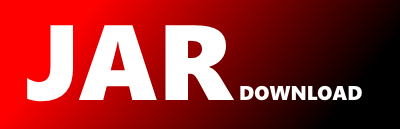
org.sonar.l10n.java.rules.java.S1948.html Maven / Gradle / Ivy
This rule raises an issue on a non-transient and non-serializable field within a serializable class, if said class does not have
writeObject
and readObject
methods defined.
Why is this an issue?
By contract, non-static fields in a Serializable
class must themselves be either Serializable
or transient
.
Even if the class is never explicitly serialized or deserialized, it is not safe to assume that this cannot happen. For instance, under load, most
J2EE application frameworks flush objects to disk.
An object that implements Serializable
but contains non-transient, non-serializable data members (and thus violates the contract)
could cause application crashes and open the door to attackers. In general, a Serializable
class is expected to fulfil its contract and
not exhibit unexpected behaviour when an instance is serialized.
This rule raises an issue on:
- Non-
Serializable
fields.
- When a field is assigned a non-
Serializable
type within the class.
- Collection fields when they are not
private
. Values that are not serializable could be added to these collections externally. Due
to type erasure, it cannot be guaranteed that the collection will only contain serializable objects at runtime despite being declared as a
collection of serializable types.
How to fix it
Consider the following scenario.
public class Address {
...
}
public class Person implements Serializable {
private static final long serialVersionUID = 1905122041950251207L;
private String name;
private Address address; // Noncompliant, Address is not serializable
}
How to fix this issue depends on the application’s needs. If the field’s value should be preserved during serialization and deserialization, you
may want to make the field’s value serializable.
public class Address implements Serializable {
private static final long serialVersionUID = 2405172041950251807L;
...
}
public class Person implements Serializable {
private static final long serialVersionUID = 1905122041950251207L;
private String name;
private Address address; // Compliant, Address is serializable
}
If the field’s value does not need to be preserved during serialization and deserialization, mark it as transient
. The field will be
ignored when the object is serialized. After deserialization, the field will be set to the default value corresponding to its type (e.g.,
null
for object references).
public class Address {
...
}
public class Person implements Serializable {
private static final long serialVersionUID = 1905122041950251207L;
private String name;
private transient Address address; // Compliant, the field is transient
}
The alternative to making all members serializable or transient
is to implement special methods which take on the responsibility of
properly serializing and de-serializing the object writeObject
and readObject
. These methods can be used to properly
(de-)serialize an object, even though it contains fields that are not transient or serializable. Hence, this rule does not raise issues on fields of
classes which implement these methods.
public class Address {
...
}
public class Person implements Serializable {
private static final long serialVersionUID = 1905122041950251207L;
private String name;
private Address address; // Compliant, writeObject and readObject handle this field
private void writeObject(java.io.ObjectOutputStream out) throws IOException {
// Appropriate serialization logic here
}
private void readObject(java.io.ObjectInputStream in) throws IOException, ClassNotFoundException {
// Appropriate deserialization logic here
}
}
Finally, static fields are out of scope for serialization, so making a field static prevents issues from being raised.
public class Person implements Serializable {
private static final long serialVersionUID = 1905122041950251207L;
private String name;
private static Logger log = getLogger(); // Compliant, static fields are not serialized
}
Resources