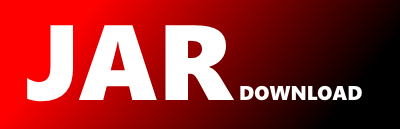
org.sonar.plugins.csharp.S2674.html Maven / Gradle / Ivy
Why is this an issue?
You cannot assume that any given stream reading call will fill the byte[]
passed in to the method with the number of bytes requested.
Instead, you must check the value returned by the read method to see how many bytes were read. Fail to do so, and you introduce a bug that is both
harmful and difficult to reproduce.
This rule raises an issue when a Stream.Read
or a Stream.ReadAsync
method is called, but the return value is not
checked.
Noncompliant code example
public void DoSomething(string fileName)
{
using (var stream = File.Open(fileName, FileMode.Open))
{
var result = new byte[stream.Length];
stream.Read(result, 0, (int)stream.Length); // Noncompliant
// ... do something with result
}
}
Compliant solution
public void DoSomething(string fileName)
{
using (var stream = File.Open(fileName, FileMode.Open))
{
var buffer = new byte[1024];
using (var ms = new MemoryStream())
{
int read;
while ((read = stream.Read(buffer, 0, buffer.Length)) > 0)
{
ms.Write(buffer, 0, read);
}
// ... do something with ms
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy