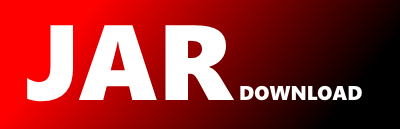
org.sonar.plugins.csharp.S2222.html Maven / Gradle / Ivy
Why is this an issue?
To prevent potential deadlocks in an application, it is crucial to release any locks that are
acquired within a method along all possible execution paths.
Failing to release locks properly can lead to potential deadlocks, where the lock might not be released, causing issues in the application.
This rule specifically focuses on tracking the following types from the System.Threading
namespace:
An issue is reported when a lock is acquired within a method but not released on all paths.
Exceptions
If the lock is never released within the method, no issue is raised, assuming that the callers will handle the release.
How to fix it
To make sure that a lock is always released correctly, you can follow one of these two methods:
- Use a
lock
statement with your
lock object.
- Use a
try-finally
statement and put the release of your lock object within the finally block.
Code examples
Noncompliant code example
class MyClass
{
private object obj = new object();
public void DoSomethingWithMonitor()
{
Monitor.Enter(obj); // Noncompliant: not all paths release the lock
if (IsInitialized())
{
// ...
Monitor.Exit(obj);
}
}
}
class MyClass
{
private ReaderWriterLockSlim lockObj = new ReaderWriterLockSlim();
public void DoSomethingWithReaderWriteLockSlim()
{
lockObj.EnterReadLock(); // Noncompliant: not all paths release the lock
if (IsInitialized())
{
// ...
lockObj.ExitReadLock();
}
}
}
Compliant solution
class MyClass
{
private object obj = new object();
public void DoSomethingWithMonitor()
{
lock(obj) // Compliant: the lock will be released at the end of the lock block
{
if (IsInitialized())
{
// ...
}
}
}
}
class MyClass
{
private ReaderWriterLockSlim lockObj = new ReaderWriterLockSlim();
public void DoSomethingWithReaderWriteLockSlim()
{
lockObj.EnterReadLock(); // Compliant: the lock will be released in the finally block
try
{
if (IsInitialized())
{
// ...
}
}
finally
{
lockObj.ExitReadLock();
}
}
}
Resources
© 2015 - 2024 Weber Informatics LLC | Privacy Policy