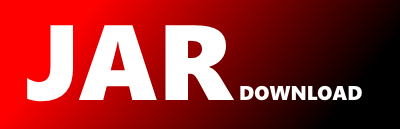
org.sonar.go.plugin.GoSensor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-go-plugin Show documentation
Show all versions of sonar-go-plugin Show documentation
SonarQube analyzer for Go language
/*
* SonarSource Go
* Copyright (C) 2018-2025 SonarSource SA
* mailto:info AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Sonar Source-Available License Version 1, as published by SonarSource SA.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the Sonar Source-Available License for more details.
*
* You should have received a copy of the Sonar Source-Available License
* along with this program; if not, see https://sonarsource.com/license/ssal/
*/
package org.sonar.go.plugin;
import java.util.function.Predicate;
import org.sonar.api.SonarRuntime;
import org.sonar.api.batch.rule.CheckFactory;
import org.sonar.api.batch.rule.Checks;
import org.sonar.api.batch.sensor.SensorContext;
import org.sonar.api.batch.sensor.SensorDescriptor;
import org.sonar.api.issue.NoSonarFilter;
import org.sonar.api.measures.FileLinesContextFactory;
import org.sonar.go.api.ASTConverter;
import org.sonar.go.api.NativeTree;
import org.sonar.go.api.Tree;
import org.sonar.go.api.VariableDeclarationTree;
import org.sonar.go.api.checks.GoCheck;
import org.sonar.go.checks.GoCheckList;
import org.sonar.go.converter.GoConverter;
public class GoSensor extends SlangSensor {
private final Checks checks;
private ASTConverter goConverter = null;
public GoSensor(SonarRuntime sonarRuntime, CheckFactory checkFactory, FileLinesContextFactory fileLinesContextFactory,
NoSonarFilter noSonarFilter, GoLanguage language, GoConverter goConverter) {
super(sonarRuntime, noSonarFilter, fileLinesContextFactory, language);
checks = initializeChecks(checkFactory);
this.goConverter = goConverter;
}
@Override
public void describe(SensorDescriptor descriptor) {
descriptor.onlyOnLanguage(GoLanguage.KEY)
.name("Code Quality and Security for Go");
}
@Override
protected ASTConverter astConverter(SensorContext sensorContext) {
return goConverter;
}
protected Checks initializeChecks(CheckFactory checkFactory) {
var goChecks = checkFactory.create(GoRulesDefinition.REPOSITORY_KEY);
goChecks.addAnnotatedChecks(GoCheckList.checks());
return goChecks;
}
@Override
protected Checks checks() {
return checks;
}
@Override
protected String repositoryKey() {
return GoRulesDefinition.REPOSITORY_KEY;
}
@Override
protected Predicate executableLineOfCodePredicate() {
return super.executableLineOfCodePredicate().and(t -> !(t instanceof VariableDeclarationTree)
&& !isGenericDeclaration(t));
}
private static boolean isGenericDeclaration(Tree tree) {
return tree instanceof NativeTree &&
((NativeTree) tree).nativeKind().toString().contains("GenDecl");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy