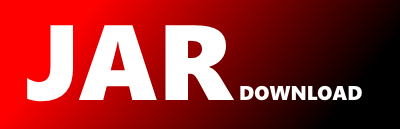
org.sonar.java.checks.CombineCatchCheck Maven / Gradle / Ivy
/*
* SonarQube Java
* Copyright (C) 2012-2024 SonarSource SA
* mailto:info AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Sonar Source-Available License Version 1, as published by SonarSource SA.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the Sonar Source-Available License for more details.
*
* You should have received a copy of the Sonar Source-Available License
* along with this program; if not, see https://sonarsource.com/license/ssal/
*/
package org.sonar.java.checks;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import org.sonar.check.Rule;
import org.sonar.plugins.java.api.JavaVersionAwareVisitor;
import org.sonar.java.checks.helpers.QuickFixHelper;
import org.sonar.java.model.JavaTree.UnionTypeTreeImpl;
import org.sonar.java.model.LineUtils;
import org.sonar.java.model.SyntacticEquivalence;
import org.sonar.java.model.expression.MemberSelectExpressionTreeImpl;
import org.sonar.java.reporting.JavaQuickFix;
import org.sonar.java.reporting.JavaTextEdit;
import org.sonar.plugins.java.api.IssuableSubscriptionVisitor;
import org.sonar.plugins.java.api.JavaFileScannerContext;
import org.sonar.plugins.java.api.JavaVersion;
import org.sonar.plugins.java.api.tree.CatchTree;
import org.sonar.plugins.java.api.tree.Tree;
import org.sonar.plugins.java.api.tree.TryStatementTree;
import org.sonar.plugins.java.api.tree.TypeTree;
@Rule(key = "S2147")
public class CombineCatchCheck extends IssuableSubscriptionVisitor implements JavaVersionAwareVisitor {
@Override
public List nodesToVisit() {
return Collections.singletonList(Tree.Kind.TRY_STATEMENT);
}
@Override
public void visitNode(Tree tree) {
ArrayDeque catches = new ArrayDeque<>();
for (CatchTree catchTree : ((TryStatementTree) tree).catches()) {
for (CatchTree catchTreeToBeCompared : catches) {
if (SyntacticEquivalence.areSemanticallyEquivalent(catchTree.block().body(), catchTreeToBeCompared.block().body())) {
reportIssueWithQuickFix(catchTree, catchTreeToBeCompared);
break;
}
}
catches.push(catchTree);
}
}
private void reportIssueWithQuickFix(CatchTree catchTree, CatchTree catchTreeToBeCompared) {
String quickFixMessage = "Combine this catch with the one at line " + LineUtils.startLine(catchTreeToBeCompared.catchKeyword());
String issueMessage = quickFixMessage + ", which has the same body." + context.getJavaVersion().java7CompatibilityMessage();
List flow = Collections.singletonList(new JavaFileScannerContext.Location("Combine with this catch", catchTreeToBeCompared));
QuickFixHelper.newIssue(context)
.forRule(this)
.onTree(catchTree.parameter())
.withMessage(issueMessage)
.withSecondaries(flow)
.withQuickFix( () -> computeQuickFix(catchTree, catchTreeToBeCompared, quickFixMessage) )
.report();
}
@Override
public boolean isCompatibleWithJavaVersion(JavaVersion version) {
return version.isJava7Compatible();
}
private JavaQuickFix computeQuickFix(CatchTree catchTree, CatchTree catchTreeToBeCompared, String qfMessage) {
List upperCatchTypes = getExceptionTypesCaught(catchTreeToBeCompared);
List lowerCatchTypes = getExceptionTypesCaught(catchTree);
List mergedTypes = mergeCatchTypes(upperCatchTypes, lowerCatchTypes);
var builder = JavaQuickFix.newQuickFix(qfMessage);
builder.addTextEdit(JavaTextEdit.removeTree(catchTree));
String replacement = computeReplacementString(mergedTypes, catchTreeToBeCompared);
builder.addTextEdit(
JavaTextEdit.replaceBetweenTree(
catchTreeToBeCompared.openParenToken(), false,
catchTreeToBeCompared.closeParenToken(), false,
replacement)
);
return builder.build();
}
private String computeReplacementString(Collection types, CatchTree toReplaceCatch) {
StringBuilder sb = new StringBuilder();
types.forEach(type -> sb.append(formatType(type) + " | "));
sb.delete(sb.lastIndexOf("| "), sb.length());
sb.append(toReplaceCatch.parameter().simpleName().name());
return sb.toString();
}
private static List mergeCatchTypes(List upperCatchTypes, List lowerCatchTypes) {
List result = new ArrayList<>();
for (TypeTree upperType : upperCatchTypes) {
if (isNotMaskedBySuperType(upperType, lowerCatchTypes)) {
result.add(upperType);
}
}
result.addAll(lowerCatchTypes);
return result;
}
private static boolean isNotMaskedBySuperType(TypeTree type, List types) {
for (TypeTree other : types) {
if (type.symbolType().isSubtypeOf(other.symbolType())) {
return false;
}
}
return true;
}
private String formatType(TypeTree type) {
if(type instanceof MemberSelectExpressionTreeImpl mtype) {
return QuickFixHelper.contentForTree(mtype, context);
}
return type.toString();
}
private static List getExceptionTypesCaught(CatchTree catchTree){
TypeTree catchType = catchTree.parameter().type();
if(catchType instanceof UnionTypeTreeImpl unionTypes) {
unionTypes.symbolType();
return unionTypes.typeAlternatives();
}else {
return List.of(catchType);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy