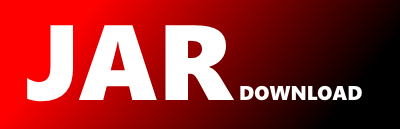
org.sonar.l10n.py.rules.python.S6327.html Maven / Gradle / Ivy
Amazon Simple Notification Service (SNS) is a managed messaging service for application-to-application (A2A) and application-to-person (A2P)
communication. SNS topics allows publisher systems to fanout messages to a large number of subscriber systems. Amazon SNS allows to encrypt messages
when they are received. In the case that adversaries gain physical access to the storage medium or otherwise leak a message they are not able to
access the data.
Ask Yourself Whether
- The topic contains sensitive data that could cause harm when leaked.
- There are compliance requirements for the service to store data encrypted.
There is a risk if you answered yes to any of those questions.
Recommended Secure Coding Practices
It is recommended to encrypt SNS topics that contain sensitive information.
To do so, create a master key and assign the SNS topic to it. Note that this system does not encrypt the following:
- Topic metadata (topic name and attributes)
- Message metadata (subject, message ID, timestamp, and attributes)
- Data protection policy
- Per-topic metrics
Then, make sure that any publishers have the kms:GenerateDataKey*
and kms:Decrypt
permissions for the AWS KMS key.
See AWS SNS Key Management
Documentation for more information.
Sensitive Code Example
from aws_cdk import (
aws_sns as sns
)
class TopicStack(Stack):
def __init__(self, scope: Construct, construct_id: str, **kwargs) -> None:
super().__init__(scope, construct_id, **kwargs)
sns.Topic( # Sensitive, unencrypted by default
self,
"example"
)
from aws_cdk import (
aws_sns as sns
)
class TopicStack(Stack):
def __init__(self, scope: Construct, construct_id: str, **kwargs) -> None:
super().__init__(scope, construct_id, **kwargs)
sns.CfnTopic( # Sensitive, unencrypted by default
self,
"example"
)
Compliant Solution
from aws_cdk import (
aws_sns as sns
)
class TopicStack(Stack):
def __init__(self, scope: Construct, construct_id: str, **kwargs) -> None:
super().__init__(scope, construct_id, **kwargs)
my_key = kms.Key(self, "key")
sns.Topic(
self,
"example",
master_key=my_key
)
from aws_cdk import (
aws_sns as sns
)
class TopicStack(Stack):
def __init__(self, scope: Construct, construct_id: str, **kwargs) -> None:
super().__init__(scope, construct_id, **kwargs)
my_key = kms.Key(self, "key")
sns.CfnTopic(
self,
"example",
kms_master_key_id=my_key.key_id
)
See
- AWS Documentation - Encryption at rest
- Encrypting messages published to
Amazon SNS with AWS KMS
- CWE - CWE-311 - Missing Encryption of Sensitive Data
- STIG Viewer - Application Security and
Development: V-222588 - The application must implement approved cryptographic mechanisms to prevent unauthorized modification of information at
rest.