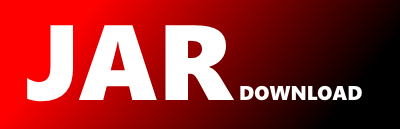
org.sonar.plugins.csharp.S5542.html Maven / Gradle / Ivy
This vulnerability exposes encrypted data to a number of attacks whose goal is to recover the plaintext.
Why is this an issue?
Encryption algorithms are essential for protecting sensitive information and ensuring secure communications in a variety of domains. They are used
for several important reasons:
- Confidentiality, privacy, and intellectual property protection
- Security during transmission or on storage devices
- Data integrity, general trust, and authentication
When selecting encryption algorithms, tools, or combinations, you should also consider two things:
- No encryption is unbreakable.
- The strength of an encryption algorithm is usually measured by the effort required to crack it within a reasonable time frame.
For these reasons, as soon as cryptography is included in a project, it is important to choose encryption algorithms that are considered strong and
secure by the cryptography community.
For AES, the weakest mode is ECB (Electronic Codebook). Repeated blocks of data are encrypted to the same value, making them easy to identify and
reducing the difficulty of recovering the original cleartext.
Unauthenticated modes such as CBC (Cipher Block Chaining) may be used but are prone to attacks that manipulate the ciphertext. They must be used
with caution.
For RSA, the weakest algorithms are either using it without padding or using the PKCS1v1.5 padding scheme.
What is the potential impact?
The cleartext of an encrypted message might be recoverable. Additionally, it might be possible to modify the cleartext of an encrypted message.
Below are some real-world scenarios that illustrate possible impacts of an attacker exploiting the vulnerability.
Theft of sensitive data
The encrypted message might contain data that is considered sensitive and should not be known to third parties.
By using a weak algorithm the likelihood that an attacker might be able to recover the cleartext drastically increases.
Additional attack surface
By modifying the cleartext of the encrypted message it might be possible for an attacker to trigger other vulnerabilities in the code. Encrypted
values are often considered trusted, since under normal circumstances it would not be possible for a third party to modify them.
How to fix it in .NET
Code examples
Noncompliant code example
Example with a symmetric cipher, AES:
using System.Security.Cryptography;
public void encrypt()
{
AesManaged aes = new AesManaged
{
keysize = 128,
blocksize = 128,
mode = ciphermode.ecb, // Noncompliant
padding = paddingmode.pkcs7
};
}
Note that Microsoft has marked derived cryptographic types like AesManaged
as no longer recommended for use.
Example with an asymmetric cipher, RSA:
using System.Security.Cryptography;
public void encrypt()
{
RSACryptoServiceProvider RsaCsp = new RSACryptoServiceProvider();
byte[] encryptedData = RsaCsp.Encrypt(dataToEncrypt, false); // Noncompliant
}
Compliant solution
For the AES symmetric cipher, use the GCM mode:
using System.Security.Cryptography;
public void encrypt()
{
AesGcm aes = AesGcm(key);
}
For the RSA asymmetric cipher, use the Optimal Asymmetric Encryption Padding (OAEP):
using System.Security.Cryptography;
public void encrypt()
{
RSACryptoServiceProvider RsaCsp = new RSACryptoServiceProvider();
byte[] encryptedData = RsaCsp.Encrypt(dataToEncrypt, true);
}
How does this work?
As a rule of thumb, use the cryptographic algorithms and mechanisms that are considered strong by the cryptographic community.
Appropriate choices are currently the following.
For AES: use authenticated encryption modes
The best-known authenticated encryption mode for AES is Galois/Counter mode (GCM).
GCM mode combines encryption with authentication and integrity checks using a cryptographic hash function and provides both confidentiality and
authenticity of data.
Other similar modes are:
- CCM:
Counter with CBC-MAC
- CWC:
Cipher Block Chaining with Message Authentication Code
- EAX:
Encrypt-and-Authenticate
- IAPM:
Integer Authenticated Parallelizable Mode
- OCB:
Offset Codebook Mode
It is also possible to use AES-CBC with HMAC for integrity checks. However, it is considered more straightforward to use AES-GCM directly
instead.
For RSA: use the OAEP scheme
The Optimal Asymmetric Encryption Padding scheme (OAEP) adds randomness and a secure hash function that strengthens the regular inner workings of
RSA.
Resources
Articles & blog posts
- Microsoft, Timing vulnerabilities with CBC-mode
symmetric decryption using padding
- Wikipedia, Padding Oracle Attack
- Wikipedia, Chosen-Ciphertext Attack
- Wikipedia, Chosen-Plaintext Attack
- Wikipedia, Semantically Secure Cryptosystems
- Wikipedia, OAEP
- Wikipedia, Galois/Counter Mode
Standards