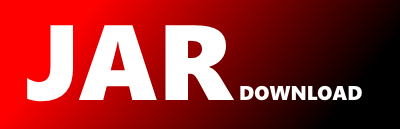
org.sonar.l10n.py.rules.python.S5644.html Maven / Gradle / Ivy
This rule raises an issue when an item operation is performed on an object which doesn’t have the corresponding method.
Why is this an issue?
Getting, setting and deleting items using square brackets requires the accessed object to have special methods:
- Getting items such as
my_variable[key]
requires my_variable
to have the __getitem__
method, or the __class_getitem__
method if
my_variable
is a class.
- Setting items such as
my_variable[key] = 42
requires my_variable
to have the __setitem__
method.
- Deleting items such as
del my_variable[key]
requires my_variable
to have the __delitem__
method.
Performing these operations on an object that doesn’t have the corresponding method will result in a TypeError
.
To fix this issue, make sure that the class for which you are trying to perform item operations implements the required methods.
Code examples
Noncompliant code example
del (1, 2)[0] # Noncompliant: tuples are immutable
(1, 2)[0] = 42 # Noncompliant
(1, 2)[0]
class A:
def __init__(self, values):
self._values = values
a = A([0,1,2])
a[0] # Noncompliant
del a[0] # Noncompliant
a[0] = 42 # Noncompliant
class B:
pass
B[0] # Noncompliant
Compliant solution
del [1, 2][0] # Lists are mutable
[1, 2][0] = 42
[1, 2][0]
class A:
def __init__(self, values):
self._values = values
def __getitem__(self, key):
return self._values[key]
def __setitem__(self, key, value):
self._values[key] = value
def __delitem__(self, key):
del self._values[key]
a = A([0,1,2])
a[0]
del a[0]
a[0] = 42
class B:
def __class_getitem__(cls, key):
return [0, 1, 2, 3][key]
B[0]
Resources
Documentation
© 2015 - 2024 Weber Informatics LLC | Privacy Policy