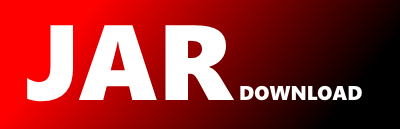
org.sonar.l10n.java.rules.java.S6073.html Maven / Gradle / Ivy
Why is this an issue?
Mockito provides argument matchers and argument captors for flexibly stubbing or verifying method calls.
Mockito.verify()
, Mockito.when()
, Stubber.when()
and BDDMockito.given()
each have overloads
with and without argument matchers.
However, if argument matchers or captors are used only on some of the parameters, all the parameters need to have matchers as well, otherwise an
InvalidUseOfMatchersException
will be thrown.
This rule consequently raises an issue every time matchers are not used on all the parameters of a stubbed/verified method.
How to fix it
Code examples
Noncompliant code example
@Test
public void myTest() {
// Setting up mock responses
given(foo.bar(anyInt(), i1, i2)).willReturn(null); // Noncompliant, no matchers for "i1" and "i2"
when(foo.baz(eq(val1), val2)).thenReturn("hi"); // Noncompliant, no matcher for "val2"
// Simulating exceptions
doThrow(new RuntimeException()).when(foo).quux(intThat(x -> x >= 42), -1); // Noncompliant, no matcher for "-1"
// Verifying method invocations
verify(foo).bar(i1, anyInt(), i2); // Noncompliant, no matchers for "i1" and "i2"
// Capturing arguments for verification
ArgumentCaptor<Integer> captor = ArgumentCaptor.forClass(Integer.class);
verify(foo).bar(captor.capture(), i1, any()); // Noncompliant, no matchers for "i1"
}
Compliant solution
@Test
public void myTest() {
// Setting up mock responses
given(foo.bar(anyInt(), eq(i1), eq(i2))).willReturn(null); // Compliant, all arguments have matchers
when(foo.baz(val1, val2)).thenReturn("hi"); // Compliant, no argument has matchers
// Simulating exceptions
doThrow(new RuntimeException()).when(foo).quux(intThat(x -> x >= 42), eq(-1)); // Compliant, all arguments have matchers
// Verifying method invocations
verify(foo).bar(eq(i1), anyInt(), eq(i2)); // Compliant, all arguments have matchers
// Capturing arguments for verification
ArgumentCaptor<Integer> captor = ArgumentCaptor.forClass(Integer.class);
verify(foo).bar(captor.capture(), any(), any()); // Compliant, all arguments have matchers
}
Resources
© 2015 - 2025 Weber Informatics LLC | Privacy Policy