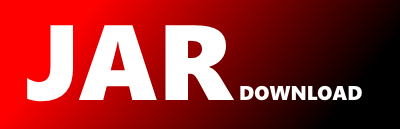
org.sonar.java.checks.RandomFloatToIntCheck Maven / Gradle / Ivy
/*
* SonarQube Java
* Copyright (C) 2012-2024 SonarSource SA
* mailto:info AT sonarsource DOT com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the Sonar Source-Available License Version 1, as published by SonarSource SA.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the Sonar Source-Available License for more details.
*
* You should have received a copy of the Sonar Source-Available License
* along with this program; if not, see https://sonarsource.com/license/ssal/
*/
package org.sonar.java.checks;
import java.util.Collections;
import java.util.List;
import org.sonar.check.Rule;
import org.sonar.plugins.java.api.IssuableSubscriptionVisitor;
import org.sonar.plugins.java.api.semantic.MethodMatchers;
import org.sonar.plugins.java.api.semantic.Type;
import org.sonar.plugins.java.api.tree.BaseTreeVisitor;
import org.sonar.plugins.java.api.tree.MethodInvocationTree;
import org.sonar.plugins.java.api.tree.NewClassTree;
import org.sonar.plugins.java.api.tree.Tree;
import org.sonar.plugins.java.api.tree.TypeCastTree;
@Rule(key = "S2140")
public class RandomFloatToIntCheck extends IssuableSubscriptionVisitor {
private static final String NEXT_FLOAT = "nextFloat";
private static final String NEXT_DOUBLE = "nextDouble";
private static final MethodMatchers MATH_RANDOM_METHOD_MATCHER = MethodMatchers.create()
.ofTypes("java.lang.Math").names("random").addWithoutParametersMatcher().build();
private static final MethodMatchers METHOD_MATCHERS = MethodMatchers.or(
MethodMatchers.create()
.ofTypes("java.util.concurrent.ThreadLocalRandom")
.names(NEXT_DOUBLE)
.withAnyParameters()
.build(),
MethodMatchers.create()
.ofTypes(
"java.util.Random",
"org.apache.commons.lang.math.JVMRandom",
"org.apache.commons.lang.math.RandomUtils",
"org.apache.commons.lang3.RandomUtils")
.names(NEXT_DOUBLE, NEXT_FLOAT)
.addWithoutParametersMatcher()
.build());
@Override
public List nodesToVisit() {
return Collections.singletonList(Tree.Kind.TYPE_CAST);
}
@Override
public void visitNode(Tree tree) {
TypeCastTree castTree = (TypeCastTree) tree;
Type castToType = castTree.type().symbolType();
if (castToType.is("int")) {
castTree.expression().accept(new RandomDoubleVisitor("nextInt()"));
} else if (castToType.is("long")) {
castTree.expression().accept(new RandomDoubleVisitor("nextLong()"));
}
}
private class RandomDoubleVisitor extends BaseTreeVisitor {
private final String methodToCall;
public RandomDoubleVisitor(String methodToCall){
this.methodToCall = methodToCall;
}
@Override
public void visitMethodInvocation(MethodInvocationTree tree) {
if (MATH_RANDOM_METHOD_MATCHER.matches(tree)) {
reportIssue(tree.methodSelect(), "Use \"java.util.Random." + methodToCall + "\" instead.");
} else if (METHOD_MATCHERS.matches(tree)) {
reportIssue(tree.methodSelect(), "Use \"" + methodToCall + "\" instead.");
}
super.visitMethodInvocation(tree);
}
@Override
public void visitNewClass(NewClassTree tree) {
scan(tree.enclosingExpression());
scan(tree.identifier());
scan(tree.typeArguments());
scan(tree.arguments());
//do not scan body of anonymous classes.
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy