }
*
* @return
* the new instance of {@link LocalizedStringType }
*/
public LocalizedStringType createLocalizedStringType() {
return new LocalizedStringType();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ThisModelType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ThisModelType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", name = "ThisModel")
public JAXBElement createThisModel(ThisModelType value) {
return new JAXBElement<>(_ThisModel_QNAME, ThisModelType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ThisDeviceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ThisDeviceType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", name = "ThisDevice")
public JAXBElement createThisDevice(ThisDeviceType value) {
return new JAXBElement<>(_ThisDevice_QNAME, ThisDeviceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link HostServiceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link HostServiceType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", name = "Host")
public JAXBElement createHost(HostServiceType value) {
return new JAXBElement<>(_Host_QNAME, HostServiceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link HostedServiceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link HostedServiceType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", name = "Hosted")
public JAXBElement createHosted(HostedServiceType value) {
return new JAXBElement<>(_Hosted_QNAME, HostedServiceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", name = "ServiceId")
public JAXBElement createServiceId(String value) {
return new JAXBElement<>(_ServiceId_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link List }{@code <}{@link QName }{@code >}{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link List }{@code <}{@link QName }{@code >}{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", name = "Types")
public JAXBElement> createTypes(List value) {
return new JAXBElement<>(_Types_QNAME, ((Class) List.class), null, ((List ) value));
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AssertionType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link AssertionType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/ws-dd/ns/dpws/2009/01", name = "Profile")
public JAXBElement createProfile(AssertionType value) {
return new JAXBElement<>(_Profile_QNAME, AssertionType.class, null, value);
}
}