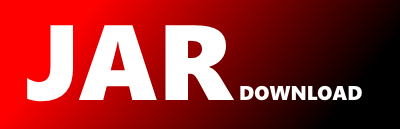
org.somda.sdc.dpws.soap.model.Fault Maven / Gradle / Ivy
package org.somda.sdc.dpws.soap.model;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import org.jvnet.jaxb.lang.CopyStrategy;
import org.jvnet.jaxb.lang.CopyTo;
import org.jvnet.jaxb.lang.JAXBCopyStrategy;
import org.jvnet.jaxb.lang.JAXBToStringStrategy;
import org.jvnet.jaxb.lang.ToString;
import org.jvnet.jaxb.lang.ToStringStrategy;
import org.jvnet.jaxb.locator.ObjectLocator;
import org.jvnet.jaxb.locator.util.LocatorUtils;
/**
* Fault reporting structure
*
* Java class for Fault complex type
.
*
* The following schema fragment specifies the expected content contained within this class.
*
* {@code
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Fault", namespace = "http://www.w3.org/2003/05/soap-envelope", propOrder = {
"code",
"reason",
"node",
"role",
"detail"
})
public class Fault implements Cloneable, CopyTo, ToString
{
@XmlElement(name = "Code", namespace = "http://www.w3.org/2003/05/soap-envelope", required = true)
protected Faultcode code;
@XmlElement(name = "Reason", namespace = "http://www.w3.org/2003/05/soap-envelope", required = true)
protected Faultreason reason;
@XmlElement(name = "Node", namespace = "http://www.w3.org/2003/05/soap-envelope")
@XmlSchemaType(name = "anyURI")
protected String node;
@XmlElement(name = "Role", namespace = "http://www.w3.org/2003/05/soap-envelope")
@XmlSchemaType(name = "anyURI")
protected String role;
@XmlElement(name = "Detail", namespace = "http://www.w3.org/2003/05/soap-envelope")
protected Detail detail;
/**
* Gets the value of the code property.
*
* @return
* possible object is
* {@link Faultcode }
*
*/
public Faultcode getCode() {
return code;
}
/**
* Sets the value of the code property.
*
* @param value
* allowed object is
* {@link Faultcode }
*
*/
public void setCode(Faultcode value) {
this.code = value;
}
/**
* Gets the value of the reason property.
*
* @return
* possible object is
* {@link Faultreason }
*
*/
public Faultreason getReason() {
return reason;
}
/**
* Sets the value of the reason property.
*
* @param value
* allowed object is
* {@link Faultreason }
*
*/
public void setReason(Faultreason value) {
this.reason = value;
}
/**
* Gets the value of the node property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNode() {
return node;
}
/**
* Sets the value of the node property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNode(String value) {
this.node = value;
}
/**
* Gets the value of the role property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRole() {
return role;
}
/**
* Sets the value of the role property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRole(String value) {
this.role = value;
}
/**
* Gets the value of the detail property.
*
* @return
* possible object is
* {@link Detail }
*
*/
public Detail getDetail() {
return detail;
}
/**
* Sets the value of the detail property.
*
* @param value
* allowed object is
* {@link Detail }
*
*/
public void setDetail(Detail value) {
this.detail = value;
}
@Override
public boolean equals(Object object) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Fault that = ((Fault) object);
{
Faultcode leftCode;
leftCode = this.getCode();
Faultcode rightCode;
rightCode = that.getCode();
if (this.code!= null) {
if (that.code!= null) {
if (!leftCode.equals(rightCode)) {
return false;
}
} else {
return false;
}
} else {
if (that.code!= null) {
return false;
}
}
}
{
Faultreason leftReason;
leftReason = this.getReason();
Faultreason rightReason;
rightReason = that.getReason();
if (this.reason!= null) {
if (that.reason!= null) {
if (!leftReason.equals(rightReason)) {
return false;
}
} else {
return false;
}
} else {
if (that.reason!= null) {
return false;
}
}
}
{
String leftNode;
leftNode = this.getNode();
String rightNode;
rightNode = that.getNode();
if (this.node!= null) {
if (that.node!= null) {
if (!leftNode.equals(rightNode)) {
return false;
}
} else {
return false;
}
} else {
if (that.node!= null) {
return false;
}
}
}
{
String leftRole;
leftRole = this.getRole();
String rightRole;
rightRole = that.getRole();
if (this.role!= null) {
if (that.role!= null) {
if (!leftRole.equals(rightRole)) {
return false;
}
} else {
return false;
}
} else {
if (that.role!= null) {
return false;
}
}
}
{
Detail leftDetail;
leftDetail = this.getDetail();
Detail rightDetail;
rightDetail = that.getDetail();
if (this.detail!= null) {
if (that.detail!= null) {
if (!leftDetail.equals(rightDetail)) {
return false;
}
} else {
return false;
}
} else {
if (that.detail!= null) {
return false;
}
}
}
return true;
}
@Override
public int hashCode() {
int currentHashCode = 1;
{
currentHashCode = (currentHashCode* 31);
Faultcode theCode;
theCode = this.getCode();
if (this.code!= null) {
currentHashCode += theCode.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Faultreason theReason;
theReason = this.getReason();
if (this.reason!= null) {
currentHashCode += theReason.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theNode;
theNode = this.getNode();
if (this.node!= null) {
currentHashCode += theNode.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
String theRole;
theRole = this.getRole();
if (this.role!= null) {
currentHashCode += theRole.hashCode();
}
}
{
currentHashCode = (currentHashCode* 31);
Detail theDetail;
theDetail = this.getDetail();
if (this.detail!= null) {
currentHashCode += theDetail.hashCode();
}
}
return currentHashCode;
}
@Override
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
Faultcode theCode;
theCode = this.getCode();
strategy.appendField(locator, this, "code", buffer, theCode, (this.code!= null));
}
{
Faultreason theReason;
theReason = this.getReason();
strategy.appendField(locator, this, "reason", buffer, theReason, (this.reason!= null));
}
{
String theNode;
theNode = this.getNode();
strategy.appendField(locator, this, "node", buffer, theNode, (this.node!= null));
}
{
String theRole;
theRole = this.getRole();
strategy.appendField(locator, this, "role", buffer, theRole, (this.role!= null));
}
{
Detail theDetail;
theDetail = this.getDetail();
strategy.appendField(locator, this, "detail", buffer, theDetail, (this.detail!= null));
}
return buffer;
}
@Override
public Object clone() {
return copyTo(createNewInstance());
}
@Override
public Object copyTo(Object target) {
final CopyStrategy strategy = JAXBCopyStrategy.getInstance();
return copyTo(null, target, strategy);
}
@Override
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof Fault) {
final Fault copy = ((Fault) draftCopy);
{
Boolean codeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.code!= null));
if (codeShouldBeCopiedAndSet == Boolean.TRUE) {
Faultcode sourceCode;
sourceCode = this.getCode();
Faultcode copyCode = ((Faultcode) strategy.copy(LocatorUtils.property(locator, "code", sourceCode), sourceCode, (this.code!= null)));
copy.setCode(copyCode);
} else {
if (codeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.code = null;
}
}
}
{
Boolean reasonShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.reason!= null));
if (reasonShouldBeCopiedAndSet == Boolean.TRUE) {
Faultreason sourceReason;
sourceReason = this.getReason();
Faultreason copyReason = ((Faultreason) strategy.copy(LocatorUtils.property(locator, "reason", sourceReason), sourceReason, (this.reason!= null)));
copy.setReason(copyReason);
} else {
if (reasonShouldBeCopiedAndSet == Boolean.FALSE) {
copy.reason = null;
}
}
}
{
Boolean nodeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.node!= null));
if (nodeShouldBeCopiedAndSet == Boolean.TRUE) {
String sourceNode;
sourceNode = this.getNode();
String copyNode = ((String) strategy.copy(LocatorUtils.property(locator, "node", sourceNode), sourceNode, (this.node!= null)));
copy.setNode(copyNode);
} else {
if (nodeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.node = null;
}
}
}
{
Boolean roleShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.role!= null));
if (roleShouldBeCopiedAndSet == Boolean.TRUE) {
String sourceRole;
sourceRole = this.getRole();
String copyRole = ((String) strategy.copy(LocatorUtils.property(locator, "role", sourceRole), sourceRole, (this.role!= null)));
copy.setRole(copyRole);
} else {
if (roleShouldBeCopiedAndSet == Boolean.FALSE) {
copy.role = null;
}
}
}
{
Boolean detailShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.detail!= null));
if (detailShouldBeCopiedAndSet == Boolean.TRUE) {
Detail sourceDetail;
sourceDetail = this.getDetail();
Detail copyDetail = ((Detail) strategy.copy(LocatorUtils.property(locator, "detail", sourceDetail), sourceDetail, (this.detail!= null)));
copy.setDetail(copyDetail);
} else {
if (detailShouldBeCopiedAndSet == Boolean.FALSE) {
copy.detail = null;
}
}
}
}
return draftCopy;
}
@Override
public Object createNewInstance() {
return new Fault();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy