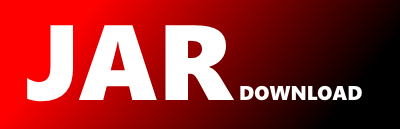
org.sonar.plugins.csharp.S4524.html Maven / Gradle / Ivy
Why is this an issue?
The switch
statement is a conditional statement that executes a sequence of instructions based on patterns matching the provided value.
switch (temperatureInCelsius)
{
case < 35.0:
Console.WriteLine("Hypothermia");
break;
case >= 36.5 and <= 37.5:
Console.WriteLine("Normal");
break;
case > 37.5 and <= 40.0:
Console.WriteLine("Fever or hyperthermia");
break;
case > 40.0:
Console.WriteLine("Hyperpyrexia");
break;
}
The switch
statement can optionally contain a default
clause, executed when none of the case
clauses are
executed (or in presence of a goto default;
).
switch (gradeLetter)
{
case "A+":
case "A":
case "A-":
Console.WriteLine("Excellent");
break;
case "B+":
case "B":
Console.WriteLine("Very Good");
break;
case "B-":
case "C+":
Console.WriteLine("Good");
break;
case "C":
Console.WriteLine("Pass");
break;
case "F":
Console.WriteLine("Fail");
break;
default:
Console.WriteLine("Invalid grade letter!");
break;
}
The default
clause can be defined for various reasons:
- to handle unexpected values, as shown in the example above
- or to show that all the cases were properly considered, making the function explicitely total (as opposed to partial)
While C# allows the default
clause to appear in any place within a switch
statement, and while its position doesn’t alter
its behavior, it is recommended to put the default
clause either at the beginning or at the end of the switch
statement.
That improves readability and helps the developer to quickly find the default behavior of a switch
statement.
This rule raises an issue if the default
clause is neither the first nor the last one of the switch
statement.
How to fix it
Code examples
Noncompliant code example
switch (param)
{
case 0:
DoSomething();
break;
default: // Noncompliant: default clause should be the first or last one
Error();
break;
case 1:
DoSomethingElse();
break;
}
Compliant solution
switch (param)
{
case 0:
DoSomething();
break;
case 1:
DoSomethingElse();
break;
default:
Error();
break;
}
Resources
Documentation