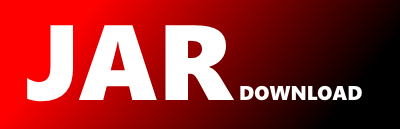
org.sonar.l10n.javascript.rules.javascript.S3776.html Maven / Gradle / Ivy
This rule raises an issue when the code cognitive complexity of a function is above a certain threshold.
Why is this an issue?
Cognitive Complexity is a measure of how hard it is to understand the control flow of a unit of code. Code with high cognitive complexity is hard
to read, understand, test, and modify.
As a rule of thumb, high cognitive complexity is a sign that the code should be refactored into smaller, easier-to-manage pieces.
Which syntax in code does impact cognitive complexity score?
Here are the core concepts:
- Cognitive complexity is incremented each time the code breaks the normal linear reading flow.
This concerns, for example,
loop structures, conditionals, catches, switches, jumps to labels, and conditions mixing multiple operators.
- Each nesting level increases complexity.
During code reading, the deeper you go through nested layers, the harder it
becomes to keep the context in mind.
- Method calls are free
A well-picked method name is a summary of multiple lines of code. A reader can first explore a
high-level view of what the code is performing then go deeper and deeper by looking at called functions content.
Note: This does not
apply to recursive calls, those will increment cognitive score.
The method of computation is fully detailed in the pdf linked in the resources.
What is the potential impact?
Developers spend more time reading and understanding code than writing it. High cognitive complexity slows down changes and increases the cost of
maintenance.
Exceptions
Cognitive complexity calculations exclude logical expressions using the ||
and ??
operators when applied within the
context of default value code patterns.
function greet(name) {
name = name || 'Guest';
console.log('Hello, ' + name + '!');
}
How to fix it
Reducing cognitive complexity can be challenging.
Here are a few suggestions:
- Extract complex conditions in a new function.
Mixed operators in condition will increase complexity. Extracting the
condition in a new function with an appropriate name will reduce cognitive load.
- Break down large functions.
Large functions can be hard to understand and maintain. If a function is doing too many
things, consider breaking it down into smaller, more manageable functions. Each function should have a single responsibility.
- Avoid deep nesting by returning early.
To avoid the nesting of conditions, process exceptional cases first and return
early.
- Use null-safe operations (if available in the language).
When available the .?
or ??
operator
replaces multiple tests and simplifies the flow.
Note that the calculation of cognitive complexity deviates from the documented process when functions are nested. Given the functional nature of
JavaScript, nesting functions is a prevalent practice, especially within frameworks like React.js. Consequently, the cognitive complexity of functions
remains independent of each other.
Code examples
Extraction of a complex condition in a new function.
Noncompliant code example
The code is using a complex condition and has a cognitive cost of 3.
function calculateFinalPrice(user, cart) {
let total = calculateTotal(cart);
if (user.hasMembership // +1 (if)
&& user.orders > 10 // +1 (more than one condition)
&& user.accountActive
&& !user.hasDiscount
|| user.orders === 1) { // +1 (change of operator in condition)
total = applyDiscount(user, total);
}
return total;
}
Compliant solution
Even if the cognitive complexity of the whole program did not change, it is easier for a reader to understand the code of the
calculateFinalPrice
function, which now only has a cognitive cost of 1.
function calculateFinalPrice(user, cart) {
let total = calculateTotal(cart);
if (isEligibleForDiscount(user)) { // +1 (if)
total = applyDiscount(user, total);
}
return total;
}
function isEligibleForDiscount(user) {
return user.hasMembership
&& user.orders > 10 // +1 (more than one condition)
&& user.accountActive
&& !user.hasDiscount
|| user.orders === 1 // +1 (change of operator in condition)
}
Break down large functions.
Noncompliant code example
For example, consider a function that calculates the total price of a shopping cart, including sales tax and shipping.
Note: The code
is simplified here, to illustrate the purpose. Please imagine there is more happening in the for
loops.
function calculateTotal(cart) {
let total = 0;
for (let i = 0; i < cart.length; i++) { // +1 (for)
total += cart[i].price;
}
// calculateSalesTax
for (let i = 0; i < cart.length; i++) { // +1 (for)
total += 0.2 * cart[i].price;
}
//calculateShipping
total += 5 * cart.length;
return total;
}
This function could be refactored into smaller functions: The complexity is spread over multiple functions and the complex
calculateTotal
has now a complexity score of zero.
Compliant solution
function calculateTotal(cart) {
let total = calculateSubtotal(cart);
total += calculateSalesTax(cart);
total += calculateShipping(cart);
return total;
}
function calculateSubtotal(cart) {
let subTotal = 0;
for (const item of cart) { // +1 (for)
subTotal += item.price;
}
return subTotal;
}
function calculateSalesTax(cart) {
let salesTax = 0;
for (const item of cart) { // +1 (for)
salesTax += 0.2 * item.price;
}
return salesTax;
}
function calculateShipping(cart) {
return 5 * cart.length;
}
Avoid deep nesting by returning early.
Noncompliant code example
The below code has a cognitive complexity of 6.
function calculateDiscount(price, user) {
if (isEligibleForDiscount(user)) { // +1 ( if )
if (user?.hasMembership) { // +2 ( nested if )
return price * 0.9;
} else if (user?.orders === 1 ) { // +1 ( else )
return price * 0.95;
} else { // +1 ( else )
return price;
}
} else { // +1 ( else )
return price;
}
}
Compliant solution
Checking for the edge case first flattens the if
statements and reduces the cognitive complexity to 3.
function calculateDiscount(price, user) {
if (!isEligibleForDiscount(user)) { // +1 ( if )
return price;
}
if (user?.hasMembership) { // +1 ( if )
return price * 0.9;
}
if (user?.orders === 1) { // +1 ( if )
return price * 0.95;
}
return price;
}
Use the optional chaining operator to access data.
In the below code, the cognitive complexity is increased due to the multiple checks required to access the manufacturer’s name. This can be
simplified using the optional chaining operator.
Noncompliant code example
let manufacturerName = null;
if (product && product.details && product.details.manufacturer) { // +1 (if) +1 (multiple condition)
manufacturerName = product.details.manufacturer.name;
}
if (manufacturerName) { // +1 (if)
console.log(manufacturerName);
} else {
console.log('Manufacturer name not found');
}
Compliant solution
The optional chaining operator will return undefined
if any reference in the chain is undefined
or null
,
avoiding multiple checks:
let manufacturerName = product?.details?.manufacturer?.name;
if (manufacturerName) { // +1 (if)
console.log(manufacturerName);
} else {
console.log('Manufacturer name not found');
}
Pitfalls
As this code is complex, ensure that you have unit tests that cover the code before refactoring.
Resources
Documentation
- Sonar - Cognitive Complexity
Articles & blog posts
- Sonar Blog - 5 Clean Code Tips for Reducing
Cognitive Complexity