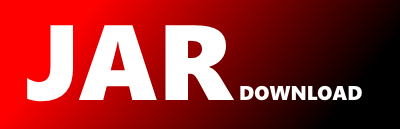
org.sonar.plugins.csharp.S2995.html Maven / Gradle / Ivy
Why is this an issue?
In C#, the Object.ReferenceEquals
method is
used to compare two reference type
variables. If you use this method to compare two value types, such as int
,
float
, or bool
you will not get the expected results because value type variables contain an instance of the type and not a
reference to it.
Due to value type variables containing directly an instance of the type, they can’t have the same reference, and using
Object.ReferenceEquals
to compare them will always return false
even if the compared variables have the same value.
How to fix it
When comparing value types, prefer using the Object.Equals
.
Note that in the case of structure types, it
is recommended to implement
value equality. If not, {rule:csharpsquid:S3898} might raise.
Code examples
Noncompliant code example
using System;
struct MyStruct
{
int valueA;
int valueB;
}
static class MyClass
{
public static void Method(MyStruct struct1, MyStruct struct2)
{
if (Object.ReferenceEquals(struct1, struct2)) // Noncompliant: this will be always false
{
// ...
}
}
}
Compliant solution
using System;
struct MyStruct : IEquatable<MyStruct>
{
int valueA;
int valueB;
public bool Equals(MyStruct other) => valueA == other.valueA && valueB == other.valueB;
public override bool Equals(object obj) => obj is MyStruct other && Equals(other);
public override int GetHashCode() => HashCode.Combine(valueA, valueB);
public static bool operator ==(MyStruct lhs, MyStruct rhs) => lhs.Equals(rhs);
public static bool operator !=(MyStruct lhs, MyStruct rhs) => !(lhs == rhs);
}
static class MyClass
{
public static void Method(MyStruct struct1, MyStruct struct2)
{
if (struct1.Equals(struct2)) // Compliant: value are compared
{
// ...
}
}
}
Resources
Documentation
- Microsoft Learn -
Object.ReferenceEquals(Object,
Object)
Method
- Microsoft Learn -
Object.Equals
Method
- Microsoft Learn - Value types (C#
reference)
- Microsoft Learn - Reference types (C#
reference)
- Microsoft Learn - Equality operators
- test if two objects are equal or not
- Microsoft Learn - How to define value equality for a class or struct (C# Programming Guide)
- Microsoft Learn - Structure types (C#
reference)
- {rule:csharpsquid:S3898} - Value types should implement "IEquatable<T>"
© 2015 - 2024 Weber Informatics LLC | Privacy Policy