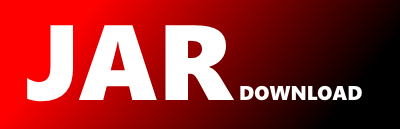
org.sonar.plugins.csharp.S3610.html Maven / Gradle / Ivy
Why is this an issue?
Calling GetType() on a nullable value type object returns
the underlying value type. Therefore, comparing the returned Type
object to typeof(Nullable<SomeType>)
will either throw an NullReferenceException or the result will always be
true
or false
and can be known at compile time.
How to fix it
Code examples
Noncompliant code example
void DoChecks<T>(Nullable<T> value) where T : struct
{
bool areEqual = value.GetType() == typeof(Nullable<int>); // Noncompliant: always false
bool areNotEqual = value.GetType() != typeof(Nullable<int>); // Noncompliant: always true
Nullable<int> nullable = null;
bool nullComparison = nullable.GetType() != typeof(Nullable<int>); // Noncompliant: throws NullReferenceException
}
Compliant solution
void DoChecks<T>(Nullable<T> value) where T : struct
{
bool areEqual = value.GetType() == typeof(int); // Compliant: can be true or false
bool areNotEqual = value.GetType() != typeof(int); // Compliant: can be true or false
Nullable<int> nullable = null;
bool nullComparison = nullable is not null && nullable.GetType() == typeof(int); // Compliant: does not throw NullReferenceException
}
Resources
Documentation
- Microsoft Learn - Object.GetType Method
- Microsoft Learn - Nullable
value types (C# reference)
- Microsoft Learn - Type Class
- Microsoft Learn - NullReferenceException Class
© 2015 - 2024 Weber Informatics LLC | Privacy Policy