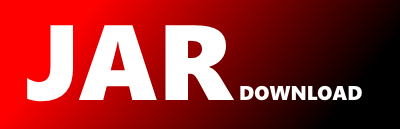
org.sonar.l10n.py.rules.python.S5708.html Maven / Gradle / Ivy
This rule raises an issue when the expression used in an except
statement is neither a class deriving from BaseException
nor a tuple of such classes.
Why is this an issue?
In Python 3’s except
statement, attempting to catch an object that does not derive from BaseException
will raise a
TypeError
.
In order to catch multiple exceptions in an except
statement, a tuple
of exception classes should be provided. Trying to
catch multiple exceptions with a list
or a set
will raise a TypeError
.
If you are about to create a custom exception class, note that custom exceptions should inherit from Exception
, rather than
BaseException
.
BaseException
is the base class for all built-in exceptions in Python, including system-exiting exceptions like
SystemExit
or KeyboardInterrupt
, which are typically not meant to be caught. On the other hand, Exception
is
intended for exceptions that are expected to be caught, which is generally the case for user-defined exceptions. See PEP 352 for more information.
To fix this issue, make sure the expression used in an except
statement is an exception which derives from
BaseException
/Exception
or a tuple of such exceptions.
Code examples
Noncompliant code example
class CustomException(object):
"""An Invalid exception class."""
pass
try:
...
except CustomException: # Noncompliant: this custom exception does not derive from BaseException or Exception.
print("exception")
try:
...
except [TypeError, ValueError]: # Noncompliant: list of exceptions, only tuples are valid.
print("exception")
Compliant solution
class CustomException(Exception):
pass
try:
...
except CustomException:
print("exception")
try:
...
except (TypeError, ValueError):
print("exception")
Note: In Python 2 it is possible to raise and catch old-style classes, which do not inherit from
BaseException
.
Resources
Documentation
- Python Documentation - Errors and Exceptions
- Python Documentation - The
try
statement
- Python Documentation - Exception hierarchy
Standards
- PEP 352 - Required Superclass for Exceptions