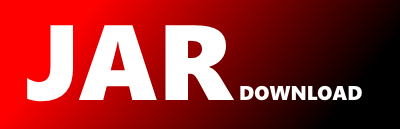
org.sonar.plugins.csharp.S6964.html Maven / Gradle / Ivy
"Under-posting"
refers to a situation where a client sends less data than expected to the server during an HTTP request, for example when the client omits some
properties from the request body that the server expects to receive.
Why is this an issue?
One of the main issues that under-posting can cause is data inconsistency. If the client sends less data than expected, the application might fill
any value type properties with their default values, leading to inaccurate or inconsistent data in your database. Additionally, there might be
unexpected behavior if there are certain data expected that are not provided and even security issues; for example, if a user omits a role or
permission field from a POST request, and the server fills in a default value, it could inadvertently grant more access than intended.
A model class (in this case the
Product
class) can be an input of an HTTP handler method:
public class ProductsController : Controller
{
[HttpPost]
public IActionResult Create([FromBody]Product product)
{
// Process product data...
}
}
Exceptions
This rule does not raise an issue when properties are decorated with the following attributes:
- ValidateNever
- JsonProperty(Required = Required.Always)
- JsonProperty(Required = Required.AllowNull)
- Newtonsoft.Json.JsonIgnore
- Newtonsoft.Json.JsonRequired
- System.Text.Json.Serialization.JsonRequired
- System.Text.Json.Serialization.JsonIgnore
- Range
- BindNever
Additionally, this rule does not raise for properties in model classes that are not in the same project as the Controller class that references
them. This is due to a limitation of Roslyn (see here).
How to fix it
You should mark any model value-type property as nullable, required or JsonRequired. Thus when a client
underposts, you ensure that the missing properties can be detected on the server side rather than being auto-filled, and therefore, incoming data
meets the application’s expectations.
Code examples
Noncompliant code example
public class Product
{
public int Id { get; set; } // Noncompliant
public string Name { get; set; }
public int NumberOfItems { get; set; } // Noncompliant
public decimal Price { get; set; } // Noncompliant
}
If the client sends a request without setting the NumberOfItems
or Price
properties, they will default to 0
.
In the request handler method, there’s no way to determine whether they were intentionally set to 0
or omitted by mistake.
Compliant solution
public class Product
{
public required int Id { get; set; }
public string Name { get; set; }
public int? NumberOfItems { get; set; } // Compliant - property is optional
[JsonRequired] public decimal Price { get; set; } // Compliant - property must have a value
}
In this example, the request handler method can
- manually check whether
NumberOfItems
was filled out through the HasValue
property
- rely on Model Validation to make sure
Price
is not missing
public class ProductsController : Controller
{
[HttpPost]
public IActionResult Create(Product product)
{
if (!ModelState.IsValid) // if product.Price is missing then the model state will not be valid
{
return View(product);
}
if (product.NumberOfItems.HasValue)
{
// ...
}
// Process input...
}
}
Recommended Secure Coding Practices
- Client-Side Validation: While server-side validation is crucial, implementing client-side validation can provide immediate feedback to the user
when certain fields are not filled out, which helps to avoid under-posting.
- Comprehensive Testing: Include testing scenarios that specifically check for under-posting vulnerabilities to ensure that all required data is
correctly validated and processed.
Resources
Documentation
- Microsoft Learn - Overview of ASP.NET Core MVC
- Microsoft Learn - Overview of
ASP.NET MVC 5
- Microsoft Learn - Overview of ASP.NET Razor Pages
- Microsoft Learn - Model Classes in ASP.NET MVC
- Microsoft Learn - Model Binding in ASP.NET Core
- Microsoft Learn - Model Validation in
ASP.NET Web API
- Microsoft Learn - Nullable
Value Types in .NET
- Microsoft Learn - Data
Annotations
- Microsoft Learn - RequiredAttribute
Class
- Microsoft Learn - ValidateNeverAttribute
Class