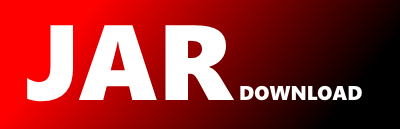
org.sonar.go.plugin.IssueSuppressionVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sonar-go-plugin Show documentation
Show all versions of sonar-go-plugin Show documentation
SonarQube analyzer for Go language
/*
* SonarSource Go
* Copyright (C) 2018-2025 SonarSource SA
* mailto:info AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Sonar Source-Available License Version 1, as published by SonarSource SA.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the Sonar Source-Available License for more details.
*
* You should have received a copy of the Sonar Source-Available License
* along with this program; if not, see https://sonarsource.com/license/ssal/
*/
package org.sonar.go.plugin;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.sonar.go.api.Annotation;
import org.sonar.go.api.ClassDeclarationTree;
import org.sonar.go.api.FunctionDeclarationTree;
import org.sonar.go.api.ParameterTree;
import org.sonar.go.api.TextRange;
import org.sonar.go.api.Tree;
import org.sonar.go.api.VariableDeclarationTree;
import org.sonar.go.visitors.TreeVisitor;
public class IssueSuppressionVisitor extends TreeVisitor {
private Map> filteredRules;
private static final List SUPPRESS_ANNOTATION_NAMES = Arrays.asList("Suppress", "SuppressWarnings");
private static final Pattern LITERAL_PATTERN = Pattern.compile("\"(.*?)\"");
public IssueSuppressionVisitor() {
register(FunctionDeclarationTree.class, (ctx, tree) -> checkSuppressAnnotations(tree));
register(ClassDeclarationTree.class, (ctx, tree) -> checkSuppressAnnotations(tree));
register(VariableDeclarationTree.class, (ctx, tree) -> checkSuppressAnnotations(tree));
register(ParameterTree.class, (ctx, tree) -> checkSuppressAnnotations(tree));
}
private void checkSuppressAnnotations(Tree tree) {
List annotations = tree.metaData().annotations();
TextRange textRange = tree.textRange();
annotations.forEach(annotation -> {
if (SUPPRESS_ANNOTATION_NAMES.contains(annotation.shortName())) {
getSuppressedKeys(annotation.argumentsText()).forEach(ruleKey -> filteredRules.computeIfAbsent(ruleKey, key -> new HashSet<>()).add(textRange));
}
});
}
private static Collection getSuppressedKeys(List argumentsText) {
List keys = new ArrayList<>();
for (String s : argumentsText) {
keys.addAll(getArgumentsValues(s));
}
return keys;
}
private static Collection getArgumentsValues(String argumentText) {
List values = new ArrayList<>();
Matcher m = LITERAL_PATTERN.matcher(argumentText);
while (m.find()) {
values.add(m.group(1));
}
return values;
}
@Override
protected void before(InputFileContext ctx, Tree root) {
filteredRules = new HashMap<>();
}
@Override
protected void after(InputFileContext ctx, Tree root) {
ctx.setFilteredRules(filteredRules);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy