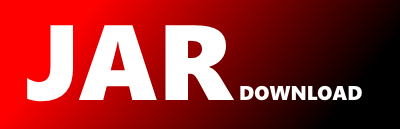
org.sonar.plugins.csharp.S2971.html Maven / Gradle / Ivy
Why is this an issue?
In the interests of readability, code that can be simplified should be simplified. To that end, there are several ways IEnumerable language integrated queries (LINQ) can be
simplified. This not only improves readabilty but can also lead to improved performance.
How to fix it
Simplify the LINQ expressions:
- Use OfType instead of Select with the as operator to type cast
elements and then null-checking in a query expression to choose elements based on type.
- Use
OfType
instead of using Where and the
is operator, followed
by a cast in a Select
- Use an expression in
Any
instead of Where(element ⇒ [expression]).Any()
.
- Use the Count or Length properties instead of the Count() method when it’s available (unless you use the
predicate parameter of the method for filtering).
- Don’t call ToArray() or ToList() in the middle of a query chain.
Using Entity Framework may require enforcing client evaluations. Such queries should use AsEnumerable() instead of ToArray()
or
ToList()
in the middle of a query chain.
Code examples
Noncompliant code example
public void Foo(IEnumerable<Vehicle> seq, List<int> list)
{
var result1 = seq.Select(x => x as Car).Any(x => x != null); // Noncompliant; use OfType
var result2 = seq.Select(x => x as Car).Any(x => x != null && x.HasOwner); // Noncompliant; use OfType before calling Any
var result3 = seq.Where(x => x is Car).Select(x => x as Car); // Noncompliant; use OfType
var result4 = seq.Where(x => x is Car).Select(x => (Car)x); // Noncompliant; use OfType
var result5 = seq.Where(x => x.HasOwner).Any(); // Noncompliant; use Any([predicate])
var num = list.Count(); // Noncompliant; use the Count property
var arr = seq.ToList().ToArray(); // Noncompliant; ToList is not needed
var count = seq.ToList().Count(x => x.HasOwner); // Noncompliant; ToList is not needed
}
Compliant solution
public void Foo(IEnumerable<Vehicle> seq, List<int> list)
{
var result1 = seq.OfType<Car>().Any();
var result2 = seq.OfType<Car>().Any(x => x.HasOwner);
var result3 = seq.OfType<Car>();
var result4 = seq.OfType<Car>();
var result5 = seq.Any(x => x.HasOwner);
var num = list.Count;
var arr = seq.ToArray();
var count = seq.Count(x => x.HasOwner);
}
Resources
Documentation
© 2015 - 2024 Weber Informatics LLC | Privacy Policy