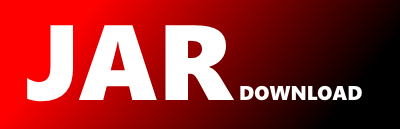
org.sonar.plugins.csharp.S6377.html Maven / Gradle / Ivy
XML signatures are a method used to ensure the integrity and authenticity of XML documents. However, if XML signatures are not validated securely,
it can lead to potential vulnerabilities.
Why is this an issue?
XML can be used for a wide variety of purposes. Using a signature on an XML message generally indicates this message requires authenticity and
integrity. However, if the signature validation is not properly implemented this authenticity can not be guaranteed.
What is the potential impact?
By not enforcing secure validation, the XML Digital Signature API is more susceptible to attacks such as signature spoofing and injections.
Increased Vulnerability to Signature Spoofing
By disabling secure validation, the application becomes more susceptible to signature spoofing attacks. Attackers can potentially manipulate the
XML signature in a way that bypasses the validation process, allowing them to forge or tamper with the signature. This can lead to the acceptance of
invalid or maliciously modified signatures, compromising the integrity and authenticity of the XML documents.
Risk of Injection Attacks
Disabling secure validation can expose the application to injection attacks. Attackers can inject malicious code or entities into the XML document,
taking advantage of the weakened validation process. In some cases, it can also expose the application to denial-of-service attacks. Attackers can
exploit vulnerabilities in the validation process to cause excessive resource consumption or system crashes, leading to service unavailability or
disruption.
How to fix it in ASP.NET Core
Code examples
The following noncompliant code example verifies an XML signature without providing a trusted public key. This code will validate the signature
against the embedded public key, accepting any forged signature.
Noncompliant code example
XmlDocument xmlDoc = new()
{
PreserveWhitespace = true
};
xmlDoc.Load("/data/login.xml");
SignedXml signedXml = new(xmlDoc);
XmlNodeList nodeList = xmlDoc.GetElementsByTagName("Signature");
signedXml.LoadXml((XmlElement?)nodeList[0]);
if (signedXml.CheckSignature()) {
// Process the XML content
} else {
// Raise an error
}
Compliant solution
CspParameters cspParams = new()
{
KeyContainerName = "MY_RSA_KEY"
};
RSACryptoServiceProvider rsaKey = new(cspParams);
XmlDocument xmlDoc = new()
{
PreserveWhitespace = true
};
xmlDoc.Load("/data/login.xml");
SignedXml signedXml = new(xmlDoc);
XmlNodeList nodeList = xmlDoc.GetElementsByTagName("Signature");
signedXml.LoadXml((XmlElement?)nodeList[0]);
if (signedXml.CheckSignature(rsaKey)) {
// Process the XML content
} else {
// Raise an error
}
How does this work?
Here, the compliant solution provides an RSA public key to the signature validation function. This will ensure only signatures computed with the
associated private key will be accepted. This prevents signature forgery attacks.
Resources
Documentation
- Microsoft Learn - System.Security.Cryptography.Xml
Namespace
- Microsfot Learn - How to: Verify the Digital
Signatures of XML Documents
Standards
- OWASP - Top 10:2021 A02:2021 - Cryptographic Failures
- OWASP - Top 10 2017 Category A3 - Sensitive Data
Exposure
- CWE - CWE-347 - Improper Verification of Cryptographic Signature
- STIG Viewer - Application Security and
Development: V-222608 - The application must not be vulnerable to XML-oriented attacks.