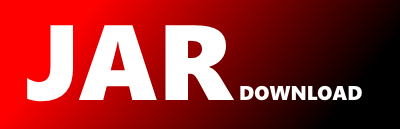
org.sonar.plugins.csharp.S2997.html Maven / Gradle / Ivy
Why is this an issue?
When you use a using
statement, the
goal is to ensure the correct disposal of an IDisposable
instance when the control leaves the using
statement block.
If you return that IDisposable
instance inside the block, using
will dispose it before the caller can use it, likely
causing exceptions at runtime. You should either remove using
statement or avoid returning the IDisposable
in the
using
statement block.
How to fix it
Code examples
Noncompliant code example
public FileStream WriteToFile(string path, string text)
{
using (var fs = File.Create(path)) // Noncompliant: 'fs' is disposed at the end of the using scope
{
var bytes = Encoding.UTF8.GetBytes(text);
fs.Write(bytes, 0, bytes.Length);
return fs;
}
}
Compliant solution
public FileStream WriteToFile(string path, string text)
{
var fs = File.Create(path);
var bytes = Encoding.UTF8.GetBytes(text);
fs.Write(bytes, 0, bytes.Length);
return fs; // Compliant: 'fs' is not disposed once the end of the scope is reached and the caller can use it
}
Resources
Documentation
- Microsoft Learn - using statement - ensure the
correct use of disposable objects
- Microsoft Learn - IDisposable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy