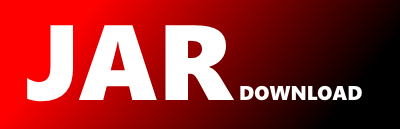
org.sonar.plugins.csharp.S1048.html Maven / Gradle / Ivy
Why is this an issue?
The finalizers are used to perform
any necessary final clean-up when
the garbage collector is collecting a class instance. The programmer has no control over when the finalizer is called; the garbage collector decides
when to call it.
When creating a finalizer, it should never throw an exception, as there is a high risk of having the application terminated leaving unmanaged
resources without a graceful cleanup.
The rule raises an issue on throw
statements used in a finalizer.
How to fix it
Code examples
Noncompliant code example
class MyClass
{
~MyClass()
{
throw new NotImplementedException(); // Noncompliant: finalizer throws an exception
}
}
Compliant solution
class MyClass
{
~MyClass()
{
// Compliant: finalizer does not throw an exception
}
}
Going the extra mile
In general object finalization can be a complex and error-prone operation and should not be implemented except within the dispose pattern.
When cleaning up unmanaged resources, it is
recommended to implement the dispose pattern or, to cover uncalled Dispose
method by the consumer, implement SafeHandle
.
Resources
Documentation
- Microsoft Learn - Fundamentals of garbage
collection
- Microsoft Learn - Cleaning up unmanaged resources
- Microsoft Learn - Implement a Dispose
method
- Microsoft Learn -
SafeHandle
Class
- Microsoft Learn -
IDisposable.Dispose
Method
- Microsoft Learn - Finalizers
(destructors)