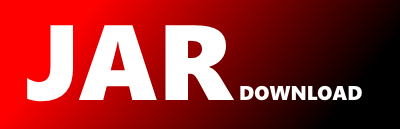
org.sonar.plugins.csharp.S3168.html Maven / Gradle / Ivy
Why is this an issue?
An async
method with a
void
return type does not follow the task asynchronous programming
(TAP) model since the return type should be Task
or Task<TResult>
Doing so prevents control over the asynchronous
execution, such as:
- waiting for the execution to complete
- catching any exception that might occur during execution
- testing execution behavior
Exceptions
- Methods with the
EventHandler
delegate signature. Using void
for EventHandler
is compliant with the TAP model.
public async void button1_Click(object sender, EventArgs e)
{
await DoSomethingAsync();
}
- Methods name matching
On[A-Z]\w*
pattern. Some frameworks may not use the same EventHandler
method signature
public async void OnClick(EventContext data)
{
await DoSomethingAsync();
}
How to fix it
Update the return type of the method from void
to Task
.
Code examples
Noncompliant code example
private async void ThrowExceptionAsync() // Noncompliant: async method return type is 'void'
{
throw new InvalidOperationException();
}
public void Method()
{
try
{
ThrowExceptionAsync();
}
catch (Exception)
{
// The exception is never caught here
throw;
}
}
Compliant solution
private async Task ThrowExceptionAsync() // Compliant: async method return type is 'Task'
{
throw new InvalidOperationException();
}
public async Task Method()
{
try
{
await ThrowExceptionAsync();
}
catch (Exception)
{
// The exception is caught here
throw;
}
}
Resources
Documentation
- Microsoft Learn -
async
(C#
Reference)
- Microsoft Learn - Asynchronous
programming
- Microsoft Learn - Task
asynchronous programming model
- Microsoft Learn -
Task
Class
- Microsoft Learn -
Task<TResult>
Class
- Microsoft Learn -
EventHandler
Delegate
© 2015 - 2024 Weber Informatics LLC | Privacy Policy