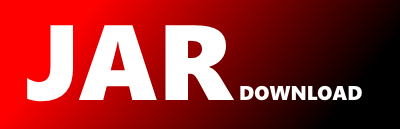
org.sonar.plugins.csharp.S5693.html Maven / Gradle / Ivy
Rejecting requests with significant content length is a good practice to control the network traffic intensity and thus resource consumption in
order to prevent DoS attacks.
Ask Yourself Whether
- size limits are not defined for the different resources of the web application.
- the web application is not protected by rate limiting features.
- the web application infrastructure has limited resources.
There is a risk if you answered yes to any of those questions.
Recommended Secure Coding Practices
- For most of the features of an application, it is recommended to limit the size of requests to:
- lower or equal to 8mb for file uploads.
- lower or equal to 2mb for other requests.
It is recommended to customize the rule with the limit values that correspond to the web application.
Sensitive Code Example
using Microsoft.AspNetCore.Mvc;
public class MyController : Controller
{
[HttpPost]
[DisableRequestSizeLimit] // Sensitive: No size limit
[RequestSizeLimit(10485760)] // Sensitive: 10485760 B = 10240 KB = 10 MB is more than the recommended limit of 8MB
public IActionResult PostRequest(Model model)
{
// ...
}
[HttpPost]
[RequestFormLimits(MultipartBodyLengthLimit = 10485760)] // Sensitive: 10485760 B = 10240 KB = 10 MB is more than the recommended limit of 8MB
public IActionResult MultipartFormRequest(Model model)
{
// ...
}
}
In Web.config:
<configuration>
<system.web>
<httpRuntime maxRequestLength="81920" executionTimeout="3600" />
<!-- Sensitive: maxRequestLength is expressed in KB, so 81920 KB = 80 MB -->
</system.web>
<system.webServer>
<security>
<requestFiltering>
<requestLimits maxAllowedContentLength="83886080" />
<!-- Sensitive: maxAllowedContentLength is expressed in bytes, so 83886080 B = 81920 KB = 80 MB -->
</requestFiltering>
</security>
</system.webServer>
</configuration>
Compliant Solution
using Microsoft.AspNetCore.Mvc;
public class MyController : Controller
{
[HttpPost]
[RequestSizeLimit(8388608)] // Compliant: 8388608 B = 8192 KB = 8 MB
public IActionResult PostRequest(Model model)
{
// ...
}
[HttpPost]
[RequestFormLimits(MultipartBodyLengthLimit = 8388608)] // Compliant: 8388608 B = 8192 KB = 8 MB
public IActionResult MultipartFormRequest(Model model)
{
// ...
}
}
In Web.config:
<configuration>
<system.web>
<httpRuntime maxRequestLength="8192" executionTimeout="3600" />
<!-- Compliant: maxRequestLength is expressed in KB, so 8192 KB = 8 MB -->
</system.web>
<system.webServer>
<security>
<requestFiltering>
<requestLimits maxAllowedContentLength="8388608" />
<!-- Compliant: maxAllowedContentLength is expressed in bytes, so 8388608 B = 8192 KB = 8 MB -->
</requestFiltering>
</security>
</system.webServer>
</configuration>
See
- OWASP - Top 10 2021 Category A5 - Security Misconfiguration
- Owasp Cheat Sheet - Owasp Denial of Service
Cheat Sheet
- OWASP - Top 10 2017 Category A6 - Security
Misconfiguration
- CWE - CWE-770 - Allocation of Resources Without Limits or Throttling
- CWE - CWE-400 - Uncontrolled Resource Consumption
- Web.config - XML-formatted config file for IIS
applications
© 2015 - 2024 Weber Informatics LLC | Privacy Policy