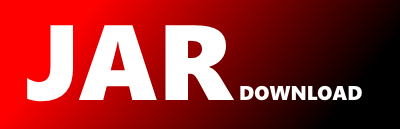
org.sonar.plugins.csharp.S3445.html Maven / Gradle / Ivy
Why is this an issue?
In C#, the throw
statement can be used in two different ways:
- by specifying an expression
- without specifying an expression
By specifying an expression
In the software development context, an expression is a value or anything that executes and ends up being a value. The expression shall be
implicitly convertible to System.Exception
, and the result of evaluating the expression is converted to System.Exception
before being thrown.
try
{
}
catch(Exception exception)
{
// code that uses the exception
throw exception; // The exception stack trace is cleared up to this point.
}
In this case, the stack trace, will be cleared, losing the list of method calls between the
original method that threw the exception and the current method.
Without specifying an expression
This syntax is supported only in a catch
block, in which case, that statement re-throws the exception currently being handled by that
catch
block, preserving the stack trace.
try
{
}
catch(Exception exception)
{
// code that uses the exception
throw; // The stack trace of the initial exception is preserved.
}
Exceptions
It is allowed using the thrown exception
as an argument and wrapping it in another exception
.
try
{
}
catch(Exception exception)
{
throw new Exception("Additional information", exception);
}
How to fix it
The recommended way to re-throw an exception is to use the throw statement without including an expression. This ensures that all call stack
information is preserved when the exception is propagated to the caller, making debugging easier.
Code examples
Noncompliant code example
try
{
}
catch(Exception exception)
{
throw exception;
}
Compliant solution
try
{
}
catch(Exception)
{
throw;
}
Resources
Documentation