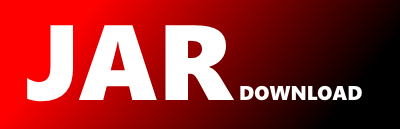
org.sonar.l10n.java.rules.squid.S2160.html Maven / Gradle / Ivy
Extend a class that overrides equals
and add fields without overriding equals
in the subclass, and you run the risk of non-equivalent instances of your subclass being seen as equal, because only the superclass fields will be considered in the equality test.
This rule looks for classes that do all of the following:
- extend classes that override
equals
.
- do not themselves override
equals
.
- add fields.
Noncompliant Code Example
public class Fruit {
private Season ripe;
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (this.class != obj.class) {
return false;
}
Fruit fobj = (Fruit) obj;
if (ripe.equals(fobj.getRipe()) {
return true;
}
return false;
}
}
public class Raspberry extends Fruit { // Noncompliant; instances will use Fruit's equals method
private Color ripeColor;
}
Compliant Solution
public class Fruit {
private Season ripe;
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (this.class != obj.class) {
return false;
}
Fruit fobj = (Fruit) obj;
if (ripe.equals(fobj.getRipe()) {
return true;
}
return false;
}
}
public class Raspberry extends Fruit {
private Color ripeColor;
public boolean equals(Object obj) {
if (! super.equals(obj)) {
return false;
}
Raspberry fobj = (Raspberry) obj;
if (ripeColor.equals(fobj.getRipeColor()) { // added fields are tested
return true;
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy