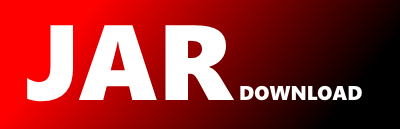
org.sonar.l10n.py.rules.python.S6663.html Maven / Gradle / Ivy
This rule raises an issue when an object used as a sequence index does not define an __index__
method.
Why is this an issue?
Objects can be used as sequence indexes to access a specific element from the sequence, through the following syntax:
my_list = [1, 2, 3, 4]
x = 1
print(my_list[x]) # This will print 2
Whenever an object is used as a sequence index, the Python interpreter calls its __index__
method to compute the index that needs to
be accessed from the sequence.
Any object can be used as sequence index, as long as it defines an __index__
method that returns an int
. Most commonly,
sequence indexes are simply integers.
Similarly, sequences can be sliced through the following syntax:
my_list = [1, 2, 3, 4]
x = 1
print(my_list[1:3]) # This will print [2, 3]
If an invalid object is used as a sequence index, a TypeError
will be raised.
How to fix it
Make sure to use an object that defines an __index__
method as sequence indexes.
Code examples
Noncompliant code example
def foo():
my_list = ["spam", "eggs"]
x = my_list["spam"] # Noncompliant: a str is not a valid index
Compliant solution
def foo():
my_list = ["spam", "eggs"]
x = my_list[0]
Resources
Documentation
Python Documentation - https://docs.python.org/3/library/operator.html#operator.index[index
method]