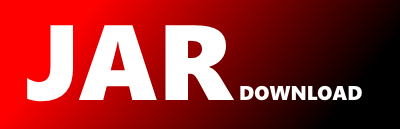
org.sonar.plugins.csharp.S6961.html Maven / Gradle / Ivy
In ASP.NET Core, controllers usually inherit either from ControllerBase
or Controller
. If a controller does not use any
View-specific functionality, it is recommended to inherit from ControllerBase
.
Why is this an issue?
The ControllerBase
class contains all the necessary functionality to handle API
requests and responses. The Controller
class inherits from ControllerBase
and adds support for Views, PartialViews and ViewComponents.
Inheriting from Controller
when not using any View-specific functionality exposes unnecessary methods and can lead to confusion about
the intention of the class.
Furthermore, inheriting from Controller
may come with a performance cost. Even though the controller might only deal with API
operations, the support for Views might introduce some overhead from the MVC framework during the request processing pipeline.
An issue is raised when:
- The class is marked with the
[ApiController]
attribute.
- The class inherits directly from
Controller
.
- No View-specific functionality is used in the class.
Exceptions
- If a class is marked with the
[NonController]
attribute.
- If a class does not have public accessibility.
How to fix it
Change the base type of the controller from Controller
to ControllerBase
.
Code examples
Noncompliant code example
[ApiController]
public class MyController : Controller // Noncompliant: Inherit from ControllerBase instead of Controller.
// ^^^^^^^^^^
{
// ..
}
Compliant solution
[ApiController]
public class MyController : ControllerBase
{
// ..
}
Resources
Documentation
- Wikipedia - Web API
- Microsoft Learn - ASP.NET Core
- Microsoft Learn - Controller Class
- Microsoft Learn - ControllerBase Class
- Microsoft Learn - ApiControllerAttribute
Class
- Microsoft Learn - NonControllerAttribute
Class
- Microsoft Learn - Views in ASP.NET Core MVC
- Microsoft Learn - Partial Views in ASP.NET Core MVC
- Microsoft Learn - View Components in ASP.NET Core MVC
- Microsoft Learn - ASP.NET Core Middleware